mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
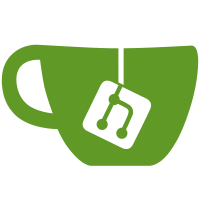
* Add database migrations, still need to update the API usage... * gofmt goimports * progress * Merge branch 'master' of github.com:prysmaticlabs/prysm into index-migration * use slot instead of index * rename LastArchivedIndex to LastArchivedSlot * rename LastArchivedIndexRoot to LastArchivedRoot * remove unused HighestSlotStates method * deprecate old key, include in migration * deprecate old key, include in migration * remove blocks index in migration * rename bucket variable * fix code to pass tests * Merge branch 'master' of github.com:prysmaticlabs/prysm into index-migration * gofmt, goimports * fix * Add state slot index * progress * lint * fix build * Merge branch 'master' of github.com:prysmaticlabs/prysm into index-migration * kafka * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * remove SaveArchivedPointRoot, a few other big changes * Merge branch 'index-migration' of github.com:prysmaticlabs/prysm into index-migration * fix tests and lint * lint again * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * block migration, some renaming * gaz, gofmt * add tests * change index to uint bytes * Merge branch 'index-migration' of github.com:prysmaticlabs/prysm into index-migration * rm method notes * stop if the bucket doesn't exist * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * @rauljordan pr feedback * Simplify * Merge refs/heads/master into index-migration * Remove unused method, add roundtrip test * gofmt * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge branch 'master' of github.com:prysmaticlabs/prysm into index-migration
103 lines
3.9 KiB
Go
103 lines
3.9 KiB
Go
package stategen
|
|
|
|
import (
|
|
"context"
|
|
"testing"
|
|
|
|
ethpb "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/cache"
|
|
testDB "github.com/prysmaticlabs/prysm/beacon-chain/db/testing"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/state/stateutil"
|
|
pb "github.com/prysmaticlabs/prysm/proto/beacon/p2p/v1"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/assert"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/require"
|
|
logTest "github.com/sirupsen/logrus/hooks/test"
|
|
)
|
|
|
|
func TestMigrateToCold_CanSaveFinalizedInfo(t *testing.T) {
|
|
ctx := context.Background()
|
|
db, c := testDB.SetupDB(t)
|
|
service := New(db, c)
|
|
beaconState, _ := testutil.DeterministicGenesisState(t, 32)
|
|
b := ðpb.SignedBeaconBlock{Block: ðpb.BeaconBlock{Slot: 1}}
|
|
br, err := stateutil.BlockRoot(b.Block)
|
|
require.NoError(t, err)
|
|
require.NoError(t, service.beaconDB.SaveBlock(ctx, b))
|
|
require.NoError(t, service.epochBoundaryStateCache.put(br, beaconState))
|
|
require.NoError(t, service.MigrateToCold(ctx, br))
|
|
|
|
wanted := &finalizedInfo{state: beaconState, root: br, slot: 1}
|
|
assert.DeepEqual(t, wanted, service.finalizedInfo, "Incorrect finalized info")
|
|
}
|
|
|
|
func TestMigrateToCold_HappyPath(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
ctx := context.Background()
|
|
db, _ := testDB.SetupDB(t)
|
|
|
|
service := New(db, cache.NewStateSummaryCache())
|
|
service.slotsPerArchivedPoint = 1
|
|
beaconState, _ := testutil.DeterministicGenesisState(t, 32)
|
|
stateSlot := uint64(1)
|
|
require.NoError(t, beaconState.SetSlot(stateSlot))
|
|
b := ðpb.SignedBeaconBlock{Block: ðpb.BeaconBlock{Slot: 2}}
|
|
fRoot, err := stateutil.BlockRoot(b.Block)
|
|
require.NoError(t, err)
|
|
require.NoError(t, service.beaconDB.SaveBlock(ctx, b))
|
|
require.NoError(t, service.epochBoundaryStateCache.put(fRoot, beaconState))
|
|
require.NoError(t, service.MigrateToCold(ctx, fRoot))
|
|
|
|
gotState, err := service.beaconDB.State(ctx, fRoot)
|
|
require.NoError(t, err)
|
|
assert.DeepEqual(t, beaconState.InnerStateUnsafe(), gotState.InnerStateUnsafe(), "Did not save state")
|
|
gotRoot := service.beaconDB.ArchivedPointRoot(ctx, stateSlot/service.slotsPerArchivedPoint)
|
|
assert.Equal(t, fRoot, gotRoot, "Did not save archived root")
|
|
lastIndex, err := service.beaconDB.LastArchivedSlot(ctx)
|
|
require.NoError(t, err)
|
|
assert.Equal(t, uint64(1), lastIndex, "Did not save last archived index")
|
|
|
|
testutil.AssertLogsContain(t, hook, "Saved state in DB")
|
|
}
|
|
|
|
func TestMigrateToCold_RegeneratePath(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
ctx := context.Background()
|
|
db, _ := testDB.SetupDB(t)
|
|
|
|
service := New(db, cache.NewStateSummaryCache())
|
|
service.slotsPerArchivedPoint = 1
|
|
beaconState, _ := testutil.DeterministicGenesisState(t, 32)
|
|
stateSlot := uint64(1)
|
|
require.NoError(t, beaconState.SetSlot(stateSlot))
|
|
blk := ðpb.SignedBeaconBlock{Block: ðpb.BeaconBlock{Slot: 2}}
|
|
fRoot, err := stateutil.BlockRoot(blk.Block)
|
|
require.NoError(t, err)
|
|
require.NoError(t, service.beaconDB.SaveBlock(ctx, blk))
|
|
require.NoError(t, service.beaconDB.SaveGenesisBlockRoot(ctx, fRoot))
|
|
if err := service.beaconDB.SaveStateSummary(ctx, &pb.StateSummary{
|
|
Slot: 1,
|
|
Root: fRoot[:],
|
|
}); err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
service.finalizedInfo = &finalizedInfo{
|
|
slot: 1,
|
|
root: fRoot,
|
|
state: beaconState,
|
|
}
|
|
|
|
require.NoError(t, service.MigrateToCold(ctx, fRoot))
|
|
|
|
gotState, err := service.beaconDB.State(ctx, fRoot)
|
|
require.NoError(t, err)
|
|
assert.DeepEqual(t, beaconState.InnerStateUnsafe(), gotState.InnerStateUnsafe(), "Did not save state")
|
|
gotRoot := service.beaconDB.ArchivedPointRoot(ctx, stateSlot/service.slotsPerArchivedPoint)
|
|
assert.Equal(t, fRoot, gotRoot, "Did not save archived root")
|
|
lastIndex, err := service.beaconDB.LastArchivedSlot(ctx)
|
|
require.NoError(t, err)
|
|
assert.Equal(t, uint64(1), lastIndex, "Did not save last archived index")
|
|
|
|
testutil.AssertLogsContain(t, hook, "Saved state in DB")
|
|
}
|