mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-24 12:27:18 +00:00
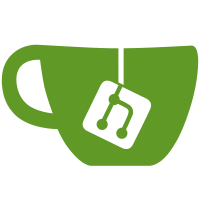
* Initial spec rewrite * Finish adding merkle tree implementation * Last bits * Move reverse function * Add comments * Add deposit tree snapshot * Add deposit tree * Add comments + cleanup * Fixes * Add missing errors * Small fixes * Add unhandled error * Cleanup * Fix unsafe file.Close * Add missing comments * Small fixes * Address some of deepSource' compaints * Add depositCount check * Add finalizedDeposit check * Replace pointer magic with copy() * Add test for slice reversal * add back bytes method * Add package level description * Remove zerohash gen and add additional checks * Add additional comments * Small lint fixes * Forgot an error * Small fixes * Move Uint64ToBytesLittleEndian32 + test * Fix uint subtraction issue * Move mixInLength below error handling * Fix * Fix deposit root * integrate 4881 * edits * added in deposit tree fetcher * add file * Add remaining fetcher functions * Add new file for inserter functions * Fixes and additional funcs * Cleanup * Add * Graph * pushed up edits * fix up * Updates * Add EIP4881 toggle flag * Add interfaces * Fix tests * More changes * Fix * Remove generated graph * Fix spacing * Changes * Fixes * Changes * Test Fix * gaz * Fix a couple tests * Fix last tests * define protos * proto methods * pushed * regen * Add proto funcs * builds * pushin up * Fix and cleanup * Fix spectest * General cleanup * add 4881 to e2e * Remove debug statements + remove test skip * Implement first set of missing methods * Replace Zerohashes + cleanup * gazelle * fmt * Put back defensive check * Add error logs * InsertFinalizedDeposits: return an error * Remove logging * Radek' Review * Lint fixes * build * Remove cancel * Update beacon-chain/deterministic-genesis/service.go Co-authored-by: Raul Jordan <raul@prysmaticlabs.com> * Update beacon-chain/cache/depositsnapshot/deposit_inserter.go Co-authored-by: Raul Jordan <raul@prysmaticlabs.com> * Cleanup * Fix panic when DepositSnapshot is nil on init * Gofmt * Fix RootEquivalence test * Gofmt * Add missing comments * Nishant' review * Add Insert benchmarks * fix up copy method * Fix deep copy * Fix conflicts * Return error * Fix linter issues * add in migration logic * Cleanup + tests * fix * Fix incorrect index in test * Fix linter * Gofmt * fix it * fixes for off by 1 * gaz * fix cast * fix it * remove ErrZeroIndex * Fix merkle_tree_test * add fallback * add fix for insertion bug * add many fixes * fix empty snapshot * clean up * use feature * remove check * fix failing tests * skip it * fix test * fix test again * fix for the last time * Apply suggestions from code review Co-authored-by: Radosław Kapka <rkapka@wp.pl> * fix it * remove cancel * fix for voting * addressing more comments * fix err * potuz's review * one more test * fix bad test * make 4881 part of dev mode * add workaround for new trie * comment * preston's review * james's review * add comment * james review * preston's review * remove skipped test * gaz --------- Co-authored-by: rauljordan <raul@prysmaticlabs.com> Co-authored-by: nisdas <nishdas93@gmail.com> Co-authored-by: Radosław Kapka <rkapka@wp.pl> Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com>
207 lines
6.7 KiB
Go
207 lines
6.7 KiB
Go
// Package interopcoldstart allows for spinning up a deterministic-genesis
|
|
// local chain without the need for eth1 deposits useful for
|
|
// local client development and interoperability testing.
|
|
package interopcoldstart
|
|
|
|
import (
|
|
"context"
|
|
"math/big"
|
|
"os"
|
|
"time"
|
|
|
|
"github.com/prysmaticlabs/prysm/v4/beacon-chain/cache"
|
|
"github.com/prysmaticlabs/prysm/v4/beacon-chain/db"
|
|
"github.com/prysmaticlabs/prysm/v4/beacon-chain/execution"
|
|
"github.com/prysmaticlabs/prysm/v4/beacon-chain/state"
|
|
state_native "github.com/prysmaticlabs/prysm/v4/beacon-chain/state/state-native"
|
|
"github.com/prysmaticlabs/prysm/v4/consensus-types/primitives"
|
|
ethpb "github.com/prysmaticlabs/prysm/v4/proto/prysm/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/v4/runtime"
|
|
"github.com/prysmaticlabs/prysm/v4/runtime/interop"
|
|
"github.com/prysmaticlabs/prysm/v4/time/slots"
|
|
)
|
|
|
|
var _ runtime.Service = (*Service)(nil)
|
|
var _ cache.FinalizedFetcher = (*Service)(nil)
|
|
var _ execution.ChainStartFetcher = (*Service)(nil)
|
|
|
|
// Service spins up an client interoperability service that handles responsibilities such
|
|
// as kickstarting a genesis state for the beacon node from cli flags or a genesis.ssz file.
|
|
type Service struct {
|
|
cfg *Config
|
|
ctx context.Context
|
|
cancel context.CancelFunc
|
|
chainStartDeposits []*ethpb.Deposit
|
|
}
|
|
|
|
// All of these methods are stubs as they are not used by a node running with deterministic-genesis.
|
|
|
|
func (s *Service) AllDepositContainers(ctx context.Context) []*ethpb.DepositContainer {
|
|
log.Errorf("AllDepositContainers should not be called")
|
|
return nil
|
|
}
|
|
|
|
func (s *Service) InsertPendingDeposit(ctx context.Context, d *ethpb.Deposit, blockNum uint64, index int64, depositRoot [32]byte) {
|
|
log.Errorf("InsertPendingDeposit should not be called")
|
|
}
|
|
|
|
func (s *Service) PendingDeposits(ctx context.Context, untilBlk *big.Int) []*ethpb.Deposit {
|
|
log.Errorf("PendingDeposits should not be called")
|
|
return nil
|
|
}
|
|
|
|
func (s *Service) PendingContainers(ctx context.Context, untilBlk *big.Int) []*ethpb.DepositContainer {
|
|
log.Errorf("PendingContainers should not be called")
|
|
return nil
|
|
}
|
|
|
|
func (s *Service) PrunePendingDeposits(ctx context.Context, merkleTreeIndex int64) {
|
|
log.Errorf("PrunePendingDeposits should not be called")
|
|
}
|
|
|
|
func (s *Service) PruneProofs(ctx context.Context, untilDepositIndex int64) error {
|
|
log.Errorf("PruneProofs should not be called")
|
|
return nil
|
|
}
|
|
|
|
// Config options for the interop service.
|
|
type Config struct {
|
|
GenesisTime uint64
|
|
NumValidators uint64
|
|
BeaconDB db.HeadAccessDatabase
|
|
DepositCache cache.DepositCache
|
|
GenesisPath string
|
|
}
|
|
|
|
// NewService is an interoperability testing service to inject a deterministically generated genesis state
|
|
// into the beacon chain database and running services at start up. This service should not be used in production
|
|
// as it does not have any value other than ease of use for testing purposes.
|
|
func NewService(ctx context.Context, cfg *Config) *Service {
|
|
ctx, cancel := context.WithCancel(ctx)
|
|
|
|
return &Service{
|
|
cfg: cfg,
|
|
ctx: ctx,
|
|
cancel: cancel,
|
|
}
|
|
}
|
|
|
|
// Start initializes the genesis state from configured flags.
|
|
func (s *Service) Start() {
|
|
log.Warn("Saving generated genesis state in database for interop testing")
|
|
|
|
if s.cfg.GenesisPath != "" {
|
|
data, err := os.ReadFile(s.cfg.GenesisPath)
|
|
if err != nil {
|
|
log.WithError(err).Fatal("Could not read pre-loaded state")
|
|
}
|
|
genesisState := ðpb.BeaconState{}
|
|
if err := genesisState.UnmarshalSSZ(data); err != nil {
|
|
log.WithError(err).Fatal("Could not unmarshal pre-loaded state")
|
|
}
|
|
genesisTrie, err := state_native.InitializeFromProtoPhase0(genesisState)
|
|
if err != nil {
|
|
log.WithError(err).Fatal("Could not get state trie")
|
|
}
|
|
if err := s.saveGenesisState(s.ctx, genesisTrie); err != nil {
|
|
log.WithError(err).Fatal("Could not save interop genesis state")
|
|
}
|
|
return
|
|
}
|
|
|
|
// Save genesis state in db
|
|
genesisState, _, err := interop.GenerateGenesisState(s.ctx, s.cfg.GenesisTime, s.cfg.NumValidators)
|
|
if err != nil {
|
|
log.WithError(err).Fatal("Could not generate interop genesis state")
|
|
}
|
|
genesisTrie, err := state_native.InitializeFromProtoPhase0(genesisState)
|
|
if err != nil {
|
|
log.WithError(err).Fatal("Could not get state trie")
|
|
}
|
|
if s.cfg.GenesisTime == 0 {
|
|
// Generated genesis time; fetch it
|
|
s.cfg.GenesisTime = genesisTrie.GenesisTime()
|
|
}
|
|
gRoot, err := genesisTrie.HashTreeRoot(s.ctx)
|
|
if err != nil {
|
|
log.WithError(err).Fatal("Could not hash tree root genesis state")
|
|
}
|
|
go slots.CountdownToGenesis(s.ctx, time.Unix(int64(s.cfg.GenesisTime), 0), s.cfg.NumValidators, gRoot)
|
|
|
|
if err := s.saveGenesisState(s.ctx, genesisTrie); err != nil {
|
|
log.WithError(err).Fatal("Could not save interop genesis state")
|
|
}
|
|
}
|
|
|
|
// Stop does nothing.
|
|
func (_ *Service) Stop() error {
|
|
return nil
|
|
}
|
|
|
|
// Status always returns nil.
|
|
func (_ *Service) Status() error {
|
|
return nil
|
|
}
|
|
|
|
// AllDeposits mocks out the deposit cache functionality for interop.
|
|
func (_ *Service) AllDeposits(_ context.Context, _ *big.Int) []*ethpb.Deposit {
|
|
return []*ethpb.Deposit{}
|
|
}
|
|
|
|
// ChainStartEth1Data mocks out the powchain functionality for interop.
|
|
func (_ *Service) ChainStartEth1Data() *ethpb.Eth1Data {
|
|
return ðpb.Eth1Data{}
|
|
}
|
|
|
|
// PreGenesisState returns an empty beacon state.
|
|
func (_ *Service) PreGenesisState() state.BeaconState {
|
|
s, err := state_native.InitializeFromProtoPhase0(ðpb.BeaconState{})
|
|
if err != nil {
|
|
panic("could not initialize state")
|
|
}
|
|
return s
|
|
}
|
|
|
|
// ClearPreGenesisData --
|
|
func (_ *Service) ClearPreGenesisData() {
|
|
// no-op
|
|
}
|
|
|
|
// DepositByPubkey mocks out the deposit cache functionality for interop.
|
|
func (_ *Service) DepositByPubkey(_ context.Context, _ []byte) (*ethpb.Deposit, *big.Int) {
|
|
return ðpb.Deposit{}, nil
|
|
}
|
|
|
|
// DepositsNumberAndRootAtHeight mocks out the deposit cache functionality for interop.
|
|
func (_ *Service) DepositsNumberAndRootAtHeight(_ context.Context, _ *big.Int) (uint64, [32]byte) {
|
|
return 0, [32]byte{}
|
|
}
|
|
|
|
// FinalizedDeposits mocks out the deposit cache functionality for interop.
|
|
func (_ *Service) FinalizedDeposits(ctx context.Context) (cache.FinalizedDeposits, error) {
|
|
return nil, nil
|
|
}
|
|
|
|
// NonFinalizedDeposits mocks out the deposit cache functionality for interop.
|
|
func (_ *Service) NonFinalizedDeposits(_ context.Context, _ int64, _ *big.Int) []*ethpb.Deposit {
|
|
return []*ethpb.Deposit{}
|
|
}
|
|
|
|
func (s *Service) saveGenesisState(ctx context.Context, genesisState state.BeaconState) error {
|
|
if err := s.cfg.BeaconDB.SaveGenesisData(ctx, genesisState); err != nil {
|
|
return err
|
|
}
|
|
|
|
s.chainStartDeposits = make([]*ethpb.Deposit, genesisState.NumValidators())
|
|
|
|
for i := primitives.ValidatorIndex(0); uint64(i) < uint64(genesisState.NumValidators()); i++ {
|
|
pk := genesisState.PubkeyAtIndex(i)
|
|
s.chainStartDeposits[i] = ðpb.Deposit{
|
|
Data: ðpb.Deposit_Data{
|
|
PublicKey: pk[:],
|
|
},
|
|
}
|
|
}
|
|
return nil
|
|
}
|