mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
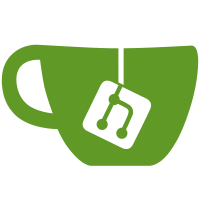
* remove unused code * remove defer use in loop * Remove unused methods and constants * gofmt and gaz * nilness check * remove unused args * Add TODO for refactoring subscribeWithBase to remove unused arg. It seems too involved to include in this sweeping PR. https://github.com/prysmaticlabs/prysm/issues/7437 * replace empty slice declaration * Remove unnecessary type conversions * remove redundant type declaration * rename receivers to be consistent * Remove bootnode query tool. It is now obsolete by discv5 * Remove relay node. It is no longer used or supported * Revert "Remove relay node. It is no longer used or supported" This reverts commit 4bd7717334dad85ef4766ed9bc4da711fb5fa810. * Delete unused test directory * Delete unsupported gcp startup script * Delete old k8s script * build fixes * fix build * go mod tidy * revert slasher/db/kv/block_header.go * fix build * remove redundant nil check * combine func args Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com> Co-authored-by: Victor Farazdagi <simple.square@gmail.com>
140 lines
6.0 KiB
Go
140 lines
6.0 KiB
Go
package validator
|
|
|
|
import (
|
|
"bytes"
|
|
"context"
|
|
|
|
ethpb "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/core/helpers"
|
|
"github.com/prysmaticlabs/prysm/shared/bytesutil"
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
"github.com/sirupsen/logrus"
|
|
"go.opencensus.io/trace"
|
|
"google.golang.org/grpc/codes"
|
|
"google.golang.org/grpc/status"
|
|
)
|
|
|
|
// SubmitAggregateSelectionProof is called by a validator when its assigned to be an aggregator.
|
|
// The aggregator submits the selection proof to obtain the aggregated attestation
|
|
// object to sign over.
|
|
func (vs *Server) SubmitAggregateSelectionProof(ctx context.Context, req *ethpb.AggregateSelectionRequest) (*ethpb.AggregateSelectionResponse, error) {
|
|
ctx, span := trace.StartSpan(ctx, "AggregatorServer.SubmitAggregateSelectionProof")
|
|
defer span.End()
|
|
span.AddAttributes(trace.Int64Attribute("slot", int64(req.Slot)))
|
|
|
|
if vs.SyncChecker.Syncing() {
|
|
return nil, status.Errorf(codes.Unavailable, "Syncing to latest head, not ready to respond")
|
|
}
|
|
|
|
st, err := vs.HeadFetcher.HeadState(ctx)
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not determine head state: %v", err)
|
|
}
|
|
|
|
validatorIndex, exists := st.ValidatorIndexByPubkey(bytesutil.ToBytes48(req.PublicKey))
|
|
if !exists {
|
|
return nil, status.Error(codes.Internal, "Could not locate validator index in DB")
|
|
}
|
|
|
|
epoch := helpers.SlotToEpoch(req.Slot)
|
|
activeValidatorIndices, err := helpers.ActiveValidatorIndices(st, epoch)
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not get validators: %v", err)
|
|
}
|
|
seed, err := helpers.Seed(st, epoch, params.BeaconConfig().DomainBeaconAttester)
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not get seed: %v", err)
|
|
}
|
|
committee, err := helpers.BeaconCommittee(activeValidatorIndices, seed, req.Slot, req.CommitteeIndex)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
// Check if the validator is an aggregator
|
|
isAggregator, err := helpers.IsAggregator(uint64(len(committee)), req.SlotSignature)
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not get aggregator status: %v", err)
|
|
}
|
|
if !isAggregator {
|
|
return nil, status.Errorf(codes.InvalidArgument, "Validator is not an aggregator")
|
|
}
|
|
|
|
if err := vs.AttPool.AggregateUnaggregatedAttestationsBySlotIndex(req.Slot, req.CommitteeIndex); err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not aggregate unaggregated attestations")
|
|
}
|
|
aggregatedAtts := vs.AttPool.AggregatedAttestationsBySlotIndex(req.Slot, req.CommitteeIndex)
|
|
|
|
// Filter out the best aggregated attestation (ie. the one with the most aggregated bits).
|
|
if len(aggregatedAtts) == 0 {
|
|
aggregatedAtts = vs.AttPool.UnaggregatedAttestationsBySlotIndex(req.Slot, req.CommitteeIndex)
|
|
if len(aggregatedAtts) == 0 {
|
|
return nil, status.Errorf(codes.Internal, "Could not find attestation for slot and committee in pool")
|
|
}
|
|
}
|
|
|
|
var indexInCommittee uint64
|
|
for i, idx := range committee {
|
|
if idx == validatorIndex {
|
|
indexInCommittee = uint64(i)
|
|
}
|
|
}
|
|
|
|
best := aggregatedAtts[0]
|
|
for _, aggregatedAtt := range aggregatedAtts[1:] {
|
|
// The aggregator should prefer an attestation that they have signed. We check this by
|
|
// looking at the attestation's committee index against the validator's committee index
|
|
// and check the aggregate bits to ensure the validator's index is set.
|
|
if aggregatedAtt.Data.CommitteeIndex == req.CommitteeIndex &&
|
|
aggregatedAtt.AggregationBits.BitAt(indexInCommittee) &&
|
|
(!best.AggregationBits.BitAt(indexInCommittee) ||
|
|
aggregatedAtt.AggregationBits.Count() > best.AggregationBits.Count()) {
|
|
best = aggregatedAtt
|
|
}
|
|
|
|
// If the "best" still doesn't contain the validator's index, check the aggregation bits to
|
|
// choose the attestation with the most bits set.
|
|
if !best.AggregationBits.BitAt(indexInCommittee) &&
|
|
aggregatedAtt.AggregationBits.Count() > best.AggregationBits.Count() {
|
|
best = aggregatedAtt
|
|
}
|
|
}
|
|
a := ðpb.AggregateAttestationAndProof{
|
|
Aggregate: best,
|
|
SelectionProof: req.SlotSignature,
|
|
AggregatorIndex: validatorIndex,
|
|
}
|
|
return ðpb.AggregateSelectionResponse{AggregateAndProof: a}, nil
|
|
}
|
|
|
|
// SubmitSignedAggregateSelectionProof is called by a validator to broadcast a signed
|
|
// aggregated and proof object.
|
|
func (vs *Server) SubmitSignedAggregateSelectionProof(ctx context.Context, req *ethpb.SignedAggregateSubmitRequest) (*ethpb.SignedAggregateSubmitResponse, error) {
|
|
if req.SignedAggregateAndProof == nil || req.SignedAggregateAndProof.Message == nil ||
|
|
req.SignedAggregateAndProof.Message.Aggregate == nil || req.SignedAggregateAndProof.Message.Aggregate.Data == nil {
|
|
return nil, status.Error(codes.InvalidArgument, "Signed aggregate request can't be nil")
|
|
}
|
|
emptySig := make([]byte, params.BeaconConfig().BLSSignatureLength)
|
|
if bytes.Equal(req.SignedAggregateAndProof.Signature, emptySig) ||
|
|
bytes.Equal(req.SignedAggregateAndProof.Message.SelectionProof, emptySig) {
|
|
return nil, status.Error(codes.InvalidArgument, "Signed signatures can't be zero hashes")
|
|
}
|
|
|
|
// As a preventive measure, a beacon node shouldn't broadcast an attestation whose slot is out of range.
|
|
if err := helpers.ValidateAttestationTime(req.SignedAggregateAndProof.Message.Aggregate.Data.Slot, vs.GenesisTimeFetcher.GenesisTime()); err != nil {
|
|
return nil, status.Error(codes.InvalidArgument, "Attestation slot is no longer valid from current time")
|
|
}
|
|
|
|
if err := vs.P2P.Broadcast(ctx, req.SignedAggregateAndProof); err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not broadcast signed aggregated attestation: %v", err)
|
|
}
|
|
|
|
log.WithFields(logrus.Fields{
|
|
"slot": req.SignedAggregateAndProof.Message.Aggregate.Data.Slot,
|
|
"committeeIndex": req.SignedAggregateAndProof.Message.Aggregate.Data.CommitteeIndex,
|
|
"validatorIndex": req.SignedAggregateAndProof.Message.AggregatorIndex,
|
|
"aggregatedCount": req.SignedAggregateAndProof.Message.Aggregate.AggregationBits.Count(),
|
|
}).Debug("Broadcasting aggregated attestation and proof")
|
|
|
|
return ðpb.SignedAggregateSubmitResponse{}, nil
|
|
}
|