mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
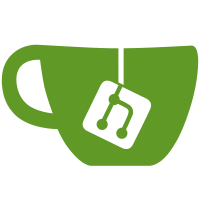
* begin move * use same import path * imports * regen protos * regen * no rename * generate ssz * gaz * fmt * edit build file * imports * modify * remove generated files * remove protos * edit imports in prysm * beacon chain all builds * edit script * add generated pbs * add replace rules * license for ethereumapis protos * change visibility * fmt * update build files to gaz ignore * use proper form * edit imports * wrap block * revert scripts * revert go mod
83 lines
2.0 KiB
Go
83 lines
2.0 KiB
Go
package migration
|
|
|
|
import (
|
|
"testing"
|
|
|
|
v1 "github.com/prysmaticlabs/prysm/proto/eth/v1"
|
|
eth "github.com/prysmaticlabs/prysm/proto/eth/v1alpha1"
|
|
)
|
|
|
|
func TestV1Alpha1ConnectionStateToV1(t *testing.T) {
|
|
tests := []struct {
|
|
name string
|
|
connState eth.ConnectionState
|
|
want v1.ConnectionState
|
|
}{
|
|
{
|
|
name: "DISCONNECTED",
|
|
connState: eth.ConnectionState_DISCONNECTED,
|
|
want: v1.ConnectionState_DISCONNECTED,
|
|
},
|
|
{
|
|
name: "CONNECTED",
|
|
connState: eth.ConnectionState_CONNECTED,
|
|
want: v1.ConnectionState_CONNECTED,
|
|
},
|
|
{
|
|
name: "CONNECTING",
|
|
connState: eth.ConnectionState_CONNECTING,
|
|
want: v1.ConnectionState_CONNECTING,
|
|
},
|
|
{
|
|
name: "DISCONNECTING",
|
|
connState: eth.ConnectionState_DISCONNECTING,
|
|
want: v1.ConnectionState_DISCONNECTING,
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
if got := V1Alpha1ConnectionStateToV1(tt.connState); got != tt.want {
|
|
t.Errorf("V1Alpha1ConnectionStateToV1() = %v, want %v", got, tt.want)
|
|
}
|
|
})
|
|
}
|
|
}
|
|
|
|
func TestV1Alpha1PeerDirectionToV1(t *testing.T) {
|
|
tests := []struct {
|
|
name string
|
|
peerDirection eth.PeerDirection
|
|
want v1.PeerDirection
|
|
wantErr bool
|
|
}{
|
|
{
|
|
name: "UNKNOWN",
|
|
peerDirection: eth.PeerDirection_UNKNOWN,
|
|
want: 0,
|
|
wantErr: true,
|
|
},
|
|
{
|
|
name: "INBOUND",
|
|
peerDirection: eth.PeerDirection_INBOUND,
|
|
want: v1.PeerDirection_INBOUND,
|
|
},
|
|
{
|
|
name: "OUTBOUND",
|
|
peerDirection: eth.PeerDirection_OUTBOUND,
|
|
want: v1.PeerDirection_OUTBOUND,
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
got, err := V1Alpha1PeerDirectionToV1(tt.peerDirection)
|
|
if (err != nil) != tt.wantErr {
|
|
t.Errorf("V1Alpha1PeerDirectionToV1() error = %v, wantErr %v", err, tt.wantErr)
|
|
return
|
|
}
|
|
if got != tt.want {
|
|
t.Errorf("V1Alpha1PeerDirectionToV1() got = %v, want %v", got, tt.want)
|
|
}
|
|
})
|
|
}
|
|
}
|