mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2025-01-10 19:51:20 +00:00
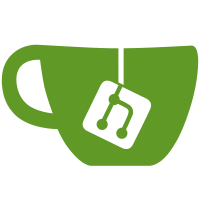
* Use ValidtorIndex across Prysm. Build ok * First take at fixing tests * Clean up e2e, fuzz... etc * Fix new lines * Update beacon-chain/cache/proposer_indices_test.go Co-authored-by: Raul Jordan <raul@prysmaticlabs.com> * Update beacon-chain/core/helpers/rewards_penalties.go Co-authored-by: Raul Jordan <raul@prysmaticlabs.com> * Update beacon-chain/core/helpers/shuffle.go Co-authored-by: Raul Jordan <raul@prysmaticlabs.com> * Update validator/graffiti/parse_graffiti_test.go Co-authored-by: Raul Jordan <raul@prysmaticlabs.com> * Raul's feedback * Fix downcast int -> uint64 * Victor's feedback Co-authored-by: Raul Jordan <raul@prysmaticlabs.com> Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com>
55 lines
1.8 KiB
Go
55 lines
1.8 KiB
Go
package kv
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/pkg/errors"
|
|
types "github.com/prysmaticlabs/eth2-types"
|
|
"github.com/prysmaticlabs/prysm/shared/bytesutil"
|
|
bolt "go.etcd.io/bbolt"
|
|
"go.opencensus.io/trace"
|
|
)
|
|
|
|
// ValidatorPubKey accepts validator id and returns the corresponding pubkey.
|
|
// Returns nil if the pubkey for this validator id does not exist.
|
|
func (s *Store) ValidatorPubKey(ctx context.Context, validatorID types.ValidatorIndex) ([]byte, error) {
|
|
ctx, span := trace.StartSpan(ctx, "SlasherDB.ValidatorPubKey")
|
|
defer span.End()
|
|
var pk []byte
|
|
err := s.view(func(tx *bolt.Tx) error {
|
|
b := tx.Bucket(validatorsPublicKeysBucket)
|
|
pk = b.Get(bytesutil.Bytes4(uint64(validatorID)))
|
|
return nil
|
|
})
|
|
return pk, err
|
|
}
|
|
|
|
// SavePubKey accepts a validator id and its public key and writes it to disk.
|
|
func (s *Store) SavePubKey(ctx context.Context, validatorID types.ValidatorIndex, pubKey []byte) error {
|
|
ctx, span := trace.StartSpan(ctx, "SlasherDB.SavePubKey")
|
|
defer span.End()
|
|
err := s.update(func(tx *bolt.Tx) error {
|
|
bucket := tx.Bucket(validatorsPublicKeysBucket)
|
|
key := bytesutil.Bytes4(uint64(validatorID))
|
|
if err := bucket.Put(key, pubKey); err != nil {
|
|
return errors.Wrap(err, "failed to add validator public key to slasher s.")
|
|
}
|
|
return nil
|
|
})
|
|
return err
|
|
}
|
|
|
|
// DeletePubKey deletes a public key of a validator id.
|
|
func (s *Store) DeletePubKey(ctx context.Context, validatorID types.ValidatorIndex) error {
|
|
ctx, span := trace.StartSpan(ctx, "SlasherDB.DeletePubKey")
|
|
defer span.End()
|
|
return s.update(func(tx *bolt.Tx) error {
|
|
bucket := tx.Bucket(validatorsPublicKeysBucket)
|
|
key := bytesutil.Bytes4(uint64(validatorID))
|
|
if err := bucket.Delete(key); err != nil {
|
|
return errors.Wrap(err, "failed to delete public key from validators public key bucket")
|
|
}
|
|
return bucket.Delete(key)
|
|
})
|
|
}
|