mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2025-01-04 00:44:27 +00:00
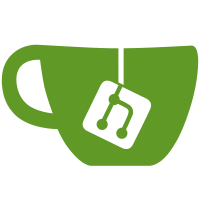
* Flat spanner improvements * Fix tests, add more to ES type * Move types to detection/types * Fix * Fix comments * Fixes * Use SlotTickerWithOffset for StreamIndexedAttestations (#5999) * Change streamindexed to slot ticker with offset * Make 2/3rds * Add test * Merge branch 'master' of github.com:prysmaticlabs/prysm into ticker-offset * Add check for offset * Merge branch 'master' of github.com:prysmaticlabs/prysm into ticker-offset * Fix test * Merge refs/heads/master into ticker-offset * Fix long running E2E after v0.12 changes (#6008) * Fix deposits in long run e2e * Fix participation Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com> * Small fixes for slasher (#6021) * Small fixes for slasher * Remove useless log * Fix test Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com> * Add voluntary exit processing to E2E (#6016) * Add voluntary exit to E2E * Fix long urnning e2e * Fix for comments Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com> * Change slashing pool to use spec code (#6030) * Change slashing pool to use more spec code * Fix test * Undo unneeded changes * Make sure to catch regression Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com> * Fix long-running E2E (#6047) * Fixes for long running E2E * Merge branch 'master' of github.com:prysmaticlabs/prysm into fix-e2e * Move metrics check up * Merge refs/heads/master into fix-e2e * E2E Improvements (#6091) * Some fixes * Merge branch 'master' into e2e-fixes * Add another small delay * Merge branch 'e2e-fixes' of github.com:prysmaticlabs/prysm into e2e-fixes * Remove genesis test, make normal e2e run longer * Gaz * more fixes * Merge branch 'master' into e2e-fixes * Merge refs/heads/master into e2e-fixes * Fix comment * Merge refs/heads/master into e2e-fixes * Begin changing tests * Start work on tests * Redo tests for EpochStore * Add test for highest index * Fixes * Gaz * Fix Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com>
47 lines
1.8 KiB
Go
47 lines
1.8 KiB
Go
package kv
|
|
|
|
import (
|
|
"github.com/prysmaticlabs/prysm/shared/bytesutil"
|
|
"github.com/prysmaticlabs/prysm/slasher/db/types"
|
|
)
|
|
|
|
const (
|
|
latestEpochKey = "LATEST_EPOCH_DETECTED"
|
|
chainHeadKey = "CHAIN_HEAD"
|
|
)
|
|
|
|
var (
|
|
indexedAttestationsRootsByTargetBucket = []byte("indexed-attestations-roots-by-target")
|
|
indexedAttestationsBucket = []byte("indexed-attestations")
|
|
// Slasher-related buckets.
|
|
historicIndexedAttestationsBucket = []byte("historic-indexed-attestations-bucket")
|
|
historicBlockHeadersBucket = []byte("historic-block-headers-bucket")
|
|
slashingBucket = []byte("slashing-bucket")
|
|
chainDataBucket = []byte("chain-data-bucket")
|
|
compressedIdxAttsBucket = []byte("compressed-idx-atts-bucket")
|
|
validatorsPublicKeysBucket = []byte("validators-public-keys-bucket")
|
|
// In order to quickly detect surround and surrounded attestations we need to store
|
|
// the min and max span for each validator for each epoch.
|
|
// see https://github.com/protolambda/eth2-surround/blob/master/README.md#min-max-surround
|
|
validatorsMinMaxSpanBucket = []byte("validators-min-max-span-bucket")
|
|
validatorsMinMaxSpanBucketNew = []byte("validators-min-max-span-bucket-new")
|
|
)
|
|
|
|
func encodeSlotValidatorID(slot uint64, validatorID uint64) []byte {
|
|
return append(bytesutil.Bytes8(slot), bytesutil.Bytes8(validatorID)...)
|
|
}
|
|
|
|
func encodeSlotValidatorIDSig(slot uint64, validatorID uint64, sig []byte) []byte {
|
|
return append(append(bytesutil.Bytes8(slot), bytesutil.Bytes8(validatorID)...), sig...)
|
|
}
|
|
|
|
func encodeEpochSig(targetEpoch uint64, sig []byte) []byte {
|
|
return append(bytesutil.Bytes8(targetEpoch), sig...)
|
|
}
|
|
func encodeType(st types.SlashingType) []byte {
|
|
return []byte{byte(st)}
|
|
}
|
|
func encodeTypeRoot(st types.SlashingType, root [32]byte) []byte {
|
|
return append([]byte{byte(st)}, root[:]...)
|
|
}
|