mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-22 03:30:35 +00:00
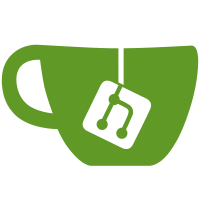
Signed-off-by: Thabokani <149070269+Thabokani@users.noreply.github.com> Co-authored-by: Nishant Das <nishdas93@gmail.com>
37 lines
847 B
Go
37 lines
847 B
Go
package keygen
|
|
|
|
import (
|
|
"encoding/json"
|
|
"fmt"
|
|
"io"
|
|
|
|
log "github.com/sirupsen/logrus"
|
|
)
|
|
|
|
// UnencryptedKeysContainer defines the structure of the unencrypted key JSON file.
|
|
type UnencryptedKeysContainer struct {
|
|
Keys []*UnencryptedKeys `json:"keys"`
|
|
}
|
|
|
|
// UnencryptedKeys is the inner struct of the JSON file.
|
|
type UnencryptedKeys struct {
|
|
ValidatorKey []byte `json:"validator_key"`
|
|
WithdrawalKey []byte `json:"withdrawal_key"`
|
|
}
|
|
|
|
// SaveUnencryptedKeysToFile JSON encodes the container and writes to the writer.
|
|
func SaveUnencryptedKeysToFile(w io.Writer, ctnr *UnencryptedKeysContainer) error {
|
|
enc, err := json.Marshal(ctnr)
|
|
if err != nil {
|
|
log.Fatal(err)
|
|
}
|
|
n, err := w.Write(enc)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if n != len(enc) {
|
|
return fmt.Errorf("failed to write %d bytes to file, wrote %d", len(enc), n)
|
|
}
|
|
return nil
|
|
}
|