mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-22 03:30:35 +00:00
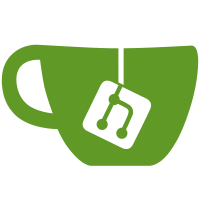
* First take at updating everything to v5 * Patch gRPC gateway to use prysm v5 Fix patch * Update go ssz --------- Co-authored-by: Preston Van Loon <pvanloon@offchainlabs.com>
41 lines
803 B
Go
41 lines
803 B
Go
package async_test
|
|
|
|
import (
|
|
"context"
|
|
"sync/atomic"
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/prysmaticlabs/prysm/v5/async"
|
|
)
|
|
|
|
func TestEveryRuns(t *testing.T) {
|
|
ctx, cancel := context.WithCancel(context.Background())
|
|
|
|
i := int32(0)
|
|
async.RunEvery(ctx, 100*time.Millisecond, func() {
|
|
atomic.AddInt32(&i, 1)
|
|
})
|
|
|
|
// Sleep for a bit and ensure the value has increased.
|
|
time.Sleep(200 * time.Millisecond)
|
|
|
|
if atomic.LoadInt32(&i) == 0 {
|
|
t.Error("Counter failed to increment with ticker")
|
|
}
|
|
|
|
cancel()
|
|
|
|
// Sleep for a bit to let the cancel take place.
|
|
time.Sleep(100 * time.Millisecond)
|
|
|
|
last := atomic.LoadInt32(&i)
|
|
|
|
// Sleep for a bit and ensure the value has not increased.
|
|
time.Sleep(200 * time.Millisecond)
|
|
|
|
if atomic.LoadInt32(&i) != last {
|
|
t.Error("Counter incremented after stop")
|
|
}
|
|
}
|