mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
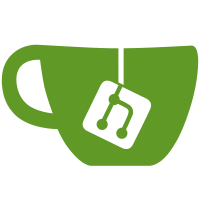
* Added analyzer for detecting recursive/nested mutex read locks. * Added type assertion checks and removed unused 'iTypes' directory * Clean up * Bazel file fixes * Cleaned up code and added comments. Co-authored-by: Raul Jordan <raul@prysmaticlabs.com>
53 lines
792 B
Go
53 lines
792 B
Go
// THE ANALYZER DOES NOT CATCH RLOCKS WHEN THE MUTEX VARIABLE IS OUTSIDE OF SCOPE LIKE THIS
|
|
|
|
package testdata
|
|
|
|
import (
|
|
"sync"
|
|
)
|
|
|
|
var mutex *sync.RWMutex
|
|
|
|
func RLockFuncs() {
|
|
regularRLock()
|
|
nestedRLock1Level()
|
|
nestedRLock2Levels()
|
|
deferredRLock()
|
|
recursiveRLock(true)
|
|
}
|
|
|
|
func regularRLock() {
|
|
mutex.RLock()
|
|
mutex.RUnlock()
|
|
}
|
|
|
|
func nestedRLock1Level() {
|
|
mutex.RLock()
|
|
regularRLock() // this is a nested RLock, but the analyzer will not pick it up
|
|
mutex.RUnlock()
|
|
}
|
|
|
|
func nestedRLock2Levels() {
|
|
mutex.RLock()
|
|
callRegularRLock()
|
|
mutex.RUnlock()
|
|
}
|
|
|
|
func callRegularRLock() {
|
|
regularRLock()
|
|
}
|
|
|
|
func deferredRLock() {
|
|
mutex.RLock()
|
|
defer mutex.RUnlock()
|
|
regularRLock()
|
|
}
|
|
|
|
func recursiveRLock(run bool) {
|
|
mutex.RLock()
|
|
defer mutex.RUnlock()
|
|
if run {
|
|
recursiveRLock(!run)
|
|
}
|
|
}
|