mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 04:47:18 +00:00
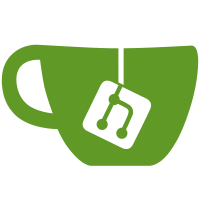
* Implemented new fork choice service and helpers * Added rest of the tests * Lint * Add back helpers test * Reformatted to doc, helpers and metrics.go * include new getter for block * create block filters from indices * give every block index a unique bucket * construct block indices by bucket mmap * almost done save for the block filters * include block filters, need a few more small touches for fetching the proper indices by bucket * full functionality to filter by parent root * tests pass when using the same logic as attestations * todo * proper todo formatting * first minimum slot range filter * slot range filters pass * more filter criteria passing * tests passing * add todos * all block tests pass and work * rem fmt * range retrieval test * fixed test conditions * instantiate the other buckets * simplify bucket lookups * deprecate non map code * revamp to remove old index prefixes * create indices from data * create indices from data * fetch block roots by slot range * better abstractions * simpler abstractions * roots rename * comment * preston feedback * Fixed existing tests * allow blocks without parent root * Cleaned up a few things * Removed todo * Lint * Cleaned up a few things * A few functions don't need to be exported * Gaz * Fixed visibility * Review feedback * Review feedback part1 * Raul's feedback, refactored OnBlock and OnAttestation to its own file * Fixed grammar * Lint * Implemented ReceiveAttestation * Use time.Time * Implemented ReceiveAttestation * All tests pass * Lint * Oooops * Typo
84 lines
2.5 KiB
Go
84 lines
2.5 KiB
Go
package blockchain
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"github.com/prysmaticlabs/go-ssz"
|
|
testDB "github.com/prysmaticlabs/prysm/beacon-chain/db/testing"
|
|
pb "github.com/prysmaticlabs/prysm/proto/beacon/p2p/v1"
|
|
ethpb "github.com/prysmaticlabs/prysm/proto/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil"
|
|
logTest "github.com/sirupsen/logrus/hooks/test"
|
|
"golang.org/x/net/context"
|
|
)
|
|
|
|
func TestReceiveAttestation_ProcessCorrectly(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
db := testDB.SetupDB(t)
|
|
defer testDB.TeardownDB(t, db)
|
|
ctx := context.Background()
|
|
|
|
chainService := setupBeaconChain(t, db)
|
|
chainService.forkChoiceStore = &store{}
|
|
|
|
b := ðpb.BeaconBlock{}
|
|
if err := chainService.beaconDB.SaveBlock(ctx, b); err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
root, err := ssz.SigningRoot(b)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if err := chainService.beaconDB.SaveState(ctx, &pb.BeaconState{}, root); err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
a := ðpb.Attestation{Data: ðpb.AttestationData{
|
|
Target: ðpb.Checkpoint{Root: root[:]},
|
|
Crosslink: ðpb.Crosslink{},
|
|
}}
|
|
if err := chainService.ReceiveAttestation(ctx, a); err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
testutil.AssertLogsContain(t, hook, "Finished updating fork choice store for attestation")
|
|
testutil.AssertLogsContain(t, hook, "Finished applying fork choice")
|
|
testutil.AssertLogsContain(t, hook, "Saved head info")
|
|
testutil.AssertLogsContain(t, hook, "Broadcasting attestation")
|
|
}
|
|
|
|
func TestReceiveAttestationNoPubsub_ProcessCorrectly(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
db := testDB.SetupDB(t)
|
|
defer testDB.TeardownDB(t, db)
|
|
ctx := context.Background()
|
|
|
|
chainService := setupBeaconChain(t, db)
|
|
chainService.forkChoiceStore = &store{}
|
|
|
|
b := ðpb.BeaconBlock{}
|
|
if err := chainService.beaconDB.SaveBlock(ctx, b); err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
root, err := ssz.SigningRoot(b)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if err := chainService.beaconDB.SaveState(ctx, &pb.BeaconState{}, root); err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
a := ðpb.Attestation{Data: ðpb.AttestationData{
|
|
Target: ðpb.Checkpoint{Root: root[:]},
|
|
Crosslink: ðpb.Crosslink{},
|
|
}}
|
|
if err := chainService.ReceiveAttestationNoPubsub(ctx, a); err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
testutil.AssertLogsContain(t, hook, "Finished updating fork choice store for attestation")
|
|
testutil.AssertLogsContain(t, hook, "Finished applying fork choice")
|
|
testutil.AssertLogsContain(t, hook, "Saved head info")
|
|
testutil.AssertLogsDoNotContain(t, hook, "Broadcasting attestation")
|
|
}
|