mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
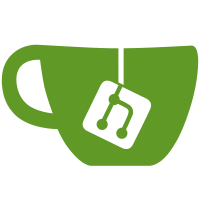
* Add a check for cold state storage * amend comment * gaz * Merge branch 'master' into coldstate-e2e * Merge refs/heads/master into coldstate-e2e * Merge refs/heads/master into coldstate-e2e * PR feedback * Merge branch 'coldstate-e2e' of github.com:prysmaticlabs/prysm into coldstate-e2e * Update endtoend/evaluators/data.go * Merge refs/heads/master into coldstate-e2e * Merge refs/heads/master into coldstate-e2e * Merge refs/heads/master into coldstate-e2e
44 lines
1.3 KiB
Go
44 lines
1.3 KiB
Go
package evaluators
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
|
|
eth "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/endtoend/types"
|
|
"google.golang.org/grpc"
|
|
)
|
|
|
|
const epochToCheck = 50 // must be more than 46 (32 hot states + 16 chkpt interval)
|
|
|
|
// ColdStateCheckpoint checks data from the database using cold state storage.
|
|
var ColdStateCheckpoint = types.Evaluator{
|
|
Name: "cold_state_assignments_from_epoch_%d",
|
|
Policy: func(currentEpoch uint64) bool {
|
|
return currentEpoch == epochToCheck
|
|
},
|
|
Evaluation: checkColdStateCheckpoint,
|
|
}
|
|
|
|
// Checks the first node for an old checkpoint using cold state storage.
|
|
func checkColdStateCheckpoint(conns ...*grpc.ClientConn) error {
|
|
ctx := context.Background()
|
|
client := eth.NewBeaconChainClient(conns[0])
|
|
|
|
for i := uint64(0); i < epochToCheck; i++ {
|
|
res, err := client.ListValidatorAssignments(ctx, ð.ListValidatorAssignmentsRequest{
|
|
QueryFilter: ð.ListValidatorAssignmentsRequest_Epoch{Epoch: i},
|
|
})
|
|
if err != nil {
|
|
return err
|
|
}
|
|
// A simple check to ensure we received some data.
|
|
if res == nil || res.Epoch != i {
|
|
return errors.New("failed to return a validator assignments response for an old epoch " +
|
|
"using cold state storage from the database")
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|