mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-22 19:40:37 +00:00
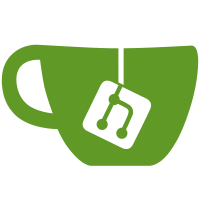
* Begin working on end to end tests using geth dev chain * Start on beacon node set up * More progress on bnode setup * Complete flow until chainstart, begin work on evaluators * More progress on evaluators * Start changing bazel run to direct binary * Move endtoend to inside beacon-chain * use bazel provided geth, use bazel test * tempdir * use fork rules_go * Change to use UUID dir and bazel binaries * Truncate UUID a bit * Get full run from chainstart to evaluating * Rewrite to react to logs rather than arbitrarily wait * Fix export * Move evaluators to evaluators.go * Add peer check test * Add more comments * Remove unneeded exports * Check all nodes have the correct amount of peers * Change name to onGenesisEpoch * Remove extra wait times where not needed * Cleanup * Add log for beacon start * Fix deposit amount * Make room for eth1follow distnce * Cleanup and fix minimal test * Goimports * Fix imports * gazelle and minimal * manual * Fix for comments * Make timing rely on reading logs, and cleanup * Fix for comments * Fix workspace * Cleanup * Fix visibility * Cleanup and some comments * Address comments * Fix for v0.9 * Modify for v0.9 * Move to own package outside of beacon-chain * Gazelle * Polishing, logging * Fix filenames * Add more logs * Add flag logging * Cover for page not having libp2p info * Improve multiAddr detection * Add more logs * Add missing flags * Add log printing to defer * Get multiAddr from logs * Fix logging and detection * Change evaluators to rely on EpochTimer * Add evaluator for ValidatorParticipation * Fix validator participation evaluator * Cleanup, comments and fix participation calculation * Cleanup * Let the file searcher search for longer * Change participation to check for full * Log out file contents if text isnt found * Split into different files * Disable IPC and use RPC instead, change tmp dir to bazel dir * Change visibility * Gazelle * Add e2e tag * new line
80 lines
1.9 KiB
Go
80 lines
1.9 KiB
Go
package endtoend
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/prysmaticlabs/prysm/shared/roughtime"
|
|
)
|
|
|
|
// EpochTicker is a special ticker for timing epoch changes.
|
|
// The channel emits over the epoch interval, and ensures that
|
|
// the ticks are in line with the genesis time. This means that
|
|
// the duration between the ticks and the genesis time are always a
|
|
// multiple of the epoch duration.
|
|
// In addition, the channel returns the new epoch number.
|
|
type EpochTicker struct {
|
|
c chan uint64
|
|
done chan struct{}
|
|
}
|
|
|
|
// C returns the ticker channel. Call Cancel afterwards to ensure
|
|
// that the goroutine exits cleanly.
|
|
func (s *EpochTicker) C() <-chan uint64 {
|
|
return s.c
|
|
}
|
|
|
|
// Done should be called to clean up the ticker.
|
|
func (s *EpochTicker) Done() {
|
|
go func() {
|
|
s.done <- struct{}{}
|
|
}()
|
|
}
|
|
|
|
// GetEpochTicker is the constructor for EpochTicker.
|
|
func GetEpochTicker(genesisTime time.Time, secondsPerEpoch uint64) *EpochTicker {
|
|
ticker := &EpochTicker{
|
|
c: make(chan uint64),
|
|
done: make(chan struct{}),
|
|
}
|
|
ticker.start(genesisTime, secondsPerEpoch, roughtime.Since, roughtime.Until, time.After)
|
|
return ticker
|
|
}
|
|
|
|
func (s *EpochTicker) start(
|
|
genesisTime time.Time,
|
|
secondsPerEpoch uint64,
|
|
since func(time.Time) time.Duration,
|
|
until func(time.Time) time.Duration,
|
|
after func(time.Duration) <-chan time.Time) {
|
|
|
|
d := time.Duration(secondsPerEpoch) * time.Second
|
|
|
|
go func() {
|
|
sinceGenesis := since(genesisTime)
|
|
|
|
var nextTickTime time.Time
|
|
var epoch uint64
|
|
if sinceGenesis < 0 {
|
|
// Handle when the current time is before the genesis time.
|
|
nextTickTime = genesisTime
|
|
epoch = 0
|
|
} else {
|
|
nextTick := sinceGenesis.Truncate(d) + d
|
|
nextTickTime = genesisTime.Add(nextTick)
|
|
epoch = uint64(nextTick / d)
|
|
}
|
|
|
|
for {
|
|
waitTime := until(nextTickTime)
|
|
select {
|
|
case <-after(waitTime):
|
|
s.c <- epoch
|
|
epoch++
|
|
nextTickTime = nextTickTime.Add(d)
|
|
case <-s.done:
|
|
return
|
|
}
|
|
}
|
|
}()
|
|
}
|