mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-22 03:30:35 +00:00
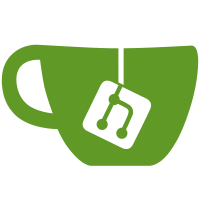
* Add AFL third_party libraries * add beacon state fuzzing, add afl fuzz bundle * rm fuzzing engine * fix and lint * Check for array out of bounds when calculating proposer delta * failing test * fix * Checkpoint progress * Add requirement that inclusion distance is not zero, add regression test * No need for HTR since that is covered in process slots * Removing some fuzzit logic, old fuzz tests * Add ssz encoder test and fix * Fuzzing checkpoint, adding fuzzing to the p2p layer * ignore some libfuzzer files * Full testing of p2p processing of blocks, with some mocked stuff * use tmpdir and always process blocks * use checkptr * Update ethereumapis * go mod tidy * benchmarks for ferran's fast ssz hash tree root * Update fastssz * fmt * gaz * goimports * Fix * fix ethereumapis * fix again * kafka * fix gen file * fix compute signing root * gofmt * checkpoint progress * progress * checkpoint * updates * updates * merge fix * WIP * merge * fix build * fix merge related issues * cleanup * revert unrelated * lint * lint * lint * manual tags for fuzz * Commentary on upload script * some import fixes, but not all * fix //fuzz:fuzz_tests * rm unused test * update generated ssz * Set // +build libfuzzer * remove debug code * A bit of refactoring ot explain why there is a committee_disabled file Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com>
133 lines
2.8 KiB
Go
Executable File
133 lines
2.8 KiB
Go
Executable File
// Code generated by fastssz. DO NOT EDIT.
|
|
package fuzz
|
|
|
|
import (
|
|
ssz "github.com/ferranbt/fastssz"
|
|
ethpb "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
pb "github.com/prysmaticlabs/prysm/proto/beacon/p2p/v1"
|
|
)
|
|
|
|
// MarshalSSZ ssz marshals the InputBlockWithPrestate object
|
|
func (i *InputBlockWithPrestate) MarshalSSZ() ([]byte, error) {
|
|
return ssz.MarshalSSZ(i)
|
|
}
|
|
|
|
// MarshalSSZTo ssz marshals the InputBlockWithPrestate object to a target array
|
|
func (i *InputBlockWithPrestate) MarshalSSZTo(buf []byte) (dst []byte, err error) {
|
|
dst = buf
|
|
offset := int(8)
|
|
|
|
// Offset (0) 'State'
|
|
dst = ssz.WriteOffset(dst, offset)
|
|
if i.State == nil {
|
|
i.State = new(pb.BeaconState)
|
|
}
|
|
offset += i.State.SizeSSZ()
|
|
|
|
// Offset (1) 'Block'
|
|
dst = ssz.WriteOffset(dst, offset)
|
|
if i.Block == nil {
|
|
i.Block = new(ethpb.SignedBeaconBlock)
|
|
}
|
|
offset += i.Block.SizeSSZ()
|
|
|
|
// Field (0) 'State'
|
|
if dst, err = i.State.MarshalSSZTo(dst); err != nil {
|
|
return
|
|
}
|
|
|
|
// Field (1) 'Block'
|
|
if dst, err = i.Block.MarshalSSZTo(dst); err != nil {
|
|
return
|
|
}
|
|
|
|
return
|
|
}
|
|
|
|
// UnmarshalSSZ ssz unmarshals the InputBlockWithPrestate object
|
|
func (i *InputBlockWithPrestate) UnmarshalSSZ(buf []byte) error {
|
|
var err error
|
|
size := uint64(len(buf))
|
|
if size < 8 {
|
|
return ssz.ErrSize
|
|
}
|
|
|
|
tail := buf
|
|
var o0, o1 uint64
|
|
|
|
// Offset (0) 'State'
|
|
if o0 = ssz.ReadOffset(buf[0:4]); o0 > size {
|
|
return ssz.ErrOffset
|
|
}
|
|
|
|
// Offset (1) 'Block'
|
|
if o1 = ssz.ReadOffset(buf[4:8]); o1 > size || o0 > o1 {
|
|
return ssz.ErrOffset
|
|
}
|
|
|
|
// Field (0) 'State'
|
|
{
|
|
buf = tail[o0:o1]
|
|
if i.State == nil {
|
|
i.State = new(pb.BeaconState)
|
|
}
|
|
if err = i.State.UnmarshalSSZ(buf); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
|
|
// Field (1) 'Block'
|
|
{
|
|
buf = tail[o1:]
|
|
if i.Block == nil {
|
|
i.Block = new(ethpb.SignedBeaconBlock)
|
|
}
|
|
if err = i.Block.UnmarshalSSZ(buf); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
return err
|
|
}
|
|
|
|
// SizeSSZ returns the ssz encoded size in bytes for the InputBlockWithPrestate object
|
|
func (i *InputBlockWithPrestate) SizeSSZ() (size int) {
|
|
size = 8
|
|
|
|
// Field (0) 'State'
|
|
if i.State == nil {
|
|
i.State = new(pb.BeaconState)
|
|
}
|
|
size += i.State.SizeSSZ()
|
|
|
|
// Field (1) 'Block'
|
|
if i.Block == nil {
|
|
i.Block = new(ethpb.SignedBeaconBlock)
|
|
}
|
|
size += i.Block.SizeSSZ()
|
|
|
|
return
|
|
}
|
|
|
|
// HashTreeRoot ssz hashes the InputBlockWithPrestate object
|
|
func (i *InputBlockWithPrestate) HashTreeRoot() ([32]byte, error) {
|
|
return ssz.HashWithDefaultHasher(i)
|
|
}
|
|
|
|
// HashTreeRootWith ssz hashes the InputBlockWithPrestate object with a hasher
|
|
func (i *InputBlockWithPrestate) HashTreeRootWith(hh *ssz.Hasher) (err error) {
|
|
indx := hh.Index()
|
|
|
|
// Field (0) 'State'
|
|
if err = i.State.HashTreeRootWith(hh); err != nil {
|
|
return
|
|
}
|
|
|
|
// Field (1) 'Block'
|
|
if err = i.Block.HashTreeRootWith(hh); err != nil {
|
|
return
|
|
}
|
|
|
|
hh.Merkleize(indx)
|
|
return
|
|
}
|