mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
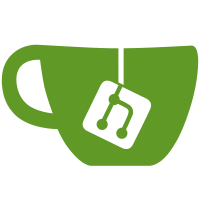
* Fix a few deps to work with go.mod, check in generated files * Update Gossipsub to 1.1 (#5998) * update libs * add new validators * add new deps * new set of deps * tls * further fix gossip update * get everything to build * clean up * gaz * fix build * fix all tests * add deps to images * imports Co-authored-by: rauljordan <raul@prysmaticlabs.com> * Beacon chain builds with go build * fix bazel * fix dep * lint * Add github action for testing go * on PR for any branch * fix libp2p test failure * Fix TestProcessBlock_PassesProcessingConditions by updating the proposer index in test * Revert "Fix TestProcessBlock_PassesProcessingConditions by updating the proposer index in test" This reverts commit 43676894ab01f03fe90a9b8ee3ecfbc2ec1ec4e4. * Compute and set proposer index instead of hard code * Add back go mod/sum, fix deps * go build ./... * Temporarily skip two tests * Fix kafka confluent patch * Fix kafka confluent patch * fix kafka build * fix kafka * Add info in DEPENDENCIES. Added a stub link for Why Bazel? until https://github.com/prysmaticlabs/documentation/issues/138 * Update fuzz ssz files as well * Update fuzz ssz files as well * getting closer * rollback rules_go and gazelle * fix gogo protobuf * install librdkafka-dev as part of github actions * Update kafka to a recent version where librkafkfa is not required for go modules * clarify comment * fix kafka build * disable go tests * comment * Fix geth dependencies for end to end * rename word * lint * fix docker Co-authored-by: Nishant Das <nishdas93@gmail.com> Co-authored-by: rauljordan <raul@prysmaticlabs.com> Co-authored-by: terence tsao <terence@prysmaticlabs.com>
399 lines
9.4 KiB
Go
Executable File
399 lines
9.4 KiB
Go
Executable File
// Code generated by fastssz. DO NOT EDIT.
|
|
package fuzz
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
ssz "github.com/ferranbt/fastssz"
|
|
ethpb "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
)
|
|
|
|
var (
|
|
errDivideInt = fmt.Errorf("incorrect int divide")
|
|
errListTooBig = fmt.Errorf("incorrect list size, too big")
|
|
errMarshalDynamicBytes = fmt.Errorf("incorrect dynamic bytes marshalling")
|
|
errMarshalFixedBytes = fmt.Errorf("incorrect fixed bytes marshalling")
|
|
errMarshalList = fmt.Errorf("incorrect vector list")
|
|
errMarshalVector = fmt.Errorf("incorrect vector marshalling")
|
|
errOffset = fmt.Errorf("incorrect offset")
|
|
errSize = fmt.Errorf("incorrect size")
|
|
)
|
|
|
|
// MarshalSSZ ssz marshals the InputBlockHeader object
|
|
func (i *InputBlockHeader) MarshalSSZ() ([]byte, error) {
|
|
buf := make([]byte, i.SizeSSZ())
|
|
return i.MarshalSSZTo(buf[:0])
|
|
}
|
|
|
|
// MarshalSSZTo ssz marshals the InputBlockHeader object to a target array
|
|
func (i *InputBlockHeader) MarshalSSZTo(dst []byte) ([]byte, error) {
|
|
var err error
|
|
offset := int(6)
|
|
|
|
// Field (0) 'StateID'
|
|
dst = ssz.MarshalUint16(dst, i.StateID)
|
|
|
|
// Offset (1) 'Block'
|
|
dst = ssz.WriteOffset(dst, offset)
|
|
if i.Block == nil {
|
|
i.Block = new(ethpb.BeaconBlock)
|
|
}
|
|
offset += i.Block.SizeSSZ()
|
|
|
|
// Field (1) 'Block'
|
|
if dst, err = i.Block.MarshalSSZTo(dst); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return dst, err
|
|
}
|
|
|
|
// UnmarshalSSZ ssz unmarshals the InputBlockHeader object
|
|
func (i *InputBlockHeader) UnmarshalSSZ(buf []byte) error {
|
|
var err error
|
|
size := uint64(len(buf))
|
|
if size < 6 {
|
|
return errSize
|
|
}
|
|
|
|
tail := buf
|
|
var o1 uint64
|
|
|
|
// Field (0) 'StateID'
|
|
i.StateID = ssz.UnmarshallUint16(buf[0:2])
|
|
|
|
// Offset (1) 'Block'
|
|
if o1 = ssz.ReadOffset(buf[2:6]); o1 > size {
|
|
return errOffset
|
|
}
|
|
|
|
// Field (1) 'Block'
|
|
{
|
|
buf = tail[o1:]
|
|
if i.Block == nil {
|
|
i.Block = new(ethpb.BeaconBlock)
|
|
}
|
|
if err = i.Block.UnmarshalSSZ(buf); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
return err
|
|
}
|
|
|
|
// SizeSSZ returns the ssz encoded size in bytes for the InputBlockHeader object
|
|
func (i *InputBlockHeader) SizeSSZ() (size int) {
|
|
size = 6
|
|
|
|
// Field (1) 'Block'
|
|
if i.Block == nil {
|
|
i.Block = new(ethpb.BeaconBlock)
|
|
}
|
|
size += i.Block.SizeSSZ()
|
|
|
|
return
|
|
}
|
|
|
|
// MarshalSSZ ssz marshals the InputAttesterSlashingWrapper object
|
|
func (i *InputAttesterSlashingWrapper) MarshalSSZ() ([]byte, error) {
|
|
buf := make([]byte, i.SizeSSZ())
|
|
return i.MarshalSSZTo(buf[:0])
|
|
}
|
|
|
|
// MarshalSSZTo ssz marshals the InputAttesterSlashingWrapper object to a target array
|
|
func (i *InputAttesterSlashingWrapper) MarshalSSZTo(dst []byte) ([]byte, error) {
|
|
var err error
|
|
offset := int(6)
|
|
|
|
// Field (0) 'StateID'
|
|
dst = ssz.MarshalUint16(dst, i.StateID)
|
|
|
|
// Offset (1) 'AttesterSlashing'
|
|
dst = ssz.WriteOffset(dst, offset)
|
|
if i.AttesterSlashing == nil {
|
|
i.AttesterSlashing = new(ethpb.AttesterSlashing)
|
|
}
|
|
offset += i.AttesterSlashing.SizeSSZ()
|
|
|
|
// Field (1) 'AttesterSlashing'
|
|
if dst, err = i.AttesterSlashing.MarshalSSZTo(dst); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return dst, err
|
|
}
|
|
|
|
// UnmarshalSSZ ssz unmarshals the InputAttesterSlashingWrapper object
|
|
func (i *InputAttesterSlashingWrapper) UnmarshalSSZ(buf []byte) error {
|
|
var err error
|
|
size := uint64(len(buf))
|
|
if size < 6 {
|
|
return errSize
|
|
}
|
|
|
|
tail := buf
|
|
var o1 uint64
|
|
|
|
// Field (0) 'StateID'
|
|
i.StateID = ssz.UnmarshallUint16(buf[0:2])
|
|
|
|
// Offset (1) 'AttesterSlashing'
|
|
if o1 = ssz.ReadOffset(buf[2:6]); o1 > size {
|
|
return errOffset
|
|
}
|
|
|
|
// Field (1) 'AttesterSlashing'
|
|
{
|
|
buf = tail[o1:]
|
|
if i.AttesterSlashing == nil {
|
|
i.AttesterSlashing = new(ethpb.AttesterSlashing)
|
|
}
|
|
if err = i.AttesterSlashing.UnmarshalSSZ(buf); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
return err
|
|
}
|
|
|
|
// SizeSSZ returns the ssz encoded size in bytes for the InputAttesterSlashingWrapper object
|
|
func (i *InputAttesterSlashingWrapper) SizeSSZ() (size int) {
|
|
size = 6
|
|
|
|
// Field (1) 'AttesterSlashing'
|
|
if i.AttesterSlashing == nil {
|
|
i.AttesterSlashing = new(ethpb.AttesterSlashing)
|
|
}
|
|
size += i.AttesterSlashing.SizeSSZ()
|
|
|
|
return
|
|
}
|
|
|
|
// MarshalSSZ ssz marshals the InputAttestationWrapper object
|
|
func (i *InputAttestationWrapper) MarshalSSZ() ([]byte, error) {
|
|
buf := make([]byte, i.SizeSSZ())
|
|
return i.MarshalSSZTo(buf[:0])
|
|
}
|
|
|
|
// MarshalSSZTo ssz marshals the InputAttestationWrapper object to a target array
|
|
func (i *InputAttestationWrapper) MarshalSSZTo(dst []byte) ([]byte, error) {
|
|
var err error
|
|
offset := int(6)
|
|
|
|
// Field (0) 'StateID'
|
|
dst = ssz.MarshalUint16(dst, i.StateID)
|
|
|
|
// Offset (1) 'Attestation'
|
|
dst = ssz.WriteOffset(dst, offset)
|
|
if i.Attestation == nil {
|
|
i.Attestation = new(ethpb.Attestation)
|
|
}
|
|
offset += i.Attestation.SizeSSZ()
|
|
|
|
// Field (1) 'Attestation'
|
|
if dst, err = i.Attestation.MarshalSSZTo(dst); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return dst, err
|
|
}
|
|
|
|
// UnmarshalSSZ ssz unmarshals the InputAttestationWrapper object
|
|
func (i *InputAttestationWrapper) UnmarshalSSZ(buf []byte) error {
|
|
var err error
|
|
size := uint64(len(buf))
|
|
if size < 6 {
|
|
return errSize
|
|
}
|
|
|
|
tail := buf
|
|
var o1 uint64
|
|
|
|
// Field (0) 'StateID'
|
|
i.StateID = ssz.UnmarshallUint16(buf[0:2])
|
|
|
|
// Offset (1) 'Attestation'
|
|
if o1 = ssz.ReadOffset(buf[2:6]); o1 > size {
|
|
return errOffset
|
|
}
|
|
|
|
// Field (1) 'Attestation'
|
|
{
|
|
buf = tail[o1:]
|
|
if i.Attestation == nil {
|
|
i.Attestation = new(ethpb.Attestation)
|
|
}
|
|
if err = i.Attestation.UnmarshalSSZ(buf); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
return err
|
|
}
|
|
|
|
// SizeSSZ returns the ssz encoded size in bytes for the InputAttestationWrapper object
|
|
func (i *InputAttestationWrapper) SizeSSZ() (size int) {
|
|
size = 6
|
|
|
|
// Field (1) 'Attestation'
|
|
if i.Attestation == nil {
|
|
i.Attestation = new(ethpb.Attestation)
|
|
}
|
|
size += i.Attestation.SizeSSZ()
|
|
|
|
return
|
|
}
|
|
|
|
// MarshalSSZ ssz marshals the InputDepositWrapper object
|
|
func (i *InputDepositWrapper) MarshalSSZ() ([]byte, error) {
|
|
buf := make([]byte, i.SizeSSZ())
|
|
return i.MarshalSSZTo(buf[:0])
|
|
}
|
|
|
|
// MarshalSSZTo ssz marshals the InputDepositWrapper object to a target array
|
|
func (i *InputDepositWrapper) MarshalSSZTo(dst []byte) ([]byte, error) {
|
|
var err error
|
|
|
|
// Field (0) 'StateID'
|
|
dst = ssz.MarshalUint16(dst, i.StateID)
|
|
|
|
// Field (1) 'Deposit'
|
|
if i.Deposit == nil {
|
|
i.Deposit = new(ethpb.Deposit)
|
|
}
|
|
if dst, err = i.Deposit.MarshalSSZTo(dst); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return dst, err
|
|
}
|
|
|
|
// UnmarshalSSZ ssz unmarshals the InputDepositWrapper object
|
|
func (i *InputDepositWrapper) UnmarshalSSZ(buf []byte) error {
|
|
var err error
|
|
size := uint64(len(buf))
|
|
if size != 1242 {
|
|
return errSize
|
|
}
|
|
|
|
// Field (0) 'StateID'
|
|
i.StateID = ssz.UnmarshallUint16(buf[0:2])
|
|
|
|
// Field (1) 'Deposit'
|
|
if i.Deposit == nil {
|
|
i.Deposit = new(ethpb.Deposit)
|
|
}
|
|
if err = i.Deposit.UnmarshalSSZ(buf[2:1242]); err != nil {
|
|
return err
|
|
}
|
|
|
|
return err
|
|
}
|
|
|
|
// SizeSSZ returns the ssz encoded size in bytes for the InputDepositWrapper object
|
|
func (i *InputDepositWrapper) SizeSSZ() (size int) {
|
|
size = 1242
|
|
return
|
|
}
|
|
|
|
// MarshalSSZ ssz marshals the InputVoluntaryExitWrapper object
|
|
func (i *InputVoluntaryExitWrapper) MarshalSSZ() ([]byte, error) {
|
|
buf := make([]byte, i.SizeSSZ())
|
|
return i.MarshalSSZTo(buf[:0])
|
|
}
|
|
|
|
// MarshalSSZTo ssz marshals the InputVoluntaryExitWrapper object to a target array
|
|
func (i *InputVoluntaryExitWrapper) MarshalSSZTo(dst []byte) ([]byte, error) {
|
|
var err error
|
|
|
|
// Field (0) 'StateID'
|
|
dst = ssz.MarshalUint16(dst, i.StateID)
|
|
|
|
// Field (1) 'VoluntaryExit'
|
|
if i.VoluntaryExit == nil {
|
|
i.VoluntaryExit = new(ethpb.VoluntaryExit)
|
|
}
|
|
if dst, err = i.VoluntaryExit.MarshalSSZTo(dst); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return dst, err
|
|
}
|
|
|
|
// UnmarshalSSZ ssz unmarshals the InputVoluntaryExitWrapper object
|
|
func (i *InputVoluntaryExitWrapper) UnmarshalSSZ(buf []byte) error {
|
|
var err error
|
|
size := uint64(len(buf))
|
|
if size != 18 {
|
|
return errSize
|
|
}
|
|
|
|
// Field (0) 'StateID'
|
|
i.StateID = ssz.UnmarshallUint16(buf[0:2])
|
|
|
|
// Field (1) 'VoluntaryExit'
|
|
if i.VoluntaryExit == nil {
|
|
i.VoluntaryExit = new(ethpb.VoluntaryExit)
|
|
}
|
|
if err = i.VoluntaryExit.UnmarshalSSZ(buf[2:18]); err != nil {
|
|
return err
|
|
}
|
|
|
|
return err
|
|
}
|
|
|
|
// SizeSSZ returns the ssz encoded size in bytes for the InputVoluntaryExitWrapper object
|
|
func (i *InputVoluntaryExitWrapper) SizeSSZ() (size int) {
|
|
size = 18
|
|
return
|
|
}
|
|
|
|
// MarshalSSZ ssz marshals the InputProposerSlashingWrapper object
|
|
func (i *InputProposerSlashingWrapper) MarshalSSZ() ([]byte, error) {
|
|
buf := make([]byte, i.SizeSSZ())
|
|
return i.MarshalSSZTo(buf[:0])
|
|
}
|
|
|
|
// MarshalSSZTo ssz marshals the InputProposerSlashingWrapper object to a target array
|
|
func (i *InputProposerSlashingWrapper) MarshalSSZTo(dst []byte) ([]byte, error) {
|
|
var err error
|
|
|
|
// Field (0) 'StateID'
|
|
dst = ssz.MarshalUint16(dst, i.StateID)
|
|
|
|
// Field (1) 'ProposerSlashing'
|
|
if i.ProposerSlashing == nil {
|
|
i.ProposerSlashing = new(ethpb.ProposerSlashing)
|
|
}
|
|
if dst, err = i.ProposerSlashing.MarshalSSZTo(dst); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return dst, err
|
|
}
|
|
|
|
// UnmarshalSSZ ssz unmarshals the InputProposerSlashingWrapper object
|
|
func (i *InputProposerSlashingWrapper) UnmarshalSSZ(buf []byte) error {
|
|
var err error
|
|
size := uint64(len(buf))
|
|
if size != 418 {
|
|
return errSize
|
|
}
|
|
|
|
// Field (0) 'StateID'
|
|
i.StateID = ssz.UnmarshallUint16(buf[0:2])
|
|
|
|
// Field (1) 'ProposerSlashing'
|
|
if i.ProposerSlashing == nil {
|
|
i.ProposerSlashing = new(ethpb.ProposerSlashing)
|
|
}
|
|
if err = i.ProposerSlashing.UnmarshalSSZ(buf[2:418]); err != nil {
|
|
return err
|
|
}
|
|
|
|
return err
|
|
}
|
|
|
|
// SizeSSZ returns the ssz encoded size in bytes for the InputProposerSlashingWrapper object
|
|
func (i *InputProposerSlashingWrapper) SizeSSZ() (size int) {
|
|
size = 418
|
|
return
|
|
}
|