mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-22 19:40:37 +00:00
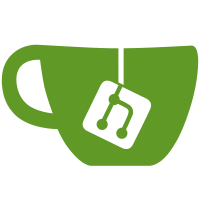
* `TestStore_GenesisValidatorsRoot_ReadAndWrite`: Make all test cases independents. In a test with multiple test cases, each test case should be independents. (aka: Removing test case `A` should not impact test case `B`) * `SaveGenesisValidatorsRoot`: Allow to overwrite the genesis validator root if the root is the same. * `ProposalHistoryForSlot`: Add `signingRootExists` Currently, it is not possible with `ProposalHistoryForSlot` to know if a proposal is stored with and `0x00000....` signing root or with an empty signing root. Both cases result to `proposalExists == true` and `signingRoot == 0x00000`. This commit adds a new return boolean: `signingRootExists`. If a proposal has been saved with a `0x00000...` signing root, then: - `proposalExists` is set to `true`, and - `signingRootExists` is set to `true`, and - `signingRoot` is set to `0x00000...` If a proposal has been saved with an empty signing root, then: - `proposalExists` is set to `true`, and - `signingRootExists` is set to `false`, and - (`signingRoot` is set to `0x00000...`) * `ImportStandardProtectionJSON`: When importing EIP-3076 Slashing Protection Interchange Format, do not filter any more slashable keys. Note: Those keys are still saved into the black-listed public keys list. There is two reason not to do so: - The EIP-3076 test cases do not know about Prysm's internal black-listed public keys list. Tests will expect, without looking into this internal black-listed public keys list, to deny a further signature. If we filter these keys from the DB (even if we keep them into the black-listed keys list), then some tests will fail. - If we import a interchange file containing slashable keys and we filter them, then, if we re-export the DB, those slashing offences won't appear in the exported interchange file. * `transformSignedBlocks`: Store an 0-len byte slice When importing an EIP-3076 interchange format, and when no signing root is specified into the file, we currently store a `0x00000.....` signing root. In such a case, instead storing `0x00000...`, this commit stores a 0-len byte array, so we can differentiate real `0x000.....` signing root and no signing-root at all. * `slashableProposalCheck`: Manage lack of sign root Currently, `slashableProposalCheck` does not really make a difference between a `0x0000.....` signing root and a missing signing root. (Signing roots can be missing when importing an EIP-3076 interchange file.) This commit differentiate, for `slashableProposalCheck`, `0x0000....` signing root and a missing signing root. * `AttestationRecord.SigningRoot`: ==> `[]byte` When importing attestations from EIP-3076 interchange format, the signing root of an attestation may be missing. Currently, Prysm consider any missing attestation signing root as `0x000...`. However, it may conflict with signing root which really are equal to `0x000...`. This commit transforms `AttestationRecord.SigningRoot` from `[32]byte` to `[]byte`, and change the minimal set of functions (sic) to support this new type. * `CheckSlashableAttestation`: Empty signing root Regarding slashing roots, 2 attestations are slashable, if: - both signing roots are defined and differs, or - one attestation exists, but without a signing root * `filterSlashablePubKeysFromAttestations`: Err sort Rergarding `CheckSlashableAttestation`, we consider that: - If slashable == NotSlashable and err != nil, then CheckSlashableAttestation failed. - If slashable != NotSlashable, then err contains the reason why the attestation is slashable. * `setupEIP3076SpecTests`: Update to `v5.3.0` This commit: - Updates the version of EIP-3076 tests to `v.5.2.1`. - Setups on anti-slashing DB per test case, instead per step. * `ImportStandardProtectionJSON`: Reduce cycl cmplxt * `AttestationHistoryForPubKey`: copy signing root BoltDB documentation specifies: | Byte slices returned from Bolt are only valid during a transaction. | Once the transaction has been committed or rolled back then the memory | they point to can be reused by a new page or can be unmapped | from virtual memory and you'll see an unexpected fault address panic | when accessing it.
87 lines
3.0 KiB
Go
87 lines
3.0 KiB
Go
package client
|
||
|
||
import (
|
||
"context"
|
||
"encoding/hex"
|
||
"fmt"
|
||
|
||
"github.com/pkg/errors"
|
||
fieldparams "github.com/prysmaticlabs/prysm/v4/config/fieldparams"
|
||
ethpb "github.com/prysmaticlabs/prysm/v4/proto/prysm/v1alpha1"
|
||
"github.com/prysmaticlabs/prysm/v4/proto/prysm/v1alpha1/slashings"
|
||
"github.com/prysmaticlabs/prysm/v4/validator/db/kv"
|
||
"go.opencensus.io/trace"
|
||
)
|
||
|
||
var failedAttLocalProtectionErr = "attempted to make slashable attestation, rejected by local slashing protection"
|
||
|
||
// Checks if an attestation is slashable by comparing it with the attesting
|
||
// history for the given public key in our DB. If it is not, we then update the history
|
||
// with new values and save it to the database.
|
||
func (v *validator) slashableAttestationCheck(
|
||
ctx context.Context,
|
||
indexedAtt *ethpb.IndexedAttestation,
|
||
pubKey [fieldparams.BLSPubkeyLength]byte,
|
||
signingRoot32 [32]byte,
|
||
) error {
|
||
ctx, span := trace.StartSpan(ctx, "validator.postAttSignUpdate")
|
||
defer span.End()
|
||
|
||
signingRoot := signingRoot32[:]
|
||
|
||
// Based on EIP3076, validator should refuse to sign any attestation with source epoch less
|
||
// than the minimum source epoch present in that signer’s attestations.
|
||
lowestSourceEpoch, exists, err := v.db.LowestSignedSourceEpoch(ctx, pubKey)
|
||
if err != nil {
|
||
return err
|
||
}
|
||
if exists && indexedAtt.Data.Source.Epoch < lowestSourceEpoch {
|
||
return fmt.Errorf(
|
||
"could not sign attestation lower than lowest source epoch in db, %d < %d",
|
||
indexedAtt.Data.Source.Epoch,
|
||
lowestSourceEpoch,
|
||
)
|
||
}
|
||
existingSigningRoot, err := v.db.SigningRootAtTargetEpoch(ctx, pubKey, indexedAtt.Data.Target.Epoch)
|
||
if err != nil {
|
||
return err
|
||
}
|
||
signingRootsDiffer := slashings.SigningRootsDiffer(existingSigningRoot, signingRoot)
|
||
|
||
// Based on EIP3076, validator should refuse to sign any attestation with target epoch less
|
||
// than or equal to the minimum target epoch present in that signer’s attestations.
|
||
lowestTargetEpoch, exists, err := v.db.LowestSignedTargetEpoch(ctx, pubKey)
|
||
if err != nil {
|
||
return err
|
||
}
|
||
if signingRootsDiffer && exists && indexedAtt.Data.Target.Epoch <= lowestTargetEpoch {
|
||
return fmt.Errorf(
|
||
"could not sign attestation lower than or equal to lowest target epoch in db, %d <= %d",
|
||
indexedAtt.Data.Target.Epoch,
|
||
lowestTargetEpoch,
|
||
)
|
||
}
|
||
fmtKey := "0x" + hex.EncodeToString(pubKey[:])
|
||
slashingKind, err := v.db.CheckSlashableAttestation(ctx, pubKey, signingRoot, indexedAtt)
|
||
if err != nil {
|
||
if v.emitAccountMetrics {
|
||
ValidatorAttestFailVec.WithLabelValues(fmtKey).Inc()
|
||
}
|
||
switch slashingKind {
|
||
case kv.DoubleVote:
|
||
log.Warn("Attestation is slashable as it is a double vote")
|
||
case kv.SurroundingVote:
|
||
log.Warn("Attestation is slashable as it is surrounding a previous attestation")
|
||
case kv.SurroundedVote:
|
||
log.Warn("Attestation is slashable as it is surrounded by a previous attestation")
|
||
}
|
||
return errors.Wrap(err, failedAttLocalProtectionErr)
|
||
}
|
||
|
||
if err := v.db.SaveAttestationForPubKey(ctx, pubKey, signingRoot32, indexedAtt); err != nil {
|
||
return errors.Wrap(err, "could not save attestation history for validator public key")
|
||
}
|
||
|
||
return nil
|
||
}
|