mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
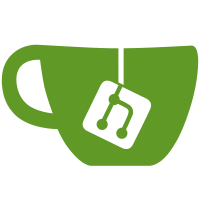
* v3 import renamings * tidy * fmt * rev * Update beacon-chain/core/epoch/precompute/reward_penalty_test.go * Update beacon-chain/core/helpers/validators_test.go * Update beacon-chain/db/alias.go * Update beacon-chain/db/alias.go * Update beacon-chain/db/alias.go * Update beacon-chain/db/iface/BUILD.bazel * Update beacon-chain/db/kv/kv.go * Update beacon-chain/db/kv/state.go * Update beacon-chain/rpc/prysm/v1alpha1/validator/attester_test.go * Update beacon-chain/rpc/prysm/v1alpha1/validator/attester_test.go * Update beacon-chain/sync/initial-sync/service.go * fix deps * fix bad replacements * fix bad replacements * change back * gohashtree version * fix deps Co-authored-by: Nishant Das <nishdas93@gmail.com> Co-authored-by: Potuz <potuz@prysmaticlabs.com>
120 lines
2.9 KiB
Go
120 lines
2.9 KiB
Go
// Package slasherkv defines a bolt-db, key-value store implementation
|
|
// of the slasher database interface for Prysm.
|
|
package slasherkv
|
|
|
|
import (
|
|
"context"
|
|
"os"
|
|
"path"
|
|
"time"
|
|
|
|
"github.com/pkg/errors"
|
|
"github.com/prysmaticlabs/prysm/v3/beacon-chain/db/iface"
|
|
"github.com/prysmaticlabs/prysm/v3/config/params"
|
|
"github.com/prysmaticlabs/prysm/v3/io/file"
|
|
bolt "go.etcd.io/bbolt"
|
|
)
|
|
|
|
var _ iface.SlasherDatabase = (*Store)(nil)
|
|
|
|
const (
|
|
// DatabaseFileName is the name of the beacon node database.
|
|
DatabaseFileName = "slasher.db"
|
|
boltAllocSize = 8 * 1024 * 1024
|
|
)
|
|
|
|
// Config for the bolt db kv store.
|
|
type Config struct {
|
|
InitialMMapSize int
|
|
}
|
|
|
|
// Store defines an implementation of the Prysm Database interface
|
|
// using BoltDB as the underlying persistent kv-store for Ethereum consensus.
|
|
type Store struct {
|
|
db *bolt.DB
|
|
databasePath string
|
|
ctx context.Context
|
|
}
|
|
|
|
// NewKVStore initializes a new boltDB key-value store at the directory
|
|
// path specified, creates the kv-buckets based on the schema, and stores
|
|
// an open connection db object as a property of the Store struct.
|
|
func NewKVStore(ctx context.Context, dirPath string, config *Config) (*Store, error) {
|
|
hasDir, err := file.HasDir(dirPath)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
if !hasDir {
|
|
if err := file.MkdirAll(dirPath); err != nil {
|
|
return nil, err
|
|
}
|
|
}
|
|
datafile := path.Join(dirPath, DatabaseFileName)
|
|
boltDB, err := bolt.Open(
|
|
datafile,
|
|
params.BeaconIoConfig().ReadWritePermissions,
|
|
&bolt.Options{
|
|
Timeout: 1 * time.Second,
|
|
InitialMmapSize: config.InitialMMapSize,
|
|
},
|
|
)
|
|
if err != nil {
|
|
if errors.Is(err, bolt.ErrTimeout) {
|
|
return nil, errors.New("cannot obtain database lock, database may be in use by another process")
|
|
}
|
|
return nil, err
|
|
}
|
|
boltDB.AllocSize = boltAllocSize
|
|
kv := &Store{
|
|
db: boltDB,
|
|
databasePath: dirPath,
|
|
ctx: ctx,
|
|
}
|
|
|
|
if err := kv.db.Update(func(tx *bolt.Tx) error {
|
|
return createBuckets(
|
|
tx,
|
|
// Slasher buckets.
|
|
attestedEpochsByValidator,
|
|
attestationRecordsBucket,
|
|
attestationDataRootsBucket,
|
|
proposalRecordsBucket,
|
|
slasherChunksBucket,
|
|
)
|
|
}); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return kv, err
|
|
}
|
|
|
|
// ClearDB removes the previously stored database in the data directory.
|
|
func (s *Store) ClearDB() error {
|
|
if _, err := os.Stat(s.databasePath); os.IsNotExist(err) {
|
|
return nil
|
|
}
|
|
if err := os.Remove(path.Join(s.databasePath, DatabaseFileName)); err != nil {
|
|
return errors.Wrap(err, "could not remove database file")
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// Close closes the underlying BoltDB database.
|
|
func (s *Store) Close() error {
|
|
return s.db.Close()
|
|
}
|
|
|
|
// DatabasePath at which this database writes files.
|
|
func (s *Store) DatabasePath() string {
|
|
return s.databasePath
|
|
}
|
|
|
|
func createBuckets(tx *bolt.Tx, buckets ...[]byte) error {
|
|
for _, bucket := range buckets {
|
|
if _, err := tx.CreateBucketIfNotExists(bucket); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
return nil
|
|
}
|