mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2025-01-10 11:41:21 +00:00
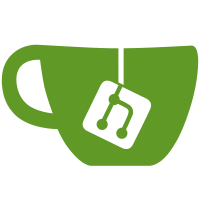
* rename proto * p header * regen * regen ssz * fix randao * random name changes * bazel builds * bt * incorrect prev randao Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com>
63 lines
2.3 KiB
Go
63 lines
2.3 KiB
Go
package validator
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/core/transition/interop"
|
|
fieldparams "github.com/prysmaticlabs/prysm/config/fieldparams"
|
|
"github.com/prysmaticlabs/prysm/config/params"
|
|
enginev1 "github.com/prysmaticlabs/prysm/proto/engine/v1"
|
|
ethpb "github.com/prysmaticlabs/prysm/proto/prysm/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/proto/prysm/v1alpha1/wrapper"
|
|
)
|
|
|
|
func (vs *Server) getBellatrixBeaconBlock(ctx context.Context, req *ethpb.BlockRequest) (*ethpb.BeaconBlockBellatrix, error) {
|
|
altairBlk, err := vs.buildAltairBeaconBlock(ctx, req)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
blk := ðpb.BeaconBlockBellatrix{
|
|
Slot: altairBlk.Slot,
|
|
ProposerIndex: altairBlk.ProposerIndex,
|
|
ParentRoot: altairBlk.ParentRoot,
|
|
StateRoot: params.BeaconConfig().ZeroHash[:],
|
|
Body: ðpb.BeaconBlockBodyBellatrix{
|
|
RandaoReveal: altairBlk.Body.RandaoReveal,
|
|
Eth1Data: altairBlk.Body.Eth1Data,
|
|
Graffiti: altairBlk.Body.Graffiti,
|
|
ProposerSlashings: altairBlk.Body.ProposerSlashings,
|
|
AttesterSlashings: altairBlk.Body.AttesterSlashings,
|
|
Attestations: altairBlk.Body.Attestations,
|
|
Deposits: altairBlk.Body.Deposits,
|
|
VoluntaryExits: altairBlk.Body.VoluntaryExits,
|
|
SyncAggregate: altairBlk.Body.SyncAggregate,
|
|
ExecutionPayload: &enginev1.ExecutionPayload{
|
|
ParentHash: make([]byte, fieldparams.RootLength),
|
|
FeeRecipient: make([]byte, fieldparams.FeeRecipientLength),
|
|
StateRoot: make([]byte, fieldparams.RootLength),
|
|
ReceiptsRoot: make([]byte, fieldparams.RootLength),
|
|
LogsBloom: make([]byte, fieldparams.LogsBloomLength),
|
|
PrevRandao: make([]byte, fieldparams.RootLength),
|
|
BaseFeePerGas: make([]byte, fieldparams.RootLength),
|
|
BlockHash: make([]byte, fieldparams.RootLength),
|
|
}, // TODO(9853) Insert real execution payload.
|
|
},
|
|
}
|
|
// Compute state root with the newly constructed block.
|
|
wsb, err := wrapper.WrappedBellatrixSignedBeaconBlock(
|
|
ðpb.SignedBeaconBlockBellatrix{Block: blk, Signature: make([]byte, 96)},
|
|
)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
stateRoot, err := vs.computeStateRoot(ctx, wsb)
|
|
if err != nil {
|
|
interop.WriteBlockToDisk(wsb, true /*failed*/)
|
|
return nil, fmt.Errorf("could not compute state root: %v", err)
|
|
}
|
|
blk.StateRoot = stateRoot
|
|
return blk, nil
|
|
}
|