mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-28 14:17:17 +00:00
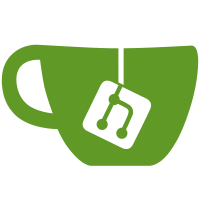
* update beacon-state proto to new spec * AttestationData * use bazel's pb.go * boundry -> boundary
485 lines
19 KiB
Go
Executable File
485 lines
19 KiB
Go
Executable File
// Code generated by protoc-gen-go. DO NOT EDIT.
|
|
// source: proto/beacon/p2p/v1/messages.proto
|
|
|
|
package v1
|
|
|
|
import proto "github.com/golang/protobuf/proto"
|
|
import fmt "fmt"
|
|
import math "math"
|
|
|
|
// Reference imports to suppress errors if they are not otherwise used.
|
|
var _ = proto.Marshal
|
|
var _ = fmt.Errorf
|
|
var _ = math.Inf
|
|
|
|
// This is a compile-time assertion to ensure that this generated file
|
|
// is compatible with the proto package it is being compiled against.
|
|
// A compilation error at this line likely means your copy of the
|
|
// proto package needs to be updated.
|
|
const _ = proto.ProtoPackageIsVersion2 // please upgrade the proto package
|
|
|
|
type Topic int32
|
|
|
|
const (
|
|
Topic_UNKNOWN Topic = 0
|
|
Topic_BEACON_BLOCK_ANNOUNCE Topic = 1
|
|
Topic_BEACON_BLOCK_REQUEST Topic = 2
|
|
Topic_BEACON_BLOCK_REQUEST_BY_SLOT_NUMBER Topic = 3
|
|
Topic_BEACON_BLOCK_RESPONSE Topic = 4
|
|
Topic_CHAIN_HEAD_REQUEST Topic = 5
|
|
Topic_CHAIN_HEAD_RESPONSE Topic = 6
|
|
Topic_BEACON_STATE_HASH_ANNOUNCE Topic = 7
|
|
Topic_BEACON_STATE_REQUEST Topic = 8
|
|
Topic_BEACON_STATE_RESPONSE Topic = 9
|
|
)
|
|
|
|
var Topic_name = map[int32]string{
|
|
0: "UNKNOWN",
|
|
1: "BEACON_BLOCK_ANNOUNCE",
|
|
2: "BEACON_BLOCK_REQUEST",
|
|
3: "BEACON_BLOCK_REQUEST_BY_SLOT_NUMBER",
|
|
4: "BEACON_BLOCK_RESPONSE",
|
|
5: "CHAIN_HEAD_REQUEST",
|
|
6: "CHAIN_HEAD_RESPONSE",
|
|
7: "BEACON_STATE_HASH_ANNOUNCE",
|
|
8: "BEACON_STATE_REQUEST",
|
|
9: "BEACON_STATE_RESPONSE",
|
|
}
|
|
var Topic_value = map[string]int32{
|
|
"UNKNOWN": 0,
|
|
"BEACON_BLOCK_ANNOUNCE": 1,
|
|
"BEACON_BLOCK_REQUEST": 2,
|
|
"BEACON_BLOCK_REQUEST_BY_SLOT_NUMBER": 3,
|
|
"BEACON_BLOCK_RESPONSE": 4,
|
|
"CHAIN_HEAD_REQUEST": 5,
|
|
"CHAIN_HEAD_RESPONSE": 6,
|
|
"BEACON_STATE_HASH_ANNOUNCE": 7,
|
|
"BEACON_STATE_REQUEST": 8,
|
|
"BEACON_STATE_RESPONSE": 9,
|
|
}
|
|
|
|
func (x Topic) String() string {
|
|
return proto.EnumName(Topic_name, int32(x))
|
|
}
|
|
func (Topic) EnumDescriptor() ([]byte, []int) {
|
|
return fileDescriptor_messages_17523cb9213d7345, []int{0}
|
|
}
|
|
|
|
type BeaconBlockAnnounce struct {
|
|
Hash []byte `protobuf:"bytes,1,opt,name=hash,proto3" json:"hash,omitempty"`
|
|
SlotNumber uint64 `protobuf:"varint,2,opt,name=slot_number,json=slotNumber,proto3" json:"slot_number,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *BeaconBlockAnnounce) Reset() { *m = BeaconBlockAnnounce{} }
|
|
func (m *BeaconBlockAnnounce) String() string { return proto.CompactTextString(m) }
|
|
func (*BeaconBlockAnnounce) ProtoMessage() {}
|
|
func (*BeaconBlockAnnounce) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_messages_17523cb9213d7345, []int{0}
|
|
}
|
|
func (m *BeaconBlockAnnounce) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_BeaconBlockAnnounce.Unmarshal(m, b)
|
|
}
|
|
func (m *BeaconBlockAnnounce) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_BeaconBlockAnnounce.Marshal(b, m, deterministic)
|
|
}
|
|
func (dst *BeaconBlockAnnounce) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_BeaconBlockAnnounce.Merge(dst, src)
|
|
}
|
|
func (m *BeaconBlockAnnounce) XXX_Size() int {
|
|
return xxx_messageInfo_BeaconBlockAnnounce.Size(m)
|
|
}
|
|
func (m *BeaconBlockAnnounce) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_BeaconBlockAnnounce.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_BeaconBlockAnnounce proto.InternalMessageInfo
|
|
|
|
func (m *BeaconBlockAnnounce) GetHash() []byte {
|
|
if m != nil {
|
|
return m.Hash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *BeaconBlockAnnounce) GetSlotNumber() uint64 {
|
|
if m != nil {
|
|
return m.SlotNumber
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type BeaconBlockRequest struct {
|
|
Hash []byte `protobuf:"bytes,1,opt,name=hash,proto3" json:"hash,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *BeaconBlockRequest) Reset() { *m = BeaconBlockRequest{} }
|
|
func (m *BeaconBlockRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*BeaconBlockRequest) ProtoMessage() {}
|
|
func (*BeaconBlockRequest) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_messages_17523cb9213d7345, []int{1}
|
|
}
|
|
func (m *BeaconBlockRequest) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_BeaconBlockRequest.Unmarshal(m, b)
|
|
}
|
|
func (m *BeaconBlockRequest) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_BeaconBlockRequest.Marshal(b, m, deterministic)
|
|
}
|
|
func (dst *BeaconBlockRequest) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_BeaconBlockRequest.Merge(dst, src)
|
|
}
|
|
func (m *BeaconBlockRequest) XXX_Size() int {
|
|
return xxx_messageInfo_BeaconBlockRequest.Size(m)
|
|
}
|
|
func (m *BeaconBlockRequest) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_BeaconBlockRequest.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_BeaconBlockRequest proto.InternalMessageInfo
|
|
|
|
func (m *BeaconBlockRequest) GetHash() []byte {
|
|
if m != nil {
|
|
return m.Hash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type BeaconBlockRequestBySlotNumber struct {
|
|
SlotNumber uint64 `protobuf:"varint,1,opt,name=slot_number,json=slotNumber,proto3" json:"slot_number,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *BeaconBlockRequestBySlotNumber) Reset() { *m = BeaconBlockRequestBySlotNumber{} }
|
|
func (m *BeaconBlockRequestBySlotNumber) String() string { return proto.CompactTextString(m) }
|
|
func (*BeaconBlockRequestBySlotNumber) ProtoMessage() {}
|
|
func (*BeaconBlockRequestBySlotNumber) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_messages_17523cb9213d7345, []int{2}
|
|
}
|
|
func (m *BeaconBlockRequestBySlotNumber) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_BeaconBlockRequestBySlotNumber.Unmarshal(m, b)
|
|
}
|
|
func (m *BeaconBlockRequestBySlotNumber) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_BeaconBlockRequestBySlotNumber.Marshal(b, m, deterministic)
|
|
}
|
|
func (dst *BeaconBlockRequestBySlotNumber) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_BeaconBlockRequestBySlotNumber.Merge(dst, src)
|
|
}
|
|
func (m *BeaconBlockRequestBySlotNumber) XXX_Size() int {
|
|
return xxx_messageInfo_BeaconBlockRequestBySlotNumber.Size(m)
|
|
}
|
|
func (m *BeaconBlockRequestBySlotNumber) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_BeaconBlockRequestBySlotNumber.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_BeaconBlockRequestBySlotNumber proto.InternalMessageInfo
|
|
|
|
func (m *BeaconBlockRequestBySlotNumber) GetSlotNumber() uint64 {
|
|
if m != nil {
|
|
return m.SlotNumber
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type BeaconBlockResponse struct {
|
|
Block *BeaconBlock `protobuf:"bytes,1,opt,name=block,proto3" json:"block,omitempty"`
|
|
Attestation *AggregatedAttestation `protobuf:"bytes,2,opt,name=attestation,proto3" json:"attestation,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *BeaconBlockResponse) Reset() { *m = BeaconBlockResponse{} }
|
|
func (m *BeaconBlockResponse) String() string { return proto.CompactTextString(m) }
|
|
func (*BeaconBlockResponse) ProtoMessage() {}
|
|
func (*BeaconBlockResponse) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_messages_17523cb9213d7345, []int{3}
|
|
}
|
|
func (m *BeaconBlockResponse) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_BeaconBlockResponse.Unmarshal(m, b)
|
|
}
|
|
func (m *BeaconBlockResponse) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_BeaconBlockResponse.Marshal(b, m, deterministic)
|
|
}
|
|
func (dst *BeaconBlockResponse) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_BeaconBlockResponse.Merge(dst, src)
|
|
}
|
|
func (m *BeaconBlockResponse) XXX_Size() int {
|
|
return xxx_messageInfo_BeaconBlockResponse.Size(m)
|
|
}
|
|
func (m *BeaconBlockResponse) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_BeaconBlockResponse.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_BeaconBlockResponse proto.InternalMessageInfo
|
|
|
|
func (m *BeaconBlockResponse) GetBlock() *BeaconBlock {
|
|
if m != nil {
|
|
return m.Block
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *BeaconBlockResponse) GetAttestation() *AggregatedAttestation {
|
|
if m != nil {
|
|
return m.Attestation
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type ChainHeadRequest struct {
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *ChainHeadRequest) Reset() { *m = ChainHeadRequest{} }
|
|
func (m *ChainHeadRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*ChainHeadRequest) ProtoMessage() {}
|
|
func (*ChainHeadRequest) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_messages_17523cb9213d7345, []int{4}
|
|
}
|
|
func (m *ChainHeadRequest) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_ChainHeadRequest.Unmarshal(m, b)
|
|
}
|
|
func (m *ChainHeadRequest) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_ChainHeadRequest.Marshal(b, m, deterministic)
|
|
}
|
|
func (dst *ChainHeadRequest) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_ChainHeadRequest.Merge(dst, src)
|
|
}
|
|
func (m *ChainHeadRequest) XXX_Size() int {
|
|
return xxx_messageInfo_ChainHeadRequest.Size(m)
|
|
}
|
|
func (m *ChainHeadRequest) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_ChainHeadRequest.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_ChainHeadRequest proto.InternalMessageInfo
|
|
|
|
type ChainHeadResponse struct {
|
|
Hash []byte `protobuf:"bytes,1,opt,name=hash,proto3" json:"hash,omitempty"`
|
|
Slot uint64 `protobuf:"varint,2,opt,name=slot,proto3" json:"slot,omitempty"`
|
|
Block *BeaconBlock `protobuf:"bytes,3,opt,name=block,proto3" json:"block,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *ChainHeadResponse) Reset() { *m = ChainHeadResponse{} }
|
|
func (m *ChainHeadResponse) String() string { return proto.CompactTextString(m) }
|
|
func (*ChainHeadResponse) ProtoMessage() {}
|
|
func (*ChainHeadResponse) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_messages_17523cb9213d7345, []int{5}
|
|
}
|
|
func (m *ChainHeadResponse) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_ChainHeadResponse.Unmarshal(m, b)
|
|
}
|
|
func (m *ChainHeadResponse) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_ChainHeadResponse.Marshal(b, m, deterministic)
|
|
}
|
|
func (dst *ChainHeadResponse) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_ChainHeadResponse.Merge(dst, src)
|
|
}
|
|
func (m *ChainHeadResponse) XXX_Size() int {
|
|
return xxx_messageInfo_ChainHeadResponse.Size(m)
|
|
}
|
|
func (m *ChainHeadResponse) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_ChainHeadResponse.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_ChainHeadResponse proto.InternalMessageInfo
|
|
|
|
func (m *ChainHeadResponse) GetHash() []byte {
|
|
if m != nil {
|
|
return m.Hash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *ChainHeadResponse) GetSlot() uint64 {
|
|
if m != nil {
|
|
return m.Slot
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (m *ChainHeadResponse) GetBlock() *BeaconBlock {
|
|
if m != nil {
|
|
return m.Block
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type BeaconStateHashAnnounce struct {
|
|
Hash []byte `protobuf:"bytes,1,opt,name=hash,proto3" json:"hash,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *BeaconStateHashAnnounce) Reset() { *m = BeaconStateHashAnnounce{} }
|
|
func (m *BeaconStateHashAnnounce) String() string { return proto.CompactTextString(m) }
|
|
func (*BeaconStateHashAnnounce) ProtoMessage() {}
|
|
func (*BeaconStateHashAnnounce) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_messages_17523cb9213d7345, []int{6}
|
|
}
|
|
func (m *BeaconStateHashAnnounce) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_BeaconStateHashAnnounce.Unmarshal(m, b)
|
|
}
|
|
func (m *BeaconStateHashAnnounce) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_BeaconStateHashAnnounce.Marshal(b, m, deterministic)
|
|
}
|
|
func (dst *BeaconStateHashAnnounce) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_BeaconStateHashAnnounce.Merge(dst, src)
|
|
}
|
|
func (m *BeaconStateHashAnnounce) XXX_Size() int {
|
|
return xxx_messageInfo_BeaconStateHashAnnounce.Size(m)
|
|
}
|
|
func (m *BeaconStateHashAnnounce) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_BeaconStateHashAnnounce.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_BeaconStateHashAnnounce proto.InternalMessageInfo
|
|
|
|
func (m *BeaconStateHashAnnounce) GetHash() []byte {
|
|
if m != nil {
|
|
return m.Hash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type BeaconStateRequest struct {
|
|
Hash []byte `protobuf:"bytes,1,opt,name=hash,proto3" json:"hash,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *BeaconStateRequest) Reset() { *m = BeaconStateRequest{} }
|
|
func (m *BeaconStateRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*BeaconStateRequest) ProtoMessage() {}
|
|
func (*BeaconStateRequest) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_messages_17523cb9213d7345, []int{7}
|
|
}
|
|
func (m *BeaconStateRequest) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_BeaconStateRequest.Unmarshal(m, b)
|
|
}
|
|
func (m *BeaconStateRequest) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_BeaconStateRequest.Marshal(b, m, deterministic)
|
|
}
|
|
func (dst *BeaconStateRequest) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_BeaconStateRequest.Merge(dst, src)
|
|
}
|
|
func (m *BeaconStateRequest) XXX_Size() int {
|
|
return xxx_messageInfo_BeaconStateRequest.Size(m)
|
|
}
|
|
func (m *BeaconStateRequest) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_BeaconStateRequest.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_BeaconStateRequest proto.InternalMessageInfo
|
|
|
|
func (m *BeaconStateRequest) GetHash() []byte {
|
|
if m != nil {
|
|
return m.Hash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type BeaconStateResponse struct {
|
|
BeaconState *BeaconState `protobuf:"bytes,1,opt,name=beacon_state,json=beaconState,proto3" json:"beacon_state,omitempty"`
|
|
XXX_NoUnkeyedLiteral struct{} `json:"-"`
|
|
XXX_unrecognized []byte `json:"-"`
|
|
XXX_sizecache int32 `json:"-"`
|
|
}
|
|
|
|
func (m *BeaconStateResponse) Reset() { *m = BeaconStateResponse{} }
|
|
func (m *BeaconStateResponse) String() string { return proto.CompactTextString(m) }
|
|
func (*BeaconStateResponse) ProtoMessage() {}
|
|
func (*BeaconStateResponse) Descriptor() ([]byte, []int) {
|
|
return fileDescriptor_messages_17523cb9213d7345, []int{8}
|
|
}
|
|
func (m *BeaconStateResponse) XXX_Unmarshal(b []byte) error {
|
|
return xxx_messageInfo_BeaconStateResponse.Unmarshal(m, b)
|
|
}
|
|
func (m *BeaconStateResponse) XXX_Marshal(b []byte, deterministic bool) ([]byte, error) {
|
|
return xxx_messageInfo_BeaconStateResponse.Marshal(b, m, deterministic)
|
|
}
|
|
func (dst *BeaconStateResponse) XXX_Merge(src proto.Message) {
|
|
xxx_messageInfo_BeaconStateResponse.Merge(dst, src)
|
|
}
|
|
func (m *BeaconStateResponse) XXX_Size() int {
|
|
return xxx_messageInfo_BeaconStateResponse.Size(m)
|
|
}
|
|
func (m *BeaconStateResponse) XXX_DiscardUnknown() {
|
|
xxx_messageInfo_BeaconStateResponse.DiscardUnknown(m)
|
|
}
|
|
|
|
var xxx_messageInfo_BeaconStateResponse proto.InternalMessageInfo
|
|
|
|
func (m *BeaconStateResponse) GetBeaconState() *BeaconState {
|
|
if m != nil {
|
|
return m.BeaconState
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func init() {
|
|
proto.RegisterType((*BeaconBlockAnnounce)(nil), "ethereum.beacon.p2p.v1.BeaconBlockAnnounce")
|
|
proto.RegisterType((*BeaconBlockRequest)(nil), "ethereum.beacon.p2p.v1.BeaconBlockRequest")
|
|
proto.RegisterType((*BeaconBlockRequestBySlotNumber)(nil), "ethereum.beacon.p2p.v1.BeaconBlockRequestBySlotNumber")
|
|
proto.RegisterType((*BeaconBlockResponse)(nil), "ethereum.beacon.p2p.v1.BeaconBlockResponse")
|
|
proto.RegisterType((*ChainHeadRequest)(nil), "ethereum.beacon.p2p.v1.ChainHeadRequest")
|
|
proto.RegisterType((*ChainHeadResponse)(nil), "ethereum.beacon.p2p.v1.ChainHeadResponse")
|
|
proto.RegisterType((*BeaconStateHashAnnounce)(nil), "ethereum.beacon.p2p.v1.BeaconStateHashAnnounce")
|
|
proto.RegisterType((*BeaconStateRequest)(nil), "ethereum.beacon.p2p.v1.BeaconStateRequest")
|
|
proto.RegisterType((*BeaconStateResponse)(nil), "ethereum.beacon.p2p.v1.BeaconStateResponse")
|
|
proto.RegisterEnum("ethereum.beacon.p2p.v1.Topic", Topic_name, Topic_value)
|
|
}
|
|
|
|
func init() {
|
|
proto.RegisterFile("proto/beacon/p2p/v1/messages.proto", fileDescriptor_messages_17523cb9213d7345)
|
|
}
|
|
|
|
var fileDescriptor_messages_17523cb9213d7345 = []byte{
|
|
// 482 bytes of a gzipped FileDescriptorProto
|
|
0x1f, 0x8b, 0x08, 0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0xff, 0x94, 0x53, 0xc1, 0x6e, 0xd3, 0x40,
|
|
0x14, 0xc4, 0x69, 0xd2, 0xc2, 0x73, 0x0f, 0x66, 0x0b, 0x6d, 0xe8, 0xa1, 0xad, 0xdc, 0x03, 0x11,
|
|
0x52, 0x1d, 0x35, 0x9c, 0x38, 0xae, 0x83, 0x91, 0xa1, 0x65, 0x0d, 0xb6, 0x23, 0xc4, 0x01, 0x59,
|
|
0xeb, 0xf4, 0x29, 0x8e, 0x68, 0x6c, 0x93, 0xdd, 0x44, 0xea, 0x77, 0xf0, 0x05, 0xfc, 0x29, 0xca,
|
|
0xda, 0xa9, 0x1d, 0x62, 0x2a, 0xb8, 0x39, 0x33, 0x6f, 0x66, 0x67, 0xde, 0x53, 0xc0, 0xcc, 0xe7,
|
|
0x99, 0xcc, 0xfa, 0x31, 0xf2, 0x71, 0x96, 0xf6, 0xf3, 0x41, 0xde, 0x5f, 0x5e, 0xf6, 0x67, 0x28,
|
|
0x04, 0x9f, 0xa0, 0xb0, 0x14, 0x49, 0x0e, 0x51, 0x26, 0x38, 0xc7, 0xc5, 0xcc, 0x2a, 0xc6, 0xac,
|
|
0x7c, 0x90, 0x5b, 0xcb, 0xcb, 0xe3, 0xd3, 0x26, 0xad, 0xbc, 0xcb, 0xd7, 0x42, 0xf3, 0x03, 0x1c,
|
|
0xd8, 0x8a, 0xb4, 0x6f, 0xb3, 0xf1, 0x77, 0x9a, 0xa6, 0xd9, 0x22, 0x1d, 0x23, 0x21, 0xd0, 0x4e,
|
|
0xb8, 0x48, 0xba, 0xda, 0x99, 0xd6, 0xdb, 0xf7, 0xd5, 0x37, 0x39, 0x05, 0x5d, 0xdc, 0x66, 0x32,
|
|
0x4a, 0x17, 0xb3, 0x18, 0xe7, 0xdd, 0xd6, 0x99, 0xd6, 0x6b, 0xfb, 0xb0, 0x82, 0x98, 0x42, 0xcc,
|
|
0x1e, 0x90, 0x9a, 0x97, 0x8f, 0x3f, 0x16, 0x28, 0x64, 0x93, 0x95, 0x49, 0xe1, 0x64, 0x7b, 0xd2,
|
|
0xbe, 0x0b, 0xee, 0xbd, 0xfe, 0x7c, 0x4c, 0xdb, 0x7a, 0xec, 0x97, 0xb6, 0x91, 0xdc, 0x47, 0x91,
|
|
0x67, 0xa9, 0x40, 0xf2, 0x06, 0x3a, 0xf1, 0x0a, 0x50, 0x12, 0x7d, 0x70, 0x6e, 0x35, 0x6f, 0xc6,
|
|
0xaa, 0x6b, 0x0b, 0x05, 0xf1, 0x40, 0xe7, 0x52, 0xa2, 0x90, 0x5c, 0x4e, 0xb3, 0x54, 0x15, 0xd4,
|
|
0x07, 0x17, 0x7f, 0x33, 0xa0, 0x93, 0xc9, 0x1c, 0x27, 0x5c, 0xe2, 0x0d, 0xad, 0x44, 0x7e, 0xdd,
|
|
0xc1, 0x24, 0x60, 0x0c, 0x13, 0x3e, 0x4d, 0x5d, 0xe4, 0x37, 0x65, 0x49, 0x73, 0x09, 0x4f, 0x6b,
|
|
0x58, 0x19, 0xba, 0x69, 0xdd, 0x04, 0xda, 0xab, 0xba, 0xe5, 0x9e, 0xd5, 0x77, 0x55, 0x6e, 0xe7,
|
|
0x7f, 0xcb, 0x99, 0x17, 0x70, 0x54, 0xa0, 0x81, 0xe4, 0x12, 0x5d, 0x2e, 0x92, 0x87, 0x8e, 0x5d,
|
|
0xdd, 0x52, 0x8d, 0x3f, 0x74, 0xcb, 0x6f, 0xeb, 0x3b, 0x94, 0x93, 0x65, 0xa5, 0x77, 0xb0, 0x5f,
|
|
0x64, 0x8a, 0x56, 0xdb, 0xc0, 0x7f, 0x3b, 0x47, 0x61, 0xa1, 0xc7, 0xd5, 0x8f, 0x57, 0x3f, 0x5b,
|
|
0xd0, 0x09, 0xb3, 0x7c, 0x3a, 0x26, 0x3a, 0xec, 0x8d, 0xd8, 0x15, 0xf3, 0xbe, 0x30, 0xe3, 0x11,
|
|
0x79, 0x01, 0xcf, 0x6d, 0x87, 0x0e, 0x3d, 0x16, 0xd9, 0xd7, 0xde, 0xf0, 0x2a, 0xa2, 0x8c, 0x79,
|
|
0x23, 0x36, 0x74, 0x0c, 0x8d, 0x74, 0xe1, 0xd9, 0x06, 0xe5, 0x3b, 0x9f, 0x47, 0x4e, 0x10, 0x1a,
|
|
0x2d, 0xf2, 0x12, 0xce, 0x9b, 0x98, 0xc8, 0xfe, 0x1a, 0x05, 0xd7, 0x5e, 0x18, 0xb1, 0xd1, 0x47,
|
|
0xdb, 0xf1, 0x8d, 0x9d, 0x2d, 0x77, 0xdf, 0x09, 0x3e, 0x79, 0x2c, 0x70, 0x8c, 0x36, 0x39, 0x04,
|
|
0x32, 0x74, 0xe9, 0x7b, 0x16, 0xb9, 0x0e, 0x7d, 0x7b, 0xef, 0xdd, 0x21, 0x47, 0x70, 0xb0, 0x81,
|
|
0x97, 0x82, 0x5d, 0x72, 0x02, 0xc7, 0xa5, 0x57, 0x10, 0xd2, 0xd0, 0x89, 0x5c, 0x1a, 0xb8, 0x55,
|
|
0xdc, 0xbd, 0x5a, 0xdc, 0x82, 0x5f, 0x5b, 0x3e, 0xae, 0xa5, 0x58, 0x33, 0xa5, 0xe9, 0x93, 0x78,
|
|
0x57, 0xfd, 0x7b, 0x5f, 0xff, 0x0e, 0x00, 0x00, 0xff, 0xff, 0x03, 0x6d, 0x1d, 0x09, 0x1c, 0x04,
|
|
0x00, 0x00,
|
|
}
|