mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
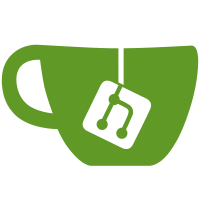
* Optimize marshal and unmarshal * Merge branch 'master' into slasher-optimize-span * Merge branch 'master' into slasher-optimize-span * Add tests and comments * Merge branch 'slasher-optimize-span' of github.com:prysmaticlabs/prysm into slasher-optimize-span * Merge branch 'master' of github.com:prysmaticlabs/prysm into slasher-optimize-span * Merge refs/heads/master into slasher-optimize-span * Merge refs/heads/master into slasher-optimize-span * Merge refs/heads/master into slasher-optimize-span
75 lines
2.7 KiB
Go
75 lines
2.7 KiB
Go
package beaconclient
|
|
|
|
import (
|
|
"context"
|
|
"strings"
|
|
|
|
ethpb "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/core/helpers"
|
|
"github.com/prysmaticlabs/prysm/shared/sliceutil"
|
|
"github.com/sirupsen/logrus"
|
|
"go.opencensus.io/trace"
|
|
)
|
|
|
|
// subscribeDetectedProposerSlashings subscribes to an event feed for
|
|
// slashing objects from the slasher runtime. Upon receiving
|
|
// a proposer slashing from the feed, we submit the object to the
|
|
// connected beacon node via a client RPC.
|
|
func (bs *Service) subscribeDetectedProposerSlashings(ctx context.Context, ch chan *ethpb.ProposerSlashing) {
|
|
ctx, span := trace.StartSpan(ctx, "beaconclient.submitProposerSlashing")
|
|
defer span.End()
|
|
sub := bs.proposerSlashingsFeed.Subscribe(ch)
|
|
defer sub.Unsubscribe()
|
|
for {
|
|
select {
|
|
case slashing := <-ch:
|
|
if _, err := bs.beaconClient.SubmitProposerSlashing(ctx, slashing); err != nil {
|
|
log.Error(err)
|
|
}
|
|
case <-sub.Err():
|
|
log.Error("Subscriber closed, exiting goroutine")
|
|
return
|
|
case <-ctx.Done():
|
|
log.Error("Context canceled")
|
|
return
|
|
}
|
|
}
|
|
}
|
|
|
|
// subscribeDetectedAttesterSlashings subscribes to an event feed for
|
|
// slashing objects from the slasher runtime. Upon receiving an
|
|
// attester slashing from the feed, we submit the object to the
|
|
// connected beacon node via a client RPC.
|
|
func (bs *Service) subscribeDetectedAttesterSlashings(ctx context.Context, ch chan *ethpb.AttesterSlashing) {
|
|
ctx, span := trace.StartSpan(ctx, "beaconclient.submitAttesterSlashing")
|
|
defer span.End()
|
|
sub := bs.attesterSlashingsFeed.Subscribe(ch)
|
|
defer sub.Unsubscribe()
|
|
for {
|
|
select {
|
|
case slashing := <-ch:
|
|
if slashing != nil && slashing.Attestation_1 != nil && slashing.Attestation_2 != nil {
|
|
slashableIndices := sliceutil.IntersectionUint64(slashing.Attestation_1.AttestingIndices, slashing.Attestation_2.AttestingIndices)
|
|
_, err := bs.beaconClient.SubmitAttesterSlashing(ctx, slashing)
|
|
if err == nil {
|
|
log.WithFields(logrus.Fields{
|
|
"sourceEpoch": slashing.Attestation_1.Data.Source.Epoch,
|
|
"targetEpoch": slashing.Attestation_1.Data.Target.Epoch,
|
|
"indices": slashableIndices,
|
|
}).Info("Found a valid attester slashing! Submitting to beacon node")
|
|
} else if strings.Contains(err.Error(), helpers.ErrSigFailedToVerify.Error()) {
|
|
log.WithError(err).Errorf("Could not submit attester slashing with indices %v", slashableIndices)
|
|
} else if !strings.Contains(err.Error(), "could not slash") {
|
|
log.WithError(err).Errorf("Could not slash validators with indices %v", slashableIndices)
|
|
}
|
|
}
|
|
case <-sub.Err():
|
|
log.Error("Subscriber closed, exiting goroutine")
|
|
return
|
|
case <-ctx.Done():
|
|
log.Error("Context canceled")
|
|
return
|
|
}
|
|
}
|
|
}
|