mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-22 11:32:09 +00:00
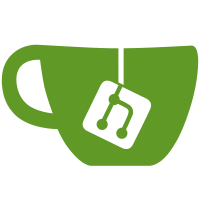
* first version of the watchtower api * first commit * remove watchtower * working version * fix < 0 * gaz * Update slasher/db/db.go * remove clear history * moved constant to config * gaz * feedback changes * compare uint64 * add constant config * PruneSlasherStoragePeriod change
104 lines
2.4 KiB
Go
104 lines
2.4 KiB
Go
package db
|
|
|
|
import (
|
|
"io"
|
|
"os"
|
|
"path"
|
|
"time"
|
|
|
|
"github.com/boltdb/bolt"
|
|
"github.com/pkg/errors"
|
|
"github.com/sirupsen/logrus"
|
|
)
|
|
|
|
var log = logrus.WithField("prefix", "beacondb")
|
|
|
|
// Database defines the necessary methods for slasher service which may
|
|
// be implemented by any key-value or relational database in practice.
|
|
type Database interface {
|
|
io.Closer
|
|
DatabasePath() string
|
|
|
|
ClearDB() error
|
|
}
|
|
|
|
// Store defines an implementation of the Prysm Database interface
|
|
// using BoltDB as the underlying persistent kv-store for eth2.
|
|
type Store struct {
|
|
db *bolt.DB
|
|
databasePath string
|
|
}
|
|
|
|
// Close closes the underlying boltdb database.
|
|
func (db *Store) Close() error {
|
|
return db.db.Close()
|
|
}
|
|
|
|
func (db *Store) update(fn func(*bolt.Tx) error) error {
|
|
return db.db.Update(fn)
|
|
}
|
|
func (db *Store) batch(fn func(*bolt.Tx) error) error {
|
|
return db.db.Batch(fn)
|
|
}
|
|
func (db *Store) view(fn func(*bolt.Tx) error) error {
|
|
return db.db.View(fn)
|
|
}
|
|
|
|
// NewDB initializes a new DB.
|
|
func NewDB(dirPath string) (*Store, error) {
|
|
return NewKVStore(dirPath)
|
|
}
|
|
|
|
// ClearDB removes the previously stored directory at the data directory.
|
|
func (db *Store) ClearDB() error {
|
|
if _, err := os.Stat(db.databasePath); os.IsNotExist(err) {
|
|
return nil
|
|
}
|
|
return os.RemoveAll(db.databasePath)
|
|
}
|
|
|
|
// DatabasePath at which this database writes files.
|
|
func (db *Store) DatabasePath() string {
|
|
return db.databasePath
|
|
}
|
|
|
|
func createBuckets(tx *bolt.Tx, buckets ...[]byte) error {
|
|
for _, bucket := range buckets {
|
|
if _, err := tx.CreateBucketIfNotExists(bucket); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// NewKVStore initializes a new boltDB key-value store at the directory
|
|
// path specified, creates the kv-buckets based on the schema, and stores
|
|
// an open connection db object as a property of the Store struct.
|
|
func NewKVStore(dirPath string) (*Store, error) {
|
|
if err := os.MkdirAll(dirPath, 0700); err != nil {
|
|
return nil, err
|
|
}
|
|
datafile := path.Join(dirPath, "slasher.db")
|
|
boltDB, err := bolt.Open(datafile, 0600, &bolt.Options{Timeout: 1 * time.Second})
|
|
if err != nil {
|
|
if err == bolt.ErrTimeout {
|
|
return nil, errors.New("cannot obtain database lock, database may be in use by another process")
|
|
}
|
|
return nil, err
|
|
}
|
|
|
|
kv := &Store{db: boltDB, databasePath: dirPath}
|
|
|
|
if err := kv.db.Update(func(tx *bolt.Tx) error {
|
|
return createBuckets(
|
|
tx,
|
|
historicAttestationsBucket,
|
|
historicBlockHeadersBucket,
|
|
)
|
|
}); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return kv, err
|
|
}
|