mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2025-01-08 10:41:19 +00:00
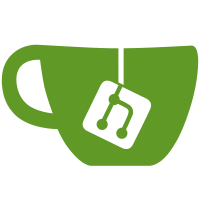
* Show error in logs if passing invalid flags in yaml The YAML configs pass if an invalid flag is set, this causes frustration because the flag could have been mispelled causing a huge mystery. This will strictly check if the flags are deserialized, if a flag doesn't exist, it will error out as a Fatal error with appropriate reasoning which flag is wrong. * Fix review comments to make some of them non-fatal * Make yaml.TypeError a pointer * Remove UnmarshalStrict from spectests since they use a different YAML package Co-authored-by: Preston Van Loon <preston@prysmaticlabs.com>
127 lines
3.5 KiB
Go
127 lines
3.5 KiB
Go
package params
|
|
|
|
import (
|
|
"encoding/hex"
|
|
"io/ioutil"
|
|
"strings"
|
|
|
|
log "github.com/sirupsen/logrus"
|
|
"gopkg.in/yaml.v2"
|
|
)
|
|
|
|
// LoadChainConfigFile load, convert hex values into valid param yaml format,
|
|
// unmarshal , and apply beacon chain config file.
|
|
func LoadChainConfigFile(chainConfigFileName string) {
|
|
yamlFile, err := ioutil.ReadFile(chainConfigFileName)
|
|
if err != nil {
|
|
log.WithError(err).Fatal("Failed to read chain config file.")
|
|
}
|
|
// Convert 0x hex inputs to fixed bytes arrays
|
|
lines := strings.Split(string(yamlFile), "\n")
|
|
for i, line := range lines {
|
|
// No need to convert the deposit contract address to byte array (as config expects a string).
|
|
if strings.HasPrefix(line, "DEPOSIT_CONTRACT_ADDRESS") {
|
|
continue
|
|
}
|
|
if !strings.HasPrefix(line, "#") && strings.Contains(line, "0x") {
|
|
parts := replaceHexStringWithYAMLFormat(line)
|
|
lines[i] = strings.Join(parts, "\n")
|
|
}
|
|
}
|
|
yamlFile = []byte(strings.Join(lines, "\n"))
|
|
conf := MainnetConfig()
|
|
if err := yaml.UnmarshalStrict(yamlFile, conf); err != nil {
|
|
if _, ok := err.(*yaml.TypeError); !ok {
|
|
log.WithError(err).Fatal("Failed to parse chain config yaml file.")
|
|
} else {
|
|
log.WithError(err).Error("There were some issues parsing the config from a yaml file")
|
|
}
|
|
}
|
|
log.Debugf("Config file values: %+v", conf)
|
|
OverrideBeaconConfig(conf)
|
|
}
|
|
|
|
func replaceHexStringWithYAMLFormat(line string) []string {
|
|
parts := strings.Split(line, "0x")
|
|
decoded, err := hex.DecodeString(parts[1])
|
|
if err != nil {
|
|
log.WithError(err).Error("Failed to decode hex string.")
|
|
}
|
|
switch l := len(decoded); {
|
|
case l == 1:
|
|
var b byte
|
|
b = decoded[0]
|
|
fixedByte, err := yaml.Marshal(b)
|
|
if err != nil {
|
|
log.WithError(err).Error("Failed to marshal config file.")
|
|
}
|
|
parts[0] += string(fixedByte)
|
|
parts = parts[:1]
|
|
case l > 1 && l <= 4:
|
|
var arr [4]byte
|
|
copy(arr[:], decoded)
|
|
fixedByte, err := yaml.Marshal(arr)
|
|
if err != nil {
|
|
log.WithError(err).Error("Failed to marshal config file.")
|
|
}
|
|
parts[1] = string(fixedByte)
|
|
case l > 4 && l <= 8:
|
|
var arr [8]byte
|
|
copy(arr[:], decoded)
|
|
fixedByte, err := yaml.Marshal(arr)
|
|
if err != nil {
|
|
log.WithError(err).Error("Failed to marshal config file.")
|
|
}
|
|
parts[1] = string(fixedByte)
|
|
case l > 8 && l <= 16:
|
|
var arr [16]byte
|
|
copy(arr[:], decoded)
|
|
fixedByte, err := yaml.Marshal(arr)
|
|
if err != nil {
|
|
log.WithError(err).Error("Failed to marshal config file.")
|
|
}
|
|
parts[1] = string(fixedByte)
|
|
case l > 16 && l <= 20:
|
|
var arr [20]byte
|
|
copy(arr[:], decoded)
|
|
fixedByte, err := yaml.Marshal(arr)
|
|
if err != nil {
|
|
log.WithError(err).Error("Failed to marshal config file.")
|
|
}
|
|
parts[1] = string(fixedByte)
|
|
case l > 20 && l <= 32:
|
|
var arr [32]byte
|
|
copy(arr[:], decoded)
|
|
fixedByte, err := yaml.Marshal(arr)
|
|
if err != nil {
|
|
log.WithError(err).Error("Failed to marshal config file.")
|
|
}
|
|
parts[1] = string(fixedByte)
|
|
case l > 32 && l <= 48:
|
|
var arr [48]byte
|
|
copy(arr[:], decoded)
|
|
fixedByte, err := yaml.Marshal(arr)
|
|
if err != nil {
|
|
log.WithError(err).Error("Failed to marshal config file.")
|
|
}
|
|
parts[1] = string(fixedByte)
|
|
case l > 48 && l <= 64:
|
|
var arr [64]byte
|
|
copy(arr[:], decoded)
|
|
fixedByte, err := yaml.Marshal(arr)
|
|
if err != nil {
|
|
log.WithError(err).Error("Failed to marshal config file.")
|
|
}
|
|
parts[1] = string(fixedByte)
|
|
case l > 64 && l <= 96:
|
|
var arr [96]byte
|
|
copy(arr[:], decoded)
|
|
fixedByte, err := yaml.Marshal(arr)
|
|
if err != nil {
|
|
log.WithError(err).Error("Failed to marshal config file.")
|
|
}
|
|
parts[1] = string(fixedByte)
|
|
}
|
|
return parts
|
|
}
|