mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
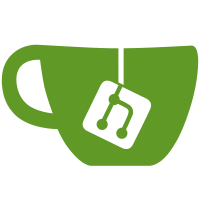
* remove v2 from all messages that are not request/responses * rename StreamBlocksAltair to StreamBlocks * fix tests * fix validator module (cherry picked from commit 7f2263e90e80499a851c2e09605e2ef5dccb0df8) # Conflicts: # testing/mock/beacon_validator_client_mock.go # testing/mock/beacon_validator_server_mock.go * fix mocks * update validator.pb.go * Revert "rename StreamBlocksAltair to StreamBlocks" This reverts commit 9c961c4e643e1c4d292f07acadab3c981e760fe4. # Conflicts: # proto/prysm/v1alpha1/validator.pb.go # testing/mock/beacon_validator_client_mock.go # testing/mock/beacon_validator_server_mock.go * Revert "Auxiliary commit to revert individual files from a872c9d59dee869da5a9c7236c7ac34fcaf8d54c" This reverts commit 89f19e4f15006c4a0efe593229abc433491e578e. * Revert "Auxiliary commit to revert individual files from 9acbf7b0160626dae0d39c58fa0d8a3e48c203e0" This reverts commit 398ecc9cef460c27e7fc92efe4df3fc9d3dbe566. * update mocks * fix build errors * patch up * fix Co-authored-by: Raul Jordan <raul@prysmaticlabs.com> Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com>
185 lines
6.2 KiB
Go
185 lines
6.2 KiB
Go
package debug
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/prysmaticlabs/prysm/v3/beacon-chain/rpc/eth/helpers"
|
|
ethpbv1 "github.com/prysmaticlabs/prysm/v3/proto/eth/v1"
|
|
ethpbv2 "github.com/prysmaticlabs/prysm/v3/proto/eth/v2"
|
|
"github.com/prysmaticlabs/prysm/v3/proto/migration"
|
|
"github.com/prysmaticlabs/prysm/v3/runtime/version"
|
|
"go.opencensus.io/trace"
|
|
"google.golang.org/grpc/codes"
|
|
"google.golang.org/grpc/status"
|
|
"google.golang.org/protobuf/types/known/emptypb"
|
|
)
|
|
|
|
// GetBeaconState returns the full beacon state for a given state ID.
|
|
func (ds *Server) GetBeaconState(ctx context.Context, req *ethpbv1.StateRequest) (*ethpbv1.BeaconStateResponse, error) {
|
|
ctx, span := trace.StartSpan(ctx, "debug.GetBeaconState")
|
|
defer span.End()
|
|
|
|
beaconSt, err := ds.StateFetcher.State(ctx, req.StateId)
|
|
if err != nil {
|
|
return nil, helpers.PrepareStateFetchGRPCError(err)
|
|
}
|
|
|
|
if beaconSt.Version() != version.Phase0 {
|
|
return nil, status.Error(codes.Internal, "State has incorrect type")
|
|
}
|
|
protoSt, err := migration.BeaconStateToProto(beaconSt)
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not convert state to proto: %v", err)
|
|
}
|
|
|
|
return ðpbv1.BeaconStateResponse{
|
|
Data: protoSt,
|
|
}, nil
|
|
}
|
|
|
|
// GetBeaconStateSSZ returns the SSZ-serialized version of the full beacon state object for given state ID.
|
|
func (ds *Server) GetBeaconStateSSZ(ctx context.Context, req *ethpbv1.StateRequest) (*ethpbv2.SSZContainer, error) {
|
|
ctx, span := trace.StartSpan(ctx, "debug.GetBeaconStateSSZ")
|
|
defer span.End()
|
|
|
|
state, err := ds.StateFetcher.State(ctx, req.StateId)
|
|
if err != nil {
|
|
return nil, helpers.PrepareStateFetchGRPCError(err)
|
|
}
|
|
|
|
sszState, err := state.MarshalSSZ()
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not marshal state into SSZ: %v", err)
|
|
}
|
|
|
|
return ðpbv2.SSZContainer{Data: sszState}, nil
|
|
}
|
|
|
|
// GetBeaconStateV2 returns the full beacon state for a given state ID.
|
|
func (ds *Server) GetBeaconStateV2(ctx context.Context, req *ethpbv2.BeaconStateRequestV2) (*ethpbv2.BeaconStateResponseV2, error) {
|
|
ctx, span := trace.StartSpan(ctx, "debug.GetBeaconStateV2")
|
|
defer span.End()
|
|
|
|
beaconSt, err := ds.StateFetcher.State(ctx, req.StateId)
|
|
if err != nil {
|
|
return nil, helpers.PrepareStateFetchGRPCError(err)
|
|
}
|
|
isOptimistic, err := helpers.IsOptimistic(ctx, beaconSt, ds.OptimisticModeFetcher)
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not check if slot's block is optimistic: %v", err)
|
|
}
|
|
|
|
switch beaconSt.Version() {
|
|
case version.Phase0:
|
|
protoSt, err := migration.BeaconStateToProto(beaconSt)
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not convert state to proto: %v", err)
|
|
}
|
|
return ðpbv2.BeaconStateResponseV2{
|
|
Version: ethpbv2.Version_PHASE0,
|
|
Data: ðpbv2.BeaconStateContainer{
|
|
State: ðpbv2.BeaconStateContainer_Phase0State{Phase0State: protoSt},
|
|
},
|
|
ExecutionOptimistic: isOptimistic,
|
|
}, nil
|
|
case version.Altair:
|
|
protoState, err := migration.BeaconStateAltairToProto(beaconSt)
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not convert state to proto: %v", err)
|
|
}
|
|
return ðpbv2.BeaconStateResponseV2{
|
|
Version: ethpbv2.Version_ALTAIR,
|
|
Data: ðpbv2.BeaconStateContainer{
|
|
State: ðpbv2.BeaconStateContainer_AltairState{AltairState: protoState},
|
|
},
|
|
ExecutionOptimistic: isOptimistic,
|
|
}, nil
|
|
case version.Bellatrix:
|
|
protoState, err := migration.BeaconStateBellatrixToProto(beaconSt)
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not convert state to proto: %v", err)
|
|
}
|
|
return ðpbv2.BeaconStateResponseV2{
|
|
Version: ethpbv2.Version_BELLATRIX,
|
|
Data: ðpbv2.BeaconStateContainer{
|
|
State: ðpbv2.BeaconStateContainer_BellatrixState{BellatrixState: protoState},
|
|
},
|
|
ExecutionOptimistic: isOptimistic,
|
|
}, nil
|
|
default:
|
|
return nil, status.Error(codes.Internal, "Unsupported state version")
|
|
}
|
|
}
|
|
|
|
// GetBeaconStateSSZV2 returns the SSZ-serialized version of the full beacon state object for given state ID.
|
|
func (ds *Server) GetBeaconStateSSZV2(ctx context.Context, req *ethpbv2.BeaconStateRequestV2) (*ethpbv2.SSZContainer, error) {
|
|
ctx, span := trace.StartSpan(ctx, "debug.GetBeaconStateSSZV2")
|
|
defer span.End()
|
|
|
|
st, err := ds.StateFetcher.State(ctx, req.StateId)
|
|
if err != nil {
|
|
return nil, helpers.PrepareStateFetchGRPCError(err)
|
|
}
|
|
|
|
sszState, err := st.MarshalSSZ()
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not marshal state into SSZ: %v", err)
|
|
}
|
|
var ver ethpbv2.Version
|
|
switch st.Version() {
|
|
case version.Phase0:
|
|
ver = ethpbv2.Version_PHASE0
|
|
case version.Altair:
|
|
ver = ethpbv2.Version_ALTAIR
|
|
case version.Bellatrix:
|
|
ver = ethpbv2.Version_BELLATRIX
|
|
default:
|
|
return nil, status.Error(codes.Internal, "Unsupported state version")
|
|
}
|
|
|
|
return ðpbv2.SSZContainer{Data: sszState, Version: ver}, nil
|
|
}
|
|
|
|
// ListForkChoiceHeads retrieves the leaves of the current fork choice tree.
|
|
func (ds *Server) ListForkChoiceHeads(ctx context.Context, _ *emptypb.Empty) (*ethpbv1.ForkChoiceHeadsResponse, error) {
|
|
ctx, span := trace.StartSpan(ctx, "debug.ListForkChoiceHeads")
|
|
defer span.End()
|
|
|
|
headRoots, headSlots := ds.HeadFetcher.ChainHeads()
|
|
resp := ðpbv1.ForkChoiceHeadsResponse{
|
|
Data: make([]*ethpbv1.ForkChoiceHead, len(headRoots)),
|
|
}
|
|
for i := range headRoots {
|
|
resp.Data[i] = ðpbv1.ForkChoiceHead{
|
|
Root: headRoots[i][:],
|
|
Slot: headSlots[i],
|
|
}
|
|
}
|
|
|
|
return resp, nil
|
|
}
|
|
|
|
// ListForkChoiceHeadsV2 retrieves the leaves of the current fork choice tree.
|
|
func (ds *Server) ListForkChoiceHeadsV2(ctx context.Context, _ *emptypb.Empty) (*ethpbv2.ForkChoiceHeadsResponse, error) {
|
|
ctx, span := trace.StartSpan(ctx, "debug.ListForkChoiceHeadsV2")
|
|
defer span.End()
|
|
|
|
headRoots, headSlots := ds.HeadFetcher.ChainHeads()
|
|
resp := ðpbv2.ForkChoiceHeadsResponse{
|
|
Data: make([]*ethpbv2.ForkChoiceHead, len(headRoots)),
|
|
}
|
|
for i := range headRoots {
|
|
isOptimistic, err := ds.OptimisticModeFetcher.IsOptimisticForRoot(ctx, headRoots[i])
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not check if head is optimistic: %v", err)
|
|
}
|
|
resp.Data[i] = ðpbv2.ForkChoiceHead{
|
|
Root: headRoots[i][:],
|
|
Slot: headSlots[i],
|
|
ExecutionOptimistic: isOptimistic,
|
|
}
|
|
}
|
|
|
|
return resp, nil
|
|
}
|