mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
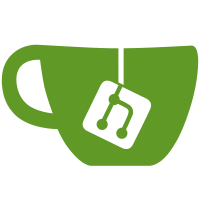
* Add `GetWithoutCopy` * Add `StateByRootInitialSync` * Use `StateByRootInitialSync` for initial syncing using new-state-mgmt * Merge branch 'master' into new-state-init-sync-mem * Skip if split slot is 0 * Merge branch 'new-state-init-sync-mem' of github.com:prysmaticlabs/prysm into new-state-init-sync-mem * Make sure we invalidate cache * Add test part 1 * Add tests part 2 * Update beacon-chain/cache/hot_state_cache.go Co-authored-by: Victor Farazdagi <simple.square@gmail.com> * Comment * Merge branch 'new-state-init-sync-mem' of github.com:prysmaticlabs/prysm into new-state-init-sync-mem * Merge branch 'master' of github.com:prysmaticlabs/prysm into new-state-init-sync-mem * Dont need to run fork choice and save head during intial sync * Invalidate cache at onBlockInitialSyncStateTransition * Merge branch 'new-state-init-sync-mem' of github.com:prysmaticlabs/prysm into new-state-init-sync-mem * Revert saveHeadNoDB changes * Add back DeleteHotStateInCache * Removed extra deletion * Merge branch 'master' of github.com:prysmaticlabs/prysm into new-state-init-sync-mem * Proper set splitslot for tests * Merge branch 'master' into new-state-init-sync-mem * Merge branch 'master' of github.com:prysmaticlabs/prysm into new-state-init-sync-mem * Merge branch 'new-state-init-sync-mem' of github.com:prysmaticlabs/prysm into new-state-init-sync-mem
47 lines
1017 B
Go
47 lines
1017 B
Go
package cache_test
|
|
|
|
import (
|
|
"reflect"
|
|
"testing"
|
|
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/cache"
|
|
stateTrie "github.com/prysmaticlabs/prysm/beacon-chain/state"
|
|
pb "github.com/prysmaticlabs/prysm/proto/beacon/p2p/v1"
|
|
)
|
|
|
|
func TestHotStateCache_RoundTrip(t *testing.T) {
|
|
c := cache.NewHotStateCache()
|
|
root := [32]byte{'A'}
|
|
state := c.Get(root)
|
|
if state != nil {
|
|
t.Errorf("Empty cache returned an object: %v", state)
|
|
}
|
|
if c.Has(root) {
|
|
t.Error("Empty cache has an object")
|
|
}
|
|
|
|
state, err := stateTrie.InitializeFromProto(&pb.BeaconState{
|
|
Slot: 10,
|
|
})
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
c.Put(root, state)
|
|
|
|
if !c.Has(root) {
|
|
t.Error("Empty cache does not have an object")
|
|
}
|
|
res := c.Get(root)
|
|
if state == nil {
|
|
t.Errorf("Empty cache returned an object: %v", state)
|
|
}
|
|
if !reflect.DeepEqual(state.CloneInnerState(), res.CloneInnerState()) {
|
|
t.Error("Expected equal protos to return from cache")
|
|
}
|
|
|
|
c.Delete(root)
|
|
if c.Has(root) {
|
|
t.Error("Cache not suppose to have the object")
|
|
}
|
|
}
|