mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
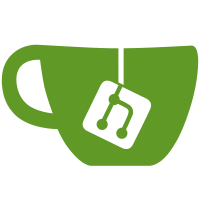
* slasher/beaconclient tests * slasher/db/kv tests * Merge branch 'master' into apply-testutils-assertions-to-slasher * fix build * slasher/detection tests * rest of the tests * misc tests * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Update slasher/db/kv/spanner_test.go Co-authored-by: Shay Zluf <thezluf@gmail.com> * Update slasher/node/node_test.go Co-authored-by: Shay Zluf <thezluf@gmail.com> * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Update slasher/db/kv/spanner_test.go * Merge refs/heads/master into apply-testutils-assertions-to-slasher
74 lines
2.2 KiB
Go
74 lines
2.2 KiB
Go
package beaconclient
|
|
|
|
import (
|
|
"context"
|
|
"testing"
|
|
|
|
"github.com/golang/mock/gomock"
|
|
ethpb "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/shared/mock"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/assert"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/require"
|
|
"github.com/prysmaticlabs/prysm/slasher/cache"
|
|
"github.com/sirupsen/logrus"
|
|
logTest "github.com/sirupsen/logrus/hooks/test"
|
|
)
|
|
|
|
func TestService_RequestValidator(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
logrus.SetLevel(logrus.TraceLevel)
|
|
ctrl := gomock.NewController(t)
|
|
defer ctrl.Finish()
|
|
client := mock.NewMockBeaconChainClient(ctrl)
|
|
validatorCache, err := cache.NewPublicKeyCache(0, nil)
|
|
require.NoError(t, err, "Could not create new cache")
|
|
bs := Service{
|
|
beaconClient: client,
|
|
publicKeyCache: validatorCache,
|
|
}
|
|
wanted := ðpb.Validators{
|
|
ValidatorList: []*ethpb.Validators_ValidatorContainer{
|
|
{
|
|
Index: 0, Validator: ðpb.Validator{PublicKey: []byte{1, 2, 3}},
|
|
},
|
|
{
|
|
Index: 1, Validator: ðpb.Validator{PublicKey: []byte{2, 4, 5}},
|
|
},
|
|
},
|
|
}
|
|
wanted2 := ðpb.Validators{
|
|
ValidatorList: []*ethpb.Validators_ValidatorContainer{
|
|
{
|
|
Index: 3, Validator: ðpb.Validator{PublicKey: []byte{3, 4, 5}},
|
|
},
|
|
},
|
|
}
|
|
client.EXPECT().ListValidators(
|
|
gomock.Any(),
|
|
gomock.Any(),
|
|
).Return(wanted, nil)
|
|
|
|
client.EXPECT().ListValidators(
|
|
gomock.Any(),
|
|
gomock.Any(),
|
|
).Return(wanted2, nil)
|
|
|
|
// We request public key of validator id 0,1.
|
|
res, err := bs.FindOrGetPublicKeys(context.Background(), []uint64{0, 1})
|
|
require.NoError(t, err)
|
|
for i, v := range wanted.ValidatorList {
|
|
assert.DeepEqual(t, wanted.ValidatorList[i].Validator.PublicKey, res[v.Index])
|
|
}
|
|
|
|
require.LogsContain(t, hook, "Retrieved validators id public key map:")
|
|
require.LogsDoNotContain(t, hook, "Retrieved validators public keys from cache:")
|
|
// We expect public key of validator id 0 to be in cache.
|
|
res, err = bs.FindOrGetPublicKeys(context.Background(), []uint64{0, 3})
|
|
require.NoError(t, err)
|
|
|
|
for i, v := range wanted2.ValidatorList {
|
|
assert.DeepEqual(t, wanted2.ValidatorList[i].Validator.PublicKey, res[v.Index])
|
|
}
|
|
require.LogsContain(t, hook, "Retrieved validators public keys from cache: map[0:[1 2 3]]")
|
|
}
|