mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-23 11:57:18 +00:00
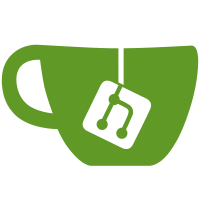
* replace eth2 types * replace protos * regen proto * replace * gaz * deps * amend * regen proto * mod * gaz * gaz * ensure build * ssz * add dep * no more eth2 types * no more eth2 * remg * all builds * buidl * tidy * clean * fmt * val serv * gaz Co-authored-by: Preston Van Loon <preston@prysmaticlabs.com>
62 lines
1.8 KiB
Go
62 lines
1.8 KiB
Go
package sync
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
|
|
"github.com/pkg/errors"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/cache"
|
|
"github.com/prysmaticlabs/prysm/config/params"
|
|
types "github.com/prysmaticlabs/prysm/consensus-types/primitives"
|
|
"github.com/prysmaticlabs/prysm/container/slice"
|
|
eth "github.com/prysmaticlabs/prysm/proto/prysm/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/time/slots"
|
|
"google.golang.org/protobuf/proto"
|
|
)
|
|
|
|
func (s *Service) committeeIndexBeaconAttestationSubscriber(_ context.Context, msg proto.Message) error {
|
|
a, ok := msg.(*eth.Attestation)
|
|
if !ok {
|
|
return fmt.Errorf("message was not type *eth.Attestation, type=%T", msg)
|
|
}
|
|
|
|
if a.Data == nil {
|
|
return errors.New("nil attestation")
|
|
}
|
|
s.setSeenCommitteeIndicesSlot(a.Data.Slot, a.Data.CommitteeIndex, a.AggregationBits)
|
|
|
|
exists, err := s.cfg.attPool.HasAggregatedAttestation(a)
|
|
if err != nil {
|
|
return errors.Wrap(err, "Could not determine if attestation pool has this atttestation")
|
|
}
|
|
if exists {
|
|
return nil
|
|
}
|
|
|
|
return s.cfg.attPool.SaveUnaggregatedAttestation(a)
|
|
}
|
|
|
|
func (_ *Service) persistentSubnetIndices() []uint64 {
|
|
return cache.SubnetIDs.GetAllSubnets()
|
|
}
|
|
|
|
func (_ *Service) aggregatorSubnetIndices(currentSlot types.Slot) []uint64 {
|
|
endEpoch := slots.ToEpoch(currentSlot) + 1
|
|
endSlot := params.BeaconConfig().SlotsPerEpoch.Mul(uint64(endEpoch))
|
|
var commIds []uint64
|
|
for i := currentSlot; i <= endSlot; i++ {
|
|
commIds = append(commIds, cache.SubnetIDs.GetAggregatorSubnetIDs(i)...)
|
|
}
|
|
return slice.SetUint64(commIds)
|
|
}
|
|
|
|
func (_ *Service) attesterSubnetIndices(currentSlot types.Slot) []uint64 {
|
|
endEpoch := slots.ToEpoch(currentSlot) + 1
|
|
endSlot := params.BeaconConfig().SlotsPerEpoch.Mul(uint64(endEpoch))
|
|
var commIds []uint64
|
|
for i := currentSlot; i <= endSlot; i++ {
|
|
commIds = append(commIds, cache.SubnetIDs.GetAttesterSubnetIDs(i)...)
|
|
}
|
|
return slice.SetUint64(commIds)
|
|
}
|