mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-28 14:17:17 +00:00
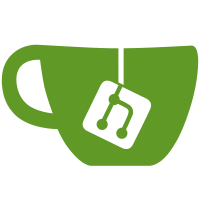
* Update io_kubernetes_build commit hash to 1246899 * Update dependency build_bazel_rules_nodejs to v0.33.1 * Update dependency com_github_hashicorp_golang_lru to v0.5.1 * Update libp2p * Update io_bazel_rules_k8s commit hash to e68d5d7 * Starting to remove old protos * Bazel build proto passes * Fixing pb version * Cleaned up core package * Fixing tests * 6 tests failing * Update proto bugs * Fixed incorrect validator ordering proto * Sync with master * Update go-ssz commit * Removed bad copies from v1alpha1 folder * add json spec json to pb handler * add nested proto example * proto/testing test works * fix refactoring build failures * use merged ssz * push latest changes * used forked json encoding * used forked json encoding * fix warning * fix build issues * fix test and lint * fix build * lint
93 lines
2.3 KiB
Go
93 lines
2.3 KiB
Go
package blocks
|
|
|
|
import (
|
|
"bytes"
|
|
"testing"
|
|
|
|
"github.com/gogo/protobuf/proto"
|
|
"github.com/prysmaticlabs/go-ssz"
|
|
ethpb "github.com/prysmaticlabs/prysm/proto/eth/v1alpha1"
|
|
)
|
|
|
|
func TestGenesisBlock_InitializedCorrectly(t *testing.T) {
|
|
stateHash := []byte{0}
|
|
b1 := NewGenesisBlock(stateHash)
|
|
|
|
if b1.ParentRoot == nil {
|
|
t.Error("genesis block missing ParentHash field")
|
|
}
|
|
|
|
if !bytes.Equal(b1.StateRoot, stateHash) {
|
|
t.Error("genesis block StateRootHash32 isn't initialized correctly")
|
|
}
|
|
}
|
|
|
|
func TestHeaderFromBlock(t *testing.T) {
|
|
dummyBody := ðpb.BeaconBlockBody{
|
|
Eth1Data: ðpb.Eth1Data{},
|
|
Graffiti: []byte{},
|
|
RandaoReveal: []byte("Reveal"),
|
|
AttesterSlashings: []*ethpb.AttesterSlashing{},
|
|
ProposerSlashings: []*ethpb.ProposerSlashing{},
|
|
Attestations: []*ethpb.Attestation{},
|
|
Transfers: []*ethpb.Transfer{},
|
|
Deposits: []*ethpb.Deposit{},
|
|
VoluntaryExits: []*ethpb.VoluntaryExit{},
|
|
}
|
|
|
|
dummyBlock := ðpb.BeaconBlock{
|
|
Slot: 10,
|
|
Signature: []byte{'S'},
|
|
ParentRoot: []byte("Parent"),
|
|
StateRoot: []byte("State"),
|
|
Body: dummyBody,
|
|
}
|
|
|
|
header, err := HeaderFromBlock(dummyBlock)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
expectedHeader := ðpb.BeaconBlockHeader{
|
|
Slot: dummyBlock.Slot,
|
|
Signature: dummyBlock.Signature,
|
|
ParentRoot: dummyBlock.ParentRoot,
|
|
StateRoot: dummyBlock.StateRoot,
|
|
}
|
|
|
|
bodyRoot, err := ssz.HashTreeRoot(dummyBody)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
expectedHeader.BodyRoot = bodyRoot[:]
|
|
|
|
if !proto.Equal(expectedHeader, header) {
|
|
t.Errorf("Expected Header not Equal to Retrieved Header. Expected %v , Got %v",
|
|
proto.MarshalTextString(expectedHeader), proto.MarshalTextString(header))
|
|
}
|
|
}
|
|
|
|
func TestBlockFromHeader(t *testing.T) {
|
|
dummyHeader := ðpb.BeaconBlockHeader{
|
|
Slot: 10,
|
|
Signature: []byte{'S'},
|
|
ParentRoot: []byte("Parent"),
|
|
StateRoot: []byte("State"),
|
|
}
|
|
|
|
block := BlockFromHeader(dummyHeader)
|
|
|
|
expectedBlock := ðpb.BeaconBlock{
|
|
Slot: dummyHeader.Slot,
|
|
Signature: dummyHeader.Signature,
|
|
ParentRoot: dummyHeader.ParentRoot,
|
|
StateRoot: dummyHeader.StateRoot,
|
|
}
|
|
|
|
if !proto.Equal(expectedBlock, block) {
|
|
t.Errorf("Expected block not equal to retrieved block. Expected %v , Got %v",
|
|
proto.MarshalTextString(expectedBlock), proto.MarshalTextString(block))
|
|
}
|
|
}
|