mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
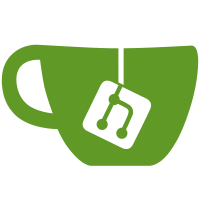
* Merge variable declaration and assignment * Use result of type assertion to simplify cases * Replace call to bytes.Compare with bytes.Equal * Drop unnecessary use of the blank identifier * Replace x.Sub(time.Now()) with time.Until(x) * Function literal can be simplified * Use a single append to concatenate two slices * Replace time.Now().Sub(x) with time.Since(x) * Omit comparison with boolean constant * Omit redundant nil check on slices * Nested if can be replaced with else-if * Function call can be replaced with helper function * Omit redundant control flow * Use plain channel send or receive * Simplify returning boolean expression * Merge branch 'origin-master' into fix-antipatterns * Merge branch 'master' into fix-antipatterns
51 lines
1.5 KiB
Go
51 lines
1.5 KiB
Go
package grpcutils
|
|
|
|
import (
|
|
"context"
|
|
"time"
|
|
|
|
"github.com/sirupsen/logrus"
|
|
"google.golang.org/grpc"
|
|
"google.golang.org/grpc/metadata"
|
|
)
|
|
|
|
// LogGRPCRequests this method logs the gRPC backend as well as request duration when the log level is set to debug
|
|
// or higher.
|
|
func LogGRPCRequests(ctx context.Context, method string, req, reply interface{}, cc *grpc.ClientConn, invoker grpc.UnaryInvoker, opts ...grpc.CallOption) error {
|
|
// Shortcut when debug logging is not enabled.
|
|
if logrus.GetLevel() < logrus.DebugLevel {
|
|
return invoker(ctx, method, req, reply, cc, opts...)
|
|
}
|
|
|
|
var header metadata.MD
|
|
opts = append(
|
|
opts,
|
|
grpc.Header(&header),
|
|
)
|
|
start := time.Now()
|
|
err := invoker(ctx, method, req, reply, cc, opts...)
|
|
logrus.WithField("backend", header["x-backend"]).
|
|
WithField("method", method).WithField("duration", time.Since(start)).
|
|
Debug("gRPC request finished.")
|
|
return err
|
|
}
|
|
|
|
// LogGRPCStream to print the method at DEBUG level at the start of the stream.
|
|
func LogGRPCStream(ctx context.Context, sd *grpc.StreamDesc, conn *grpc.ClientConn, method string, streamer grpc.Streamer, opts ...grpc.CallOption) (grpc.ClientStream, error) {
|
|
// Shortcut when debug logging is not enabled.
|
|
if logrus.GetLevel() < logrus.DebugLevel {
|
|
return streamer(ctx, sd, conn, method, opts...)
|
|
}
|
|
|
|
var header metadata.MD
|
|
opts = append(
|
|
opts,
|
|
grpc.Header(&header),
|
|
)
|
|
strm, err := streamer(ctx, sd, conn, method, opts...)
|
|
logrus.WithField("backend", header["x-backend"]).
|
|
WithField("method", method).
|
|
Debug("gRPC stream started.")
|
|
return strm, err
|
|
}
|