mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
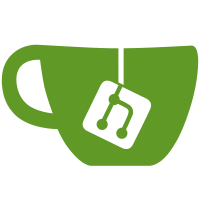
* Unify `GenesisValidator(s)Root` throughout the codebase * comments and literals Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com> Co-authored-by: terence tsao <terence@prysmaticlabs.com>
59 lines
1.9 KiB
Go
59 lines
1.9 KiB
Go
package validator
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/core/blocks"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/core/feed"
|
|
opfeed "github.com/prysmaticlabs/prysm/beacon-chain/core/feed/operation"
|
|
fieldparams "github.com/prysmaticlabs/prysm/config/fieldparams"
|
|
ethpb "github.com/prysmaticlabs/prysm/proto/prysm/v1alpha1"
|
|
"google.golang.org/grpc/codes"
|
|
"google.golang.org/grpc/status"
|
|
)
|
|
|
|
// ProposeExit proposes an exit for a validator.
|
|
func (vs *Server) ProposeExit(ctx context.Context, req *ethpb.SignedVoluntaryExit) (*ethpb.ProposeExitResponse, error) {
|
|
if req == nil {
|
|
return nil, status.Error(codes.InvalidArgument, "nil request")
|
|
}
|
|
s, err := vs.HeadFetcher.HeadState(ctx)
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not get head state: %v", err)
|
|
}
|
|
if req.Exit == nil {
|
|
return nil, status.Error(codes.InvalidArgument, "voluntary exit does not exist")
|
|
}
|
|
if req.Signature == nil || len(req.Signature) != fieldparams.BLSSignatureLength {
|
|
return nil, status.Error(codes.InvalidArgument, "invalid signature provided")
|
|
}
|
|
|
|
// Confirm the validator is eligible to exit with the parameters provided.
|
|
val, err := s.ValidatorAtIndexReadOnly(req.Exit.ValidatorIndex)
|
|
if err != nil {
|
|
return nil, status.Error(codes.InvalidArgument, "validator index exceeds validator set length")
|
|
}
|
|
|
|
if err := blocks.VerifyExitAndSignature(val, s.Slot(), s.Fork(), req, s.GenesisValidatorsRoot()); err != nil {
|
|
return nil, status.Error(codes.InvalidArgument, err.Error())
|
|
}
|
|
|
|
vs.OperationNotifier.OperationFeed().Send(&feed.Event{
|
|
Type: opfeed.ExitReceived,
|
|
Data: &opfeed.ExitReceivedData{
|
|
Exit: req,
|
|
},
|
|
})
|
|
|
|
vs.ExitPool.InsertVoluntaryExit(ctx, s, req)
|
|
|
|
r, err := req.Exit.HashTreeRoot()
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not get tree hash of exit: %v", err)
|
|
}
|
|
|
|
return ðpb.ProposeExitResponse{
|
|
ExitRoot: r[:],
|
|
}, vs.P2P.Broadcast(ctx, req)
|
|
}
|