mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
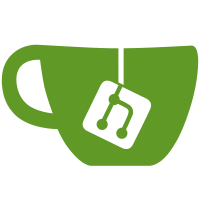
* Add in context * use better function signatures * add in chainservice * fix rpc requests * add in test * fix tests * add in comments * Update beacon-chain/sync/rpc_chunked_response.go Co-authored-by: terence tsao <terence@prysmaticlabs.com> * Update beacon-chain/sync/rpc_send_request.go Co-authored-by: terence tsao <terence@prysmaticlabs.com> * rename files * fmt Co-authored-by: Raul Jordan <raul@prysmaticlabs.com> Co-authored-by: terence tsao <terence@prysmaticlabs.com> Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com>
73 lines
1.9 KiB
Go
73 lines
1.9 KiB
Go
package sync
|
|
|
|
import (
|
|
"context"
|
|
"sync"
|
|
"testing"
|
|
"time"
|
|
|
|
core "github.com/libp2p/go-libp2p-core"
|
|
"github.com/libp2p/go-libp2p-core/network"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/p2p"
|
|
p2ptest "github.com/prysmaticlabs/prysm/beacon-chain/p2p/testing"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/assert"
|
|
)
|
|
|
|
func TestContextWrite_NoWrites(t *testing.T) {
|
|
p1 := p2ptest.NewTestP2P(t)
|
|
nPeer := p2ptest.NewTestP2P(t)
|
|
p1.Connect(nPeer)
|
|
|
|
wg := new(sync.WaitGroup)
|
|
prID := p2p.RPCPingTopicV1
|
|
wg.Add(1)
|
|
nPeer.BHost.SetStreamHandler(core.ProtocolID(prID), func(stream network.Stream) {
|
|
wg.Done()
|
|
// no-op
|
|
})
|
|
strm, err := p1.BHost.NewStream(context.Background(), nPeer.PeerID(), p2p.RPCPingTopicV1)
|
|
assert.NoError(t, err)
|
|
|
|
// Nothing will be written to the stream
|
|
assert.NoError(t, writeContextToStream(strm, nil))
|
|
if testutil.WaitTimeout(wg, 1*time.Second) {
|
|
t.Fatal("Did not receive stream within 1 sec")
|
|
}
|
|
}
|
|
|
|
func TestContextRead_NoReads(t *testing.T) {
|
|
p1 := p2ptest.NewTestP2P(t)
|
|
nPeer := p2ptest.NewTestP2P(t)
|
|
p1.Connect(nPeer)
|
|
|
|
wg := new(sync.WaitGroup)
|
|
prID := p2p.RPCPingTopicV1
|
|
wg.Add(1)
|
|
wantedData := []byte{'A', 'B', 'C', 'D'}
|
|
nPeer.BHost.SetStreamHandler(core.ProtocolID(prID), func(stream network.Stream) {
|
|
// No Context will be read from it
|
|
dt, err := readContextFromStream(stream, nil)
|
|
assert.NoError(t, err)
|
|
assert.Equal(t, 0, len(dt))
|
|
|
|
// Ensure sent over data hasn't been modified.
|
|
buf := make([]byte, len(wantedData))
|
|
n, err := stream.Read(buf)
|
|
assert.NoError(t, err)
|
|
assert.Equal(t, len(wantedData), n)
|
|
assert.DeepEqual(t, wantedData, buf)
|
|
|
|
wg.Done()
|
|
})
|
|
strm, err := p1.BHost.NewStream(context.Background(), nPeer.PeerID(), p2p.RPCPingTopicV1)
|
|
assert.NoError(t, err)
|
|
|
|
n, err := strm.Write(wantedData)
|
|
assert.NoError(t, err)
|
|
assert.Equal(t, len(wantedData), n)
|
|
if testutil.WaitTimeout(wg, 1*time.Second) {
|
|
t.Fatal("Did not receive stream within 1 sec")
|
|
}
|
|
}
|