mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 12:57:18 +00:00
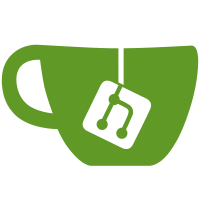
* init * bellatrix + altair tests passing * Add Phase0 support * add feature flag * phase0 test * restore testvectors * mod tidy * state tests * gaz * do not call precompute * fix test * Fix context * move to own's method * remove spectests pulltips * time import * remove phase0 * mod tidy * fix getters * Update beacon-chain/forkchoice/doubly-linked-tree/types.go * reviews * fix workspace * Recursive rlocks Co-authored-by: terencechain <terence@prysmaticlabs.com> Co-authored-by: Radosław Kapka <rkapka@wp.pl>
49 lines
1.7 KiB
Go
49 lines
1.7 KiB
Go
package stateutil
|
|
|
|
import (
|
|
"github.com/pkg/errors"
|
|
"github.com/prysmaticlabs/prysm/config/params"
|
|
types "github.com/prysmaticlabs/prysm/consensus-types/primitives"
|
|
"github.com/prysmaticlabs/prysm/math"
|
|
ethpb "github.com/prysmaticlabs/prysm/proto/prysm/v1alpha1"
|
|
)
|
|
|
|
// UnrealizedCheckpointBalances returns the total current active balance, the
|
|
// total previous epoch correctly attested for target balance, and the total
|
|
// current epoch correctly attested for target balance. It takes the current and
|
|
// previous epoch participation bits as parameters so implicitly only works for
|
|
// beacon states post-Altair.
|
|
func UnrealizedCheckpointBalances(cp, pp []byte, validators []*ethpb.Validator, currentEpoch types.Epoch) (uint64, uint64, uint64, error) {
|
|
targetIdx := params.BeaconConfig().TimelyTargetFlagIndex
|
|
activeBalance := uint64(0)
|
|
currentTarget := uint64(0)
|
|
prevTarget := uint64(0)
|
|
if len(cp) < len(validators) || len(pp) < len(validators) {
|
|
return 0, 0, 0, errors.New("participation does not match validator set")
|
|
}
|
|
|
|
var err error
|
|
for i, v := range validators {
|
|
active := v.ActivationEpoch <= currentEpoch && currentEpoch < v.ExitEpoch
|
|
if active && !v.Slashed {
|
|
activeBalance, err = math.Add64(activeBalance, v.EffectiveBalance)
|
|
if err != nil {
|
|
return 0, 0, 0, err
|
|
}
|
|
if ((cp[i] >> targetIdx) & 1) == 1 {
|
|
currentTarget, err = math.Add64(currentTarget, v.EffectiveBalance)
|
|
if err != nil {
|
|
return 0, 0, 0, err
|
|
}
|
|
}
|
|
if ((pp[i] >> targetIdx) & 1) == 1 {
|
|
prevTarget, err = math.Add64(prevTarget, v.EffectiveBalance)
|
|
if err != nil {
|
|
return 0, 0, 0, err
|
|
}
|
|
}
|
|
}
|
|
}
|
|
return activeBalance, prevTarget, currentTarget, nil
|
|
}
|