mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
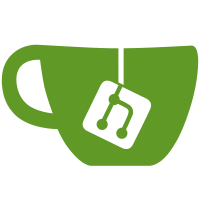
* slasher grpc client * do not export * slasher on a different package * fix featureconfig * change to rough time * revert roughtime * remove extra comma * revert order change * goimports * fix comments and tests * fix package name * revert reorder * comment for start * service * fix visibility * external slasher validator protection implementation * gaz * fix comment * add comments * nishant feedback * raul feedback * preston feedback * fix flags * fix imports * fix imports * port 4002 * added tests * fix log * fix imports * fix imports name * raul feedback * gaz * terence comment * change name * runtime fixes * add flag check Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com>
40 lines
1.0 KiB
Go
40 lines
1.0 KiB
Go
package testing
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"strconv"
|
|
|
|
pb "github.com/prysmaticlabs/prysm/proto/beacon/p2p/v1"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil"
|
|
)
|
|
|
|
const fileBase = "0-11-0/mainnet/beaconstate"
|
|
const fileBaseENV = "BEACONSTATES_PATH"
|
|
|
|
// GetBeaconFuzzState returns a beacon state by ID using the beacon-fuzz corpora.
|
|
func GetBeaconFuzzState(ID uint16) (*pb.BeaconState, error) {
|
|
base := fileBase
|
|
// Using an environment variable allows a host image to specify the path when only the binary
|
|
// executable was uploaded (without the runfiles). i.e. fuzzit's platform.
|
|
if p, ok := os.LookupEnv(fileBaseENV); ok {
|
|
base = p
|
|
}
|
|
ok, err := testutil.BazelDirectoryNonEmpty(base)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
if !ok {
|
|
panic(fmt.Sprintf("Beacon states directory (%s) does not exist or has no files.", base))
|
|
}
|
|
b, err := testutil.BazelFileBytes(base, strconv.Itoa(int(ID)))
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
st := &pb.BeaconState{}
|
|
if err := st.UnmarshalSSZ(b); err != nil {
|
|
return nil, err
|
|
}
|
|
return st, nil
|
|
}
|