mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 12:57:18 +00:00
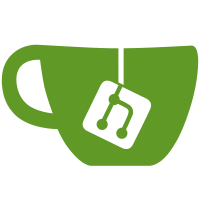
* more spanner additions * implement iface * begin implement * wrapped up spanner functions * rem interface * added in necessary comments * comments on enums * begin adding tests * plug in surround vote detection * saved indexed db implementation * finally plugin slashing for historical data * Small fixes * add in all gazelle * save incoming new functions * resolve todo * fix broken test channel item * tests passing when fixing certain arguments and setups * Add comment and change unimplemented * find surround * added in gazelle * gazz * feedback from shay * fixed up naming * Update * Add tests for detectSurroundVotes * Remove logs * Fix slasher test * formatting * Remove unneeded condition * Test indices better * fixing broken build * pass tests * skip tests * imports * Update slasher/detection/attestations/attestations_test.go * Update slasher/beaconclient/historical_data_retrieval_test.go * Address comments * Rename function * Add comment for future optimization * Fix comment Co-authored-by: Ivan Martinez <ivanthegreatdev@gmail.com>
51 lines
1.8 KiB
Go
51 lines
1.8 KiB
Go
package blockchain
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
ethpb "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/core/helpers"
|
|
stateTrie "github.com/prysmaticlabs/prysm/beacon-chain/state"
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
"github.com/sirupsen/logrus"
|
|
)
|
|
|
|
var log = logrus.WithField("prefix", "blockchain")
|
|
|
|
// logs state transition related data every slot.
|
|
func logStateTransitionData(b *ethpb.BeaconBlock) {
|
|
log.WithFields(logrus.Fields{
|
|
"slot": b.Slot,
|
|
"attestations": len(b.Body.Attestations),
|
|
"deposits": len(b.Body.Deposits),
|
|
"attesterSlashings": len(b.Body.AttesterSlashings),
|
|
}).Info("Finished applying state transition")
|
|
}
|
|
|
|
func logEpochData(beaconState *stateTrie.BeaconState) {
|
|
log.WithFields(logrus.Fields{
|
|
"epoch": helpers.CurrentEpoch(beaconState),
|
|
"finalizedEpoch": beaconState.FinalizedCheckpointEpoch(),
|
|
"justifiedEpoch": beaconState.CurrentJustifiedCheckpoint().Epoch,
|
|
"previousJustifiedEpoch": beaconState.PreviousJustifiedCheckpoint().Epoch,
|
|
}).Info("Starting next epoch")
|
|
activeVals, err := helpers.ActiveValidatorIndices(beaconState, helpers.CurrentEpoch(beaconState))
|
|
if err != nil {
|
|
log.WithError(err).Error("Could not get active validator indices")
|
|
return
|
|
}
|
|
log.WithFields(logrus.Fields{
|
|
"totalValidators": len(beaconState.Validators()),
|
|
"activeValidators": len(activeVals),
|
|
"averageBalance": fmt.Sprintf("%.5f ETH", averageBalance(beaconState.Balances())),
|
|
}).Info("Validator registry information")
|
|
}
|
|
|
|
func averageBalance(balances []uint64) float64 {
|
|
total := uint64(0)
|
|
for i := 0; i < len(balances); i++ {
|
|
total += balances[i]
|
|
}
|
|
return float64(total) / float64(len(balances)) / float64(params.BeaconConfig().GweiPerEth)
|
|
}
|