mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
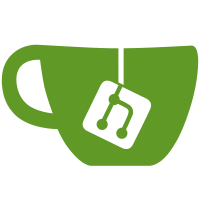
* Add database migrations, still need to update the API usage... * gofmt goimports * progress * Merge branch 'master' of github.com:prysmaticlabs/prysm into index-migration * use slot instead of index * rename LastArchivedIndex to LastArchivedSlot * rename LastArchivedIndexRoot to LastArchivedRoot * remove unused HighestSlotStates method * deprecate old key, include in migration * deprecate old key, include in migration * remove blocks index in migration * rename bucket variable * fix code to pass tests * Merge branch 'master' of github.com:prysmaticlabs/prysm into index-migration * gofmt, goimports * fix * Add state slot index * progress * lint * fix build * Merge branch 'master' of github.com:prysmaticlabs/prysm into index-migration * kafka * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * remove SaveArchivedPointRoot, a few other big changes * Merge branch 'index-migration' of github.com:prysmaticlabs/prysm into index-migration * fix tests and lint * lint again * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * block migration, some renaming * gaz, gofmt * add tests * change index to uint bytes * Merge branch 'index-migration' of github.com:prysmaticlabs/prysm into index-migration * rm method notes * stop if the bucket doesn't exist * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * @rauljordan pr feedback * Simplify * Merge refs/heads/master into index-migration * Remove unused method, add roundtrip test * gofmt * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge branch 'master' of github.com:prysmaticlabs/prysm into index-migration
75 lines
2.2 KiB
Go
75 lines
2.2 KiB
Go
package kv
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/prysmaticlabs/prysm/shared/bytesutil"
|
|
bolt "go.etcd.io/bbolt"
|
|
"go.opencensus.io/trace"
|
|
)
|
|
|
|
// LastArchivedSlot from the db.
|
|
func (kv *Store) LastArchivedSlot(ctx context.Context) (uint64, error) {
|
|
ctx, span := trace.StartSpan(ctx, "BeaconDB.LastArchivedSlot")
|
|
defer span.End()
|
|
var index uint64
|
|
err := kv.db.View(func(tx *bolt.Tx) error {
|
|
bkt := tx.Bucket(stateSlotIndicesBucket)
|
|
b, _ := bkt.Cursor().Last()
|
|
index = bytesutil.BytesToUint64BigEndian(b)
|
|
return nil
|
|
})
|
|
|
|
return index, err
|
|
}
|
|
|
|
// LastArchivedRoot from the db.
|
|
func (kv *Store) LastArchivedRoot(ctx context.Context) [32]byte {
|
|
ctx, span := trace.StartSpan(ctx, "BeaconDB.LastArchivedRoot")
|
|
defer span.End()
|
|
|
|
var blockRoot []byte
|
|
if err := kv.db.View(func(tx *bolt.Tx) error {
|
|
bkt := tx.Bucket(stateSlotIndicesBucket)
|
|
_, blockRoot = bkt.Cursor().Last()
|
|
return nil
|
|
}); err != nil { // This view never returns an error, but we'll handle anyway for sanity.
|
|
panic(err)
|
|
}
|
|
|
|
return bytesutil.ToBytes32(blockRoot)
|
|
}
|
|
|
|
// ArchivedPointRoot returns the block root of an archived point from the DB.
|
|
// This is essential for cold state management and to restore a cold state.
|
|
func (kv *Store) ArchivedPointRoot(ctx context.Context, slot uint64) [32]byte {
|
|
ctx, span := trace.StartSpan(ctx, "BeaconDB.ArchivedPointRoot")
|
|
defer span.End()
|
|
|
|
var blockRoot []byte
|
|
if err := kv.db.View(func(tx *bolt.Tx) error {
|
|
bucket := tx.Bucket(stateSlotIndicesBucket)
|
|
blockRoot = bucket.Get(bytesutil.Uint64ToBytesBigEndian(slot))
|
|
return nil
|
|
}); err != nil { // This view never returns an error, but we'll handle anyway for sanity.
|
|
panic(err)
|
|
}
|
|
|
|
return bytesutil.ToBytes32(blockRoot)
|
|
}
|
|
|
|
// HasArchivedPoint returns true if an archived point exists in DB.
|
|
func (kv *Store) HasArchivedPoint(ctx context.Context, slot uint64) bool {
|
|
ctx, span := trace.StartSpan(ctx, "BeaconDB.HasArchivedPoint")
|
|
defer span.End()
|
|
var exists bool
|
|
if err := kv.db.View(func(tx *bolt.Tx) error {
|
|
iBucket := tx.Bucket(stateSlotIndicesBucket)
|
|
exists = iBucket.Get(bytesutil.Uint64ToBytesBigEndian(slot)) != nil
|
|
return nil
|
|
}); err != nil { // This view never returns an error, but we'll handle anyway for sanity.
|
|
panic(err)
|
|
}
|
|
return exists
|
|
}
|