mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2025-01-03 16:37:39 +00:00
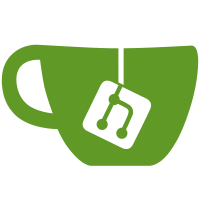
* begin move * use same import path * imports * regen protos * regen * no rename * generate ssz * gaz * fmt * edit build file * imports * modify * remove generated files * remove protos * edit imports in prysm * beacon chain all builds * edit script * add generated pbs * add replace rules * license for ethereumapis protos * change visibility * fmt * update build files to gaz ignore * use proper form * edit imports * wrap block * revert scripts * revert go mod
84 lines
2.3 KiB
Go
84 lines
2.3 KiB
Go
package validator
|
|
|
|
import (
|
|
"fmt"
|
|
"testing"
|
|
|
|
"github.com/prysmaticlabs/prysm/shared/copyutil"
|
|
|
|
"github.com/prysmaticlabs/go-bitfield"
|
|
ethpb "github.com/prysmaticlabs/prysm/proto/eth/v1alpha1"
|
|
aggtesting "github.com/prysmaticlabs/prysm/shared/aggregation/testing"
|
|
"github.com/prysmaticlabs/prysm/shared/featureconfig"
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
)
|
|
|
|
func BenchmarkProposerAtts_sortByProfitability(b *testing.B) {
|
|
bitlistLen := params.BeaconConfig().MaxValidatorsPerCommittee
|
|
|
|
tests := []struct {
|
|
name string
|
|
inputs []bitfield.Bitlist
|
|
}{
|
|
{
|
|
name: "256 attestations with single bit set",
|
|
inputs: aggtesting.BitlistsWithSingleBitSet(256, bitlistLen),
|
|
},
|
|
{
|
|
name: "256 attestations with 64 random bits set",
|
|
inputs: aggtesting.BitlistsWithSingleBitSet(256, bitlistLen),
|
|
},
|
|
{
|
|
name: "512 attestations with single bit set",
|
|
inputs: aggtesting.BitlistsWithSingleBitSet(512, bitlistLen),
|
|
},
|
|
{
|
|
name: "1024 attestations with 64 random bits set",
|
|
inputs: aggtesting.BitlistsWithMultipleBitSet(b, 1024, bitlistLen, 64),
|
|
},
|
|
{
|
|
name: "1024 attestations with 512 random bits set",
|
|
inputs: aggtesting.BitlistsWithMultipleBitSet(b, 1024, bitlistLen, 512),
|
|
},
|
|
{
|
|
name: "1024 attestations with 1000 random bits set",
|
|
inputs: aggtesting.BitlistsWithMultipleBitSet(b, 1024, bitlistLen, 1000),
|
|
},
|
|
}
|
|
|
|
runner := func(atts []*ethpb.Attestation) {
|
|
attsCopy := make(proposerAtts, len(atts))
|
|
for i, att := range atts {
|
|
attsCopy[i] = copyutil.CopyAttestation(att)
|
|
}
|
|
attsCopy.sortByProfitability()
|
|
}
|
|
|
|
for _, tt := range tests {
|
|
b.Run(fmt.Sprintf("naive_%s", tt.name), func(b *testing.B) {
|
|
b.StopTimer()
|
|
resetCfg := featureconfig.InitWithReset(&featureconfig.Flags{
|
|
ProposerAttsSelectionUsingMaxCover: false,
|
|
})
|
|
defer resetCfg()
|
|
atts := aggtesting.MakeAttestationsFromBitlists(tt.inputs)
|
|
b.StartTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
runner(atts)
|
|
}
|
|
})
|
|
b.Run(fmt.Sprintf("max-cover_%s", tt.name), func(b *testing.B) {
|
|
b.StopTimer()
|
|
resetCfg := featureconfig.InitWithReset(&featureconfig.Flags{
|
|
ProposerAttsSelectionUsingMaxCover: true,
|
|
})
|
|
defer resetCfg()
|
|
atts := aggtesting.MakeAttestationsFromBitlists(tt.inputs)
|
|
b.StartTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
runner(atts)
|
|
}
|
|
})
|
|
}
|
|
}
|