mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 13:18:57 +00:00
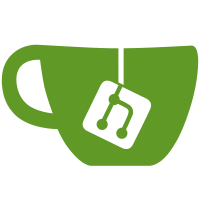
* Add sync subnet id cache * Update BUILD.bazel * Update BUILD.bazel * Update beacon-chain/cache/sync_subnet_ids.go Co-authored-by: Preston Van Loon <preston@prysmaticlabs.com> * Add comments * add more comments Co-authored-by: Raul Jordan <raul@prysmaticlabs.com> Co-authored-by: Preston Van Loon <preston@prysmaticlabs.com> Co-authored-by: nisdas <nishdas93@gmail.com>
58 lines
1.4 KiB
Go
58 lines
1.4 KiB
Go
package cache
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/assert"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/require"
|
|
)
|
|
|
|
func TestSyncSubnetIDsCache_Roundtrip(t *testing.T) {
|
|
c := newSyncSubnetIDs()
|
|
|
|
for i := 0; i < 20; i++ {
|
|
pubkey := [48]byte{byte(i)}
|
|
c.AddSyncCommitteeSubnets(pubkey[:], 100, []uint64{uint64(i)}, 0)
|
|
}
|
|
|
|
for i := uint64(0); i < 20; i++ {
|
|
pubkey := [48]byte{byte(i)}
|
|
|
|
idxs, _, ok, _ := c.GetSyncCommitteeSubnets(pubkey[:], 100)
|
|
if !ok {
|
|
t.Errorf("Couldn't find entry in cache for pubkey %#x", pubkey)
|
|
continue
|
|
}
|
|
require.Equal(t, i, idxs[0])
|
|
}
|
|
coms := c.GetAllSubnets(100)
|
|
assert.Equal(t, 20, len(coms))
|
|
}
|
|
|
|
func TestSyncSubnetIDsCache_ValidateCurrentEpoch(t *testing.T) {
|
|
c := newSyncSubnetIDs()
|
|
|
|
for i := 0; i < 20; i++ {
|
|
pubkey := [48]byte{byte(i)}
|
|
c.AddSyncCommitteeSubnets(pubkey[:], 100, []uint64{uint64(i)}, 0)
|
|
}
|
|
|
|
coms := c.GetAllSubnets(50)
|
|
assert.Equal(t, 0, len(coms))
|
|
|
|
for i := uint64(0); i < 20; i++ {
|
|
pubkey := [48]byte{byte(i)}
|
|
|
|
_, jEpoch, ok, _ := c.GetSyncCommitteeSubnets(pubkey[:], 100)
|
|
if !ok {
|
|
t.Errorf("Couldn't find entry in cache for pubkey %#x", pubkey)
|
|
continue
|
|
}
|
|
require.Equal(t, true, uint64(jEpoch) >= 100-params.BeaconConfig().SyncCommitteeSubnetCount)
|
|
}
|
|
|
|
coms = c.GetAllSubnets(99)
|
|
assert.Equal(t, 20, len(coms))
|
|
}
|