mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
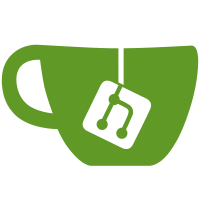
* update shared/params * update eth2-types deps * update protobufs * update shared/* * fix testutil/state * update beacon-chain/state * update beacon-chain/db * update tests * fix test * update beacon-chain/core * update beacon-chain/blockchain * update beacon-chain/cache * beacon-chain/forkchoice * update beacon-chain/operations * update beacon-chain/p2p * update beacon-chain/rpc * update sync/initial-sync * update deps * update deps * go fmt * update beacon-chain/sync * update endtoend/ * bazel build //beacon-chain - works w/o issues * update slasher code * udpate tools/ * update validator/ * update fastssz * fix build * fix test building * update tests * update ethereumapis deps * fix tests * update state/stategen * fix build * fix test * add FarFutureSlot * go imports * Radek's suggestions * Ivan's suggestions * type conversions * Nishant's suggestions * add more tests to rpc_send_request * fix test * clean up * fix conflicts Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com> Co-authored-by: nisdas <nishdas93@gmail.com>
66 lines
1.7 KiB
Go
66 lines
1.7 KiB
Go
package cache
|
|
|
|
import (
|
|
"testing"
|
|
|
|
types "github.com/prysmaticlabs/eth2-types"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/assert"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/require"
|
|
)
|
|
|
|
func TestSubnetIDsCache_RoundTrip(t *testing.T) {
|
|
c := newSubnetIDs()
|
|
slot := types.Slot(100)
|
|
committeeIDs := c.GetAggregatorSubnetIDs(slot)
|
|
assert.Equal(t, 0, len(committeeIDs), "Empty cache returned an object")
|
|
|
|
c.AddAggregatorSubnetID(slot, 1)
|
|
res := c.GetAggregatorSubnetIDs(slot)
|
|
assert.DeepEqual(t, []uint64{1}, res)
|
|
|
|
c.AddAggregatorSubnetID(slot, 2)
|
|
res = c.GetAggregatorSubnetIDs(slot)
|
|
assert.DeepEqual(t, []uint64{1, 2}, res)
|
|
|
|
c.AddAggregatorSubnetID(slot, 3)
|
|
res = c.GetAggregatorSubnetIDs(slot)
|
|
assert.DeepEqual(t, []uint64{1, 2, 3}, res)
|
|
|
|
committeeIDs = c.GetAttesterSubnetIDs(slot)
|
|
assert.Equal(t, 0, len(committeeIDs), "Empty cache returned an object")
|
|
|
|
c.AddAttesterSubnetID(slot, 11)
|
|
res = c.GetAttesterSubnetIDs(slot)
|
|
assert.DeepEqual(t, []uint64{11}, res)
|
|
|
|
c.AddAttesterSubnetID(slot, 22)
|
|
res = c.GetAttesterSubnetIDs(slot)
|
|
assert.DeepEqual(t, []uint64{11, 22}, res)
|
|
|
|
c.AddAttesterSubnetID(slot, 33)
|
|
res = c.GetAttesterSubnetIDs(slot)
|
|
assert.DeepEqual(t, []uint64{11, 22, 33}, res)
|
|
}
|
|
|
|
func TestSubnetIDsCache_PersistentCommitteeRoundtrip(t *testing.T) {
|
|
c := newSubnetIDs()
|
|
|
|
for i := 0; i < 20; i++ {
|
|
pubkey := [48]byte{byte(i)}
|
|
c.AddPersistentCommittee(pubkey[:], []uint64{uint64(i)}, 0)
|
|
}
|
|
|
|
for i := uint64(0); i < 20; i++ {
|
|
pubkey := [48]byte{byte(i)}
|
|
|
|
idxs, ok, _ := c.GetPersistentSubnets(pubkey[:])
|
|
if !ok {
|
|
t.Errorf("Couldn't find entry in cache for pubkey %#x", pubkey)
|
|
continue
|
|
}
|
|
require.Equal(t, i, idxs[0])
|
|
}
|
|
coms := c.GetAllSubnets()
|
|
assert.Equal(t, 20, len(coms))
|
|
}
|