mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
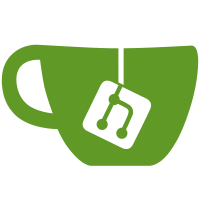
* add main.go * interop readme * proper visibility * standardize and abstract into simpler funcs * formatting * no os pkg * add test * no panics anywhere, properly and nicely handle errors * proper comments * fix broken test * readme * comment * recommend ssz * install * tool now works * README * build * readme * 64 validators * rem print * register the no powchain flag * work on mock eth1 start * common interface * getting closer with the interface defs * only two uses of powchain * remove powchain dependency * remove powchain dependency * common powchain interface * proper comment in case of flag * proper args into rpc services * rename fields * pass in mock flag into RPC * conforms to iface * use client instead of block fetcher iface * broken tests * block fetcher * finalized * resolved broken build * fix build * comment * fix tests * tests pass * resolved confs * took them out * rename into smaller interfaces * resolve some confs * ensure tests pass * properly utilize mock instead of localized mock * res lint * lint * finish test for mock eth1data * run gazelle * include flag again * fix broken build * disable powchain * dont dial eth1 nodes * reenable pow * use smaller interfaces, standardize naming * abstract mock into its own package * faulty mock lint * fix stutter in lint * rpc tests all passing * use mocks for operations * no more mocks in the entire rpc package * no mock * viz * testonly
102 lines
2.6 KiB
Go
102 lines
2.6 KiB
Go
package sync
|
|
|
|
import (
|
|
"context"
|
|
"sync"
|
|
|
|
"github.com/libp2p/go-libp2p-core/peer"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/blockchain"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/db"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/operations"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/p2p"
|
|
pb "github.com/prysmaticlabs/prysm/proto/beacon/p2p/v1"
|
|
"github.com/prysmaticlabs/prysm/shared"
|
|
)
|
|
|
|
var _ = shared.Service(&RegularSync{})
|
|
|
|
// Config to set up the regular sync service.
|
|
type Config struct {
|
|
P2P p2p.P2P
|
|
DB db.Database
|
|
Operations *operations.Service
|
|
Chain blockchainService
|
|
}
|
|
|
|
// This defines the interface for interacting with block chain service
|
|
type blockchainService interface {
|
|
blockchain.BlockReceiver
|
|
blockchain.HeadFetcher
|
|
blockchain.FinalizationFetcher
|
|
blockchain.AttestationReceiver
|
|
}
|
|
|
|
// NewRegularSync service.
|
|
func NewRegularSync(cfg *Config) *RegularSync {
|
|
r := &RegularSync{
|
|
ctx: context.Background(),
|
|
db: cfg.DB,
|
|
p2p: cfg.P2P,
|
|
operations: cfg.Operations,
|
|
chain: cfg.Chain,
|
|
helloTracker: make(map[peer.ID]*pb.Hello),
|
|
}
|
|
|
|
r.registerRPCHandlers()
|
|
r.registerSubscribers()
|
|
|
|
return r
|
|
}
|
|
|
|
// RegularSync service is responsible for handling all run time p2p related operations as the
|
|
// main entry point for network messages.
|
|
type RegularSync struct {
|
|
ctx context.Context
|
|
p2p p2p.P2P
|
|
db db.Database
|
|
operations *operations.Service
|
|
chain blockchainService
|
|
helloTracker map[peer.ID]*pb.Hello
|
|
helloTrackerLock sync.RWMutex
|
|
}
|
|
|
|
// Start the regular sync service.
|
|
func (r *RegularSync) Start() {
|
|
r.p2p.AddConnectionHandler(r.sendRPCHelloRequest)
|
|
}
|
|
|
|
// Stop the regular sync service.
|
|
func (r *RegularSync) Stop() error {
|
|
return nil
|
|
}
|
|
|
|
// Status of the currently running regular sync service.
|
|
func (r *RegularSync) Status() error {
|
|
return nil
|
|
}
|
|
|
|
// Syncing returns true if the node is currently syncing with the network.
|
|
func (r *RegularSync) Syncing() bool {
|
|
// TODO(3147): Use real value.
|
|
return false
|
|
}
|
|
|
|
// Hellos returns the map of hello messages received so far.
|
|
func (r *RegularSync) Hellos() map[peer.ID]*pb.Hello {
|
|
r.helloTrackerLock.RLock()
|
|
defer r.helloTrackerLock.RUnlock()
|
|
return r.helloTracker
|
|
}
|
|
|
|
// Checker defines a struct which can verify whether a node is currently
|
|
// synchronizing a chain with the rest of peers in the network.
|
|
type Checker interface {
|
|
Syncing() bool
|
|
Status() error
|
|
}
|
|
|
|
// HelloTracker interface for accessing the hello / handshake messages received so far.
|
|
type HelloTracker interface {
|
|
Hellos() map[peer.ID]*pb.Hello
|
|
}
|