mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
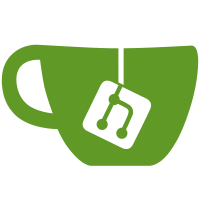
* checkpoint * checkpoint * varint prefix for ssz * move the encoding API around a little bit to support reader writer * add a simple test for the happy path subscribe * move wait timeout to testutil * Add inverted topic mapping * Add varint prefixing to ssz network encoder * fix spacing * fix comments * fix comments * make anon methods more clear * clean up log fields * move topic mapping, reformat TODOs, get ready for brutal team review * lint * lint * lint * Update beacon-chain/p2p/gossip_topic_mappings.go Co-Authored-By: Nishant Das <nishdas93@gmail.com> * PR feedback * PR feedback * PR feedback * PR feedback * PR feedback * basic test with a hardcoded fork choice * updated beacon config to use genesis fork version * checkpoint on hello handler * create a testing db method that can be used with the new database interface * lint * lint * PR feedback * checkpoint * passing tests * comments, errors * comments, errors * remove swarm * Add basic sanity test, naive implementation * add test with real data
66 lines
1.9 KiB
Go
66 lines
1.9 KiB
Go
package sync
|
|
|
|
import (
|
|
"context"
|
|
"time"
|
|
|
|
"github.com/gogo/protobuf/proto"
|
|
libp2pcore "github.com/libp2p/go-libp2p-core"
|
|
"github.com/pkg/errors"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/db/filters"
|
|
pb "github.com/prysmaticlabs/prysm/proto/beacon/p2p/v1"
|
|
)
|
|
|
|
// beaconBlocksRPCHandler looks up the request blocks from the database from a given start block.
|
|
func (r *RegularSync) beaconBlocksRPCHandler(ctx context.Context, msg proto.Message, stream libp2pcore.Stream) error {
|
|
defer stream.Close()
|
|
ctx, cancel := context.WithTimeout(ctx, 5*time.Second)
|
|
defer cancel()
|
|
setRPCStreamDeadlines(stream)
|
|
|
|
m := msg.(*pb.BeaconBlocksRequest)
|
|
|
|
startSlot := m.HeadSlot
|
|
endSlot := startSlot + (m.Step * m.Count)
|
|
|
|
// TODO(3147): Update this with reasonable constraints.
|
|
if endSlot-startSlot > 1000 || m.Step == 0 {
|
|
resp, err := r.generateErrorResponse(responseCodeInvalidRequest, "invalid range or step")
|
|
if err != nil {
|
|
log.WithError(err).Error("Failed to generate a response error")
|
|
} else {
|
|
if _, err := stream.Write(resp); err != nil {
|
|
log.WithError(err).Errorf("Failed to write to stream")
|
|
}
|
|
}
|
|
return errors.New("invalid range or step")
|
|
}
|
|
|
|
// TODO(3147): Only return canonical blocks.
|
|
blks, err := r.db.Blocks(ctx, filters.NewFilter().SetStartSlot(startSlot).SetEndSlot(endSlot))
|
|
if err != nil {
|
|
resp, err := r.generateErrorResponse(responseCodeServerError, genericError)
|
|
if err != nil {
|
|
log.WithError(err).Error("Failed to generate a response error")
|
|
} else {
|
|
if _, err := stream.Write(resp); err != nil {
|
|
log.WithError(err).Errorf("Failed to write to stream")
|
|
}
|
|
}
|
|
return err
|
|
}
|
|
ret := &pb.BeaconBlocksResponse{}
|
|
|
|
for _, blk := range blks {
|
|
if (blk.Slot-startSlot)%m.Step == 0 {
|
|
ret.Blocks = append(ret.Blocks, blk)
|
|
}
|
|
}
|
|
|
|
if _, err := stream.Write([]byte{responseCodeSuccess}); err != nil {
|
|
log.WithError(err).Error("Failed to write to stream")
|
|
}
|
|
_, err = r.p2p.Encoding().Encode(stream, ret)
|
|
return err
|
|
}
|