mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-27 21:57:16 +00:00
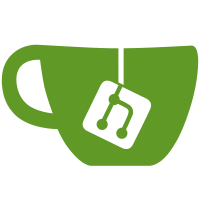
* begin reorder * move into beacon server * add proposer server * fix * add proposer server * wrap up rpc reorder * eliminated deprecated RPC endpoints * formatted nicely * RPC protos * fix lint * integrate hash proto * autoclean * deprecate all old code * include the rest of methods mocks * bazel run * lint fixes
97 lines
3.0 KiB
Go
97 lines
3.0 KiB
Go
package rpc
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
"testing"
|
|
|
|
ptypes "github.com/gogo/protobuf/types"
|
|
"github.com/golang/mock/gomock"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/internal"
|
|
pbp2p "github.com/prysmaticlabs/prysm/proto/beacon/p2p/v1"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil"
|
|
logTest "github.com/sirupsen/logrus/hooks/test"
|
|
)
|
|
|
|
func TestLatestAttestationContextClosed(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
mockAttestationService := &mockAttestationService{}
|
|
ctx, cancel := context.WithCancel(context.Background())
|
|
beaconServer := &BeaconServer{
|
|
ctx: ctx,
|
|
attestationService: mockAttestationService,
|
|
}
|
|
exitRoutine := make(chan bool)
|
|
ctrl := gomock.NewController(t)
|
|
defer ctrl.Finish()
|
|
mockStream := internal.NewMockBeaconService_LatestAttestationServer(ctrl)
|
|
go func(tt *testing.T) {
|
|
if err := beaconServer.LatestAttestation(&ptypes.Empty{}, mockStream); err != nil {
|
|
tt.Errorf("Could not call RPC method: %v", err)
|
|
}
|
|
<-exitRoutine
|
|
}(t)
|
|
cancel()
|
|
exitRoutine <- true
|
|
testutil.AssertLogsContain(t, hook, "RPC context closed, exiting goroutine")
|
|
}
|
|
|
|
func TestLatestAttestationFaulty(t *testing.T) {
|
|
attestationService := &mockAttestationService{}
|
|
ctx, cancel := context.WithCancel(context.Background())
|
|
beaconServer := &BeaconServer{
|
|
ctx: ctx,
|
|
attestationService: attestationService,
|
|
incomingAttestation: make(chan *pbp2p.Attestation, 0),
|
|
}
|
|
ctrl := gomock.NewController(t)
|
|
defer ctrl.Finish()
|
|
|
|
exitRoutine := make(chan bool)
|
|
attestation := &pbp2p.Attestation{}
|
|
|
|
mockStream := internal.NewMockBeaconService_LatestAttestationServer(ctrl)
|
|
mockStream.EXPECT().Send(attestation).Return(errors.New("something wrong"))
|
|
// Tests a faulty stream.
|
|
go func(tt *testing.T) {
|
|
if err := beaconServer.LatestAttestation(&ptypes.Empty{}, mockStream); err.Error() != "something wrong" {
|
|
tt.Errorf("Faulty stream should throw correct error, wanted 'something wrong', got %v", err)
|
|
}
|
|
<-exitRoutine
|
|
}(t)
|
|
|
|
beaconServer.incomingAttestation <- attestation
|
|
cancel()
|
|
exitRoutine <- true
|
|
}
|
|
|
|
func TestLatestAttestation(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
attestationService := &mockAttestationService{}
|
|
ctx, cancel := context.WithCancel(context.Background())
|
|
beaconServer := &BeaconServer{
|
|
ctx: ctx,
|
|
attestationService: attestationService,
|
|
incomingAttestation: make(chan *pbp2p.Attestation, 0),
|
|
}
|
|
ctrl := gomock.NewController(t)
|
|
defer ctrl.Finish()
|
|
|
|
exitRoutine := make(chan bool)
|
|
attestation := &pbp2p.Attestation{}
|
|
mockStream := internal.NewMockBeaconService_LatestAttestationServer(ctrl)
|
|
mockStream.EXPECT().Send(attestation).Return(nil)
|
|
// Tests a good stream.
|
|
go func(tt *testing.T) {
|
|
if err := beaconServer.LatestAttestation(&ptypes.Empty{}, mockStream); err != nil {
|
|
tt.Errorf("Could not call RPC method: %v", err)
|
|
}
|
|
<-exitRoutine
|
|
}(t)
|
|
beaconServer.incomingAttestation <- attestation
|
|
cancel()
|
|
exitRoutine <- true
|
|
|
|
testutil.AssertLogsContain(t, hook, "Sending attestation to RPC clients")
|
|
}
|