mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
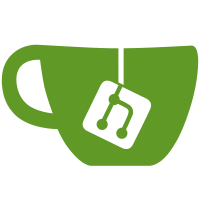
* transition proto * gen pb * builds * impl transition config * begin tests * transition config messed up * amend proto * use str * passing * gaz * config * client test * pb * set to 0 * rem log * gaz * check transition config * check config differences * check transition config in background * gaz * pass * redundant * fix up error handling and healthz * simplify status * gazelle * build * err config check * test * gaz * Fix run time Co-authored-by: terence tsao <terence@prysmaticlabs.com> Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com>
50 lines
1.4 KiB
Go
50 lines
1.4 KiB
Go
package blockchain
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/ethereum/go-ethereum/common"
|
|
"github.com/pkg/errors"
|
|
enginev1 "github.com/prysmaticlabs/prysm/proto/engine/v1"
|
|
)
|
|
|
|
type mockEngineService struct {
|
|
newPayloadError error
|
|
forkchoiceError error
|
|
blks map[[32]byte]*enginev1.ExecutionBlock
|
|
}
|
|
|
|
func (m *mockEngineService) NewPayload(context.Context, *enginev1.ExecutionPayload) ([]byte, error) {
|
|
return nil, m.newPayloadError
|
|
}
|
|
|
|
func (m *mockEngineService) ForkchoiceUpdated(context.Context, *enginev1.ForkchoiceState, *enginev1.PayloadAttributes) (*enginev1.PayloadIDBytes, []byte, error) {
|
|
return nil, nil, m.forkchoiceError
|
|
}
|
|
|
|
func (*mockEngineService) GetPayloadV1(
|
|
_ context.Context, _ enginev1.PayloadIDBytes,
|
|
) *enginev1.ExecutionPayload {
|
|
return nil
|
|
}
|
|
|
|
func (*mockEngineService) GetPayload(context.Context, [8]byte) (*enginev1.ExecutionPayload, error) {
|
|
return nil, nil
|
|
}
|
|
|
|
func (*mockEngineService) ExchangeTransitionConfiguration(context.Context, *enginev1.TransitionConfiguration) error {
|
|
return nil
|
|
}
|
|
|
|
func (*mockEngineService) LatestExecutionBlock(context.Context) (*enginev1.ExecutionBlock, error) {
|
|
return nil, nil
|
|
}
|
|
|
|
func (m *mockEngineService) ExecutionBlockByHash(_ context.Context, hash common.Hash) (*enginev1.ExecutionBlock, error) {
|
|
blk, ok := m.blks[common.BytesToHash(hash.Bytes())]
|
|
if !ok {
|
|
return nil, errors.New("block not found")
|
|
}
|
|
return blk, nil
|
|
}
|