mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
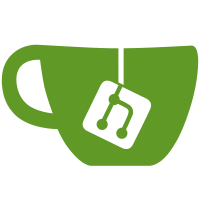
* Merge variable declaration and assignment * Use result of type assertion to simplify cases * Replace call to bytes.Compare with bytes.Equal * Drop unnecessary use of the blank identifier * Replace x.Sub(time.Now()) with time.Until(x) * Function literal can be simplified * Use a single append to concatenate two slices * Replace time.Now().Sub(x) with time.Since(x) * Omit comparison with boolean constant * Omit redundant nil check on slices * Nested if can be replaced with else-if * Function call can be replaced with helper function * Omit redundant control flow * Use plain channel send or receive * Simplify returning boolean expression * Merge branch 'origin-master' into fix-antipatterns * Merge branch 'master' into fix-antipatterns
67 lines
1.8 KiB
Go
67 lines
1.8 KiB
Go
package attestations
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
"github.com/prysmaticlabs/prysm/shared/roughtime"
|
|
)
|
|
|
|
// pruneAttsPool prunes attestations pool on every slot interval.
|
|
func (s *Service) pruneAttsPool() {
|
|
ticker := time.NewTicker(s.pruneInterval)
|
|
for {
|
|
select {
|
|
case <-ticker.C:
|
|
s.pruneExpiredAtts()
|
|
s.updateMetrics()
|
|
case <-s.ctx.Done():
|
|
log.Debug("Context closed, exiting routine")
|
|
ticker.Stop()
|
|
return
|
|
}
|
|
}
|
|
}
|
|
|
|
// This prunes expired attestations from the pool.
|
|
func (s *Service) pruneExpiredAtts() {
|
|
aggregatedAtts := s.pool.AggregatedAttestations()
|
|
for _, att := range aggregatedAtts {
|
|
if s.expired(att.Data.Slot) {
|
|
if err := s.pool.DeleteAggregatedAttestation(att); err != nil {
|
|
log.WithError(err).Error("Could not delete expired aggregated attestation")
|
|
}
|
|
expiredAggregatedAtts.Inc()
|
|
}
|
|
}
|
|
|
|
unAggregatedAtts := s.pool.UnaggregatedAttestations()
|
|
for _, att := range unAggregatedAtts {
|
|
if s.expired(att.Data.Slot) {
|
|
if err := s.pool.DeleteUnaggregatedAttestation(att); err != nil {
|
|
log.WithError(err).Error("Could not delete expired unaggregated attestation")
|
|
}
|
|
expiredUnaggregatedAtts.Inc()
|
|
}
|
|
}
|
|
|
|
blockAtts := s.pool.BlockAttestations()
|
|
for _, att := range blockAtts {
|
|
if s.expired(att.Data.Slot) {
|
|
if err := s.pool.DeleteBlockAttestation(att); err != nil {
|
|
log.WithError(err).Error("Could not delete expired block attestation")
|
|
}
|
|
}
|
|
expiredBlockAtts.Inc()
|
|
}
|
|
}
|
|
|
|
// Return true if the input slot has been expired.
|
|
// Expired is defined as one epoch behind than current time.
|
|
func (s *Service) expired(slot uint64) bool {
|
|
expirationSlot := slot + params.BeaconConfig().SlotsPerEpoch
|
|
expirationTime := s.genesisTime + expirationSlot*params.BeaconConfig().SecondsPerSlot
|
|
currentTime := uint64(roughtime.Now().Unix())
|
|
return currentTime >= expirationTime
|
|
}
|