mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
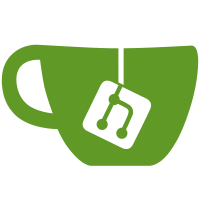
* first working implementation * assertions tests * adds to requires * merges assert and require tests into a single suite * gazelle * Merge branch 'merge-assert-require-tests' into assert-logs-contains-move-to-assertions * gazelle * updates references * fixes build issue * Merge branch 'master' into assert-logs-contains-move-to-assertions * Merge refs/heads/master into assert-logs-contains-move-to-assertions * Merge branch 'master' into assert-logs-contains-move-to-assertions * fixes build issue * Merge branch 'assert-logs-contains-move-to-assertions' of github.com:prysmaticlabs/prysm into assert-logs-contains-move-to-assertions * Merge refs/heads/master into assert-logs-contains-move-to-assertions * Merge refs/heads/master into assert-logs-contains-move-to-assertions
99 lines
2.5 KiB
Go
99 lines
2.5 KiB
Go
package beaconclient
|
|
|
|
import (
|
|
"context"
|
|
"testing"
|
|
|
|
"github.com/golang/mock/gomock"
|
|
ethpb "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/shared/event"
|
|
"github.com/prysmaticlabs/prysm/shared/mock"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/require"
|
|
logTest "github.com/sirupsen/logrus/hooks/test"
|
|
)
|
|
|
|
func TestService_SubscribeDetectedProposerSlashings(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
ctrl := gomock.NewController(t)
|
|
defer ctrl.Finish()
|
|
client := mock.NewMockBeaconChainClient(ctrl)
|
|
|
|
bs := Service{
|
|
beaconClient: client,
|
|
proposerSlashingsFeed: new(event.Feed),
|
|
}
|
|
|
|
slashing := ðpb.ProposerSlashing{
|
|
Header_1: ðpb.SignedBeaconBlockHeader{
|
|
Header: ðpb.BeaconBlockHeader{
|
|
ProposerIndex: 5,
|
|
Slot: 5,
|
|
},
|
|
Signature: make([]byte, 96),
|
|
},
|
|
Header_2: ðpb.SignedBeaconBlockHeader{
|
|
Header: ðpb.BeaconBlockHeader{
|
|
ProposerIndex: 5,
|
|
Slot: 5,
|
|
},
|
|
Signature: make([]byte, 96),
|
|
},
|
|
}
|
|
|
|
exitRoutine := make(chan bool)
|
|
slashingsChan := make(chan *ethpb.ProposerSlashing)
|
|
ctx, cancel := context.WithCancel(context.Background())
|
|
client.EXPECT().SubmitProposerSlashing(gomock.Any(), slashing)
|
|
go func(tt *testing.T) {
|
|
bs.subscribeDetectedProposerSlashings(ctx, slashingsChan)
|
|
<-exitRoutine
|
|
}(t)
|
|
slashingsChan <- slashing
|
|
cancel()
|
|
exitRoutine <- true
|
|
require.LogsContain(t, hook, "Context canceled")
|
|
}
|
|
|
|
func TestService_SubscribeDetectedAttesterSlashings(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
ctrl := gomock.NewController(t)
|
|
defer ctrl.Finish()
|
|
client := mock.NewMockBeaconChainClient(ctrl)
|
|
|
|
bs := Service{
|
|
beaconClient: client,
|
|
attesterSlashingsFeed: new(event.Feed),
|
|
}
|
|
|
|
slashing := ðpb.AttesterSlashing{
|
|
Attestation_1: ðpb.IndexedAttestation{
|
|
AttestingIndices: []uint64{1, 2, 3},
|
|
Data: ðpb.AttestationData{
|
|
Source: ðpb.Checkpoint{
|
|
Epoch: 3,
|
|
},
|
|
Target: ðpb.Checkpoint{
|
|
Epoch: 4,
|
|
},
|
|
},
|
|
},
|
|
Attestation_2: ðpb.IndexedAttestation{
|
|
AttestingIndices: []uint64{3, 4, 5},
|
|
Data: nil,
|
|
},
|
|
}
|
|
|
|
exitRoutine := make(chan bool)
|
|
slashingsChan := make(chan *ethpb.AttesterSlashing)
|
|
ctx, cancel := context.WithCancel(context.Background())
|
|
client.EXPECT().SubmitAttesterSlashing(gomock.Any(), slashing)
|
|
go func(tt *testing.T) {
|
|
bs.subscribeDetectedAttesterSlashings(ctx, slashingsChan)
|
|
<-exitRoutine
|
|
}(t)
|
|
slashingsChan <- slashing
|
|
cancel()
|
|
exitRoutine <- true
|
|
require.LogsContain(t, hook, "Context canceled")
|
|
}
|