mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
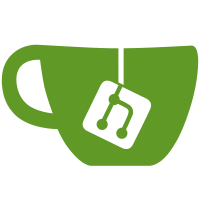
* Begin adding DB to validator client Begin adding ValidatorProposalHistory Implement most of proposal history Finish tests Fix marking a proposal for the first time Change proposalhistory to not using bit shifting Add pb.go Change after proto/slashing added Finally fix protos Fix most tests Fix all tests for double proposal protection Start initialiing DB in validator client Add db to validator struct Add DB to ProposeBlock Fix test errors and begin mocking Fix test formatting and pass test for validator protection! Fix merge issues Fix renames Fix tests * Fix tests * Fix first startup on DB * Fix nil check tests * Fix E2E * Fix e2e flag * Fix comments * Fix for comments * Move proposal hepers to validator/client to keep DB clean * Add clear-db flag to validator client * Fix formatting * Clear out unintended changes * Fix build issues * Fix build issues * Gazelle * Fix mock test * Remove proposal history * Add terminal confirmation to DB clearing * Add interface for validatorDB, add context to DB functions * Add force-clear-db flag * Cleanup * Update validator/node/node.go Co-Authored-By: Raul Jordan <raul@prysmaticlabs.com> * Change db to clear file, not whole folder * Fix db test * Fix teardown test Co-authored-by: Raul Jordan <raul@prysmaticlabs.com>
49 lines
1.1 KiB
Go
49 lines
1.1 KiB
Go
package db
|
|
|
|
import (
|
|
"crypto/rand"
|
|
"fmt"
|
|
"math/big"
|
|
"os"
|
|
"path/filepath"
|
|
"testing"
|
|
)
|
|
|
|
// SetupDB instantiates and returns a DB instance for the validator client.
|
|
func SetupDB(t testing.TB, pubkeys [][48]byte) *Store {
|
|
randPath, err := rand.Int(rand.Reader, big.NewInt(1000000))
|
|
if err != nil {
|
|
t.Fatalf("Could not generate random file path: %v", err)
|
|
}
|
|
p := filepath.Join(TempDir(), fmt.Sprintf("/%d", randPath))
|
|
if err := os.RemoveAll(p); err != nil {
|
|
t.Fatalf("Failed to remove directory: %v", err)
|
|
}
|
|
db, err := NewKVStore(p, pubkeys)
|
|
if err != nil {
|
|
t.Fatalf("Failed to instantiate DB: %v", err)
|
|
}
|
|
return db
|
|
}
|
|
|
|
// TempDir returns a directory path for temporary test storage.
|
|
func TempDir() string {
|
|
d := os.Getenv("TEST_TMPDIR")
|
|
|
|
// If the test is not run via bazel, the environment var won't be set.
|
|
if d == "" {
|
|
return os.TempDir()
|
|
}
|
|
return d
|
|
}
|
|
|
|
// TeardownDB cleans up a test DB instance.
|
|
func TeardownDB(t testing.TB, db *Store) {
|
|
if err := db.Close(); err != nil {
|
|
t.Fatalf("Failed to close database: %v", err)
|
|
}
|
|
if err := db.ClearDB(); err != nil {
|
|
t.Fatalf("Failed to clear database: %v", err)
|
|
}
|
|
}
|