mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
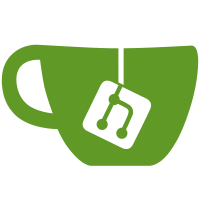
* replace eth2 types * replace protos * regen proto * replace * gaz * deps * amend * regen proto * mod * gaz * gaz * ensure build * ssz * add dep * no more eth2 types * no more eth2 * remg * all builds * buidl * tidy * clean * fmt * val serv * gaz Co-authored-by: Preston Van Loon <preston@prysmaticlabs.com>
94 lines
2.0 KiB
Go
94 lines
2.0 KiB
Go
package slashings
|
|
|
|
import (
|
|
"testing"
|
|
|
|
types "github.com/prysmaticlabs/prysm/consensus-types/primitives"
|
|
ethpb "github.com/prysmaticlabs/prysm/proto/prysm/v1alpha1"
|
|
)
|
|
|
|
func TestIsSurround(t *testing.T) {
|
|
type args struct {
|
|
a *ethpb.IndexedAttestation
|
|
b *ethpb.IndexedAttestation
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
args args
|
|
want bool
|
|
}{
|
|
{
|
|
name: "0 values returns false",
|
|
args: args{
|
|
a: createAttestation(0, 0),
|
|
b: createAttestation(0, 0),
|
|
},
|
|
want: false,
|
|
},
|
|
{
|
|
name: "detects surrounding attestation",
|
|
args: args{
|
|
a: createAttestation(2, 5),
|
|
b: createAttestation(3, 4),
|
|
},
|
|
want: true,
|
|
},
|
|
{
|
|
name: "new attestation source == old source, but new target < old target",
|
|
args: args{
|
|
a: createAttestation(3, 5),
|
|
b: createAttestation(3, 4),
|
|
},
|
|
want: false,
|
|
},
|
|
{
|
|
name: "new attestation source > old source, but new target == old target",
|
|
args: args{
|
|
a: createAttestation(3, 5),
|
|
b: createAttestation(4, 5),
|
|
},
|
|
want: false,
|
|
},
|
|
{
|
|
name: "new attestation source and targets equal to old one",
|
|
args: args{
|
|
a: createAttestation(3, 5),
|
|
b: createAttestation(3, 5),
|
|
},
|
|
want: false,
|
|
},
|
|
{
|
|
name: "new attestation source == old source, but new target > old target",
|
|
args: args{
|
|
a: createAttestation(3, 5),
|
|
b: createAttestation(3, 6),
|
|
},
|
|
want: false,
|
|
},
|
|
{
|
|
name: "new attestation source < old source, but new target == old target",
|
|
args: args{
|
|
a: createAttestation(3, 5),
|
|
b: createAttestation(2, 5),
|
|
},
|
|
want: false,
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
if got := IsSurround(tt.args.a, tt.args.b); got != tt.want {
|
|
t.Errorf("IsSurrounding() = %v, want %v", got, tt.want)
|
|
}
|
|
})
|
|
}
|
|
}
|
|
|
|
func createAttestation(source, target types.Epoch) *ethpb.IndexedAttestation {
|
|
return ðpb.IndexedAttestation{
|
|
Data: ðpb.AttestationData{
|
|
Source: ðpb.Checkpoint{Epoch: source},
|
|
Target: ðpb.Checkpoint{Epoch: target},
|
|
},
|
|
}
|
|
}
|