mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
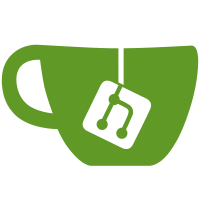
* Clean testutil, change tool names to Deterministic * Cleanup errors * Merge branch 'master' of https://github.com/prysmaticlabs/prysm into clean-testutil * Fix bug with generating deposits * Fix a few tests * Fix most tests * Clean up some tests * Remove err pt. 1 * Remove err pt. 2 * Change tests to use genesis state util * Remove err from deposits * Merge branch 'master' of https://github.com/prysmaticlabs/Prysm into clean-testutil * Remove circular dependency * Remove uncompressed signature test * Merge branch 'master' of https://github.com/prysmaticlabs/Prysm into clean-testutil * Merge branch 'master' into clean-testutil * Goimports * gazelle * Merge branch 'master' of https://github.com/prysmaticlabs/Prysm into clean-testutil * Add back error handling * New attestation pool (#4185) * New pool * Better namings * Fmt * Gazelle * Merge branch 'master' of https://github.com/prysmaticlabs/prysm into define-pool * Raul's feedback * Raul's feedback * Log peer conected log for incoming connections (#4173) * Log peer conected log for incoming connections * Merge branch 'master' into peerconnected * Merge branch 'master' into peerconnected * Update handshake.go * Update handshake.go * Merge branch 'master' into peerconnected * Merge branch 'master' into peerconnected * Attestation pool to use go-cache (#4187) * Update EthereumAPIs (#4186) * include new patch targeting latest ethapis master * ensure project builds * Merge branch 'master' into update-all-api * fix up committees * Merge branch 'update-all-api' of github.com:prysmaticlabs/prysm into update-all-api * include latest eth apis * Merge branch 'master' into update-all-api * update block tests * Merge branch 'update-all-api' of github.com:prysmaticlabs/prysm into update-all-api * Merge branch 'master' into update-all-api * add todos * Implement GetValidator RPC Endpoint (#4188) * include new patch targeting latest ethapis master * ensure project builds * Merge branch 'master' into update-all-api * fix up committees * Merge branch 'update-all-api' of github.com:prysmaticlabs/prysm into update-all-api * include latest eth apis * Merge branch 'master' into update-all-api * update block tests * Merge branch 'update-all-api' of github.com:prysmaticlabs/prysm into update-all-api * Merge branch 'master' into update-all-api * add todos * implement get validator rpc * add test for get validator * table driven test * fix up test * fix confs * tests for more cases * fix up tests and add out of range * Slasher optimization (#4172) * size * batching and concurrency improvements * gaz * merge fixes * fix comment * fix test * fix test * fix build * ethpb * ethpb * fix test * fix comment * add benchmark * fix benchmark * Handle error for all testutil uses * Fix errors * Merge branch 'master' of https://github.com/prysmaticlabs/Prysm into clean-testutil * Revert error handling Revert "Fix errors" This reverts commit db081f5486f62c854e7a34e792f5e380cfa922e7. Revert "Handle error for all testutil uses" This reverts commit bdabef230632dd184491c2dad63c7d3e74a3861b. Revert "Add back error handling" This reverts commit da7e3d2020cd906f45f452d4e441b566f2d5c8aa. * Change genesis state func to use testing.T * Merge branch 'master' of https://github.com/prysmaticlabs/Prysm into clean-testutil * Merge branch 'master' of https://github.com/prysmaticlabs/Prysm into clean-testutil * Fix conflict * Merge branch 'master' of https://github.com/prysmaticlabs/Prysm into clean-testutil * Merge branch 'master' into clean-testutil * Merge branch 'master' into clean-testutil * Captialize other logs * Merge branch 'clean-testutil' of https://github.com/0xKiwi/Prysm into clean-testutil * Merge branch 'master' of https://github.com/prysmaticlabs/Prysm into clean-testutil * Merge branch 'master' into clean-testutil
165 lines
4.4 KiB
Go
165 lines
4.4 KiB
Go
package endtoend
|
|
|
|
import (
|
|
"bufio"
|
|
"fmt"
|
|
"io"
|
|
"io/ioutil"
|
|
"os"
|
|
"os/exec"
|
|
"path"
|
|
"strings"
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/bazelbuild/rules_go/go/tools/bazel"
|
|
"github.com/ethereum/go-ethereum/common"
|
|
"github.com/pkg/errors"
|
|
ev "github.com/prysmaticlabs/prysm/endtoend/evaluators"
|
|
)
|
|
|
|
type beaconNodeInfo struct {
|
|
processID int
|
|
datadir string
|
|
rpcPort uint64
|
|
monitorPort uint64
|
|
grpcPort uint64
|
|
multiAddr string
|
|
}
|
|
|
|
type end2EndConfig struct {
|
|
minimalConfig bool
|
|
tmpPath string
|
|
epochsToRun uint64
|
|
numValidators uint64
|
|
numBeaconNodes uint64
|
|
contractAddr common.Address
|
|
evaluators []ev.Evaluator
|
|
}
|
|
|
|
// startBeaconNodes starts the requested amount of beacon nodes, passing in the deposit contract given.
|
|
func startBeaconNodes(t *testing.T, config *end2EndConfig) []*beaconNodeInfo {
|
|
numNodes := config.numBeaconNodes
|
|
|
|
nodeInfo := []*beaconNodeInfo{}
|
|
for i := uint64(0); i < numNodes; i++ {
|
|
newNode := startNewBeaconNode(t, config, nodeInfo)
|
|
nodeInfo = append(nodeInfo, newNode)
|
|
}
|
|
|
|
return nodeInfo
|
|
}
|
|
|
|
func startNewBeaconNode(t *testing.T, config *end2EndConfig, beaconNodes []*beaconNodeInfo) *beaconNodeInfo {
|
|
tmpPath := config.tmpPath
|
|
index := len(beaconNodes)
|
|
binaryPath, found := bazel.FindBinary("beacon-chain", "beacon-chain")
|
|
if !found {
|
|
t.Log(binaryPath)
|
|
t.Fatal("beacon chain binary not found")
|
|
}
|
|
file, err := os.Create(path.Join(tmpPath, fmt.Sprintf("beacon-%d.log", index)))
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
args := []string{
|
|
"--no-discovery",
|
|
"--http-web3provider=http://127.0.0.1:8545",
|
|
"--web3provider=ws://127.0.0.1:8546",
|
|
fmt.Sprintf("--datadir=%s/eth2-beacon-node-%d", tmpPath, index),
|
|
fmt.Sprintf("--deposit-contract=%s", config.contractAddr.Hex()),
|
|
fmt.Sprintf("--rpc-port=%d", 4000+index),
|
|
fmt.Sprintf("--p2p-udp-port=%d", 12000+index),
|
|
fmt.Sprintf("--p2p-tcp-port=%d", 13000+index),
|
|
fmt.Sprintf("--monitoring-port=%d", 8080+index),
|
|
fmt.Sprintf("--grpc-gateway-port=%d", 3200+index),
|
|
}
|
|
|
|
if config.minimalConfig {
|
|
args = append(args, "--minimal-config")
|
|
}
|
|
// After the first node is made, have all following nodes connect to all previously made nodes.
|
|
if index >= 1 {
|
|
for p := 0; p < index; p++ {
|
|
args = append(args, fmt.Sprintf("--peer=%s", beaconNodes[p].multiAddr))
|
|
}
|
|
}
|
|
|
|
t.Logf("Starting beacon chain with flags %s", strings.Join(args, " "))
|
|
cmd := exec.Command(binaryPath, args...)
|
|
cmd.Stderr = file
|
|
cmd.Stdout = file
|
|
if err := cmd.Start(); err != nil {
|
|
t.Fatalf("Failed to start beacon node: %v", err)
|
|
}
|
|
|
|
if err = waitForTextInFile(file, "Node started p2p server"); err != nil {
|
|
t.Fatalf("could not find multiaddr for node %d, this means the node had issues starting: %v", index, err)
|
|
}
|
|
|
|
multiAddr, err := getMultiAddrFromLogFile(file.Name())
|
|
if err != nil {
|
|
t.Fatalf("could not get multiaddr fpr node %d: %v", index, err)
|
|
}
|
|
|
|
return &beaconNodeInfo{
|
|
processID: cmd.Process.Pid,
|
|
datadir: fmt.Sprintf("%s/eth2-beacon-node-%d", tmpPath, index),
|
|
rpcPort: 4000 + uint64(index),
|
|
monitorPort: 8080 + uint64(index),
|
|
grpcPort: 3200 + uint64(index),
|
|
multiAddr: multiAddr,
|
|
}
|
|
}
|
|
|
|
func getMultiAddrFromLogFile(name string) (string, error) {
|
|
byteContent, err := ioutil.ReadFile(name)
|
|
if err != nil {
|
|
return "", err
|
|
}
|
|
contents := string(byteContent)
|
|
|
|
searchText := "\"Node started p2p server\" multiAddr=\""
|
|
startIdx := strings.Index(contents, searchText)
|
|
if startIdx == -1 {
|
|
return "", fmt.Errorf("did not find peer text in %s", contents)
|
|
}
|
|
startIdx += len(searchText)
|
|
endIdx := strings.Index(contents[startIdx:], "\"")
|
|
if endIdx == -1 {
|
|
return "", fmt.Errorf("did not find peer text in %s", contents)
|
|
}
|
|
return contents[startIdx : startIdx+endIdx], nil
|
|
}
|
|
|
|
func waitForTextInFile(file *os.File, text string) error {
|
|
wait := 0
|
|
// Putting the wait cap at 24 seconds.
|
|
totalWait := 24
|
|
for wait < totalWait {
|
|
time.Sleep(2 * time.Second)
|
|
// Rewind the file pointer to the start of the file so we can read it again.
|
|
_, err := file.Seek(0, io.SeekStart)
|
|
if err != nil {
|
|
return errors.Wrap(err, "could not rewind file to start")
|
|
}
|
|
|
|
scanner := bufio.NewScanner(file)
|
|
for scanner.Scan() {
|
|
if strings.Contains(scanner.Text(), text) {
|
|
return nil
|
|
}
|
|
}
|
|
if err := scanner.Err(); err != nil {
|
|
return err
|
|
}
|
|
wait += 2
|
|
}
|
|
contents, err := ioutil.ReadFile(file.Name())
|
|
if err != nil {
|
|
return err
|
|
}
|
|
return fmt.Errorf("could not find requested text %s in logs:\n%s", text, string(contents))
|
|
}
|