mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
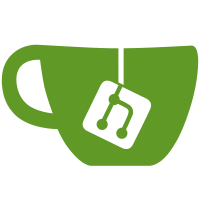
* e2e docs * slasher docs * Merge branch 'other-package-godocs' of github.com:prysmaticlabs/prysm into other-package-godocs * all validator package comments * Merge branch 'master' into other-package-godocs * completed all other packages * Merge branch 'master' into other-package-godocs * Merge refs/heads/master into other-package-godocs
70 lines
1.7 KiB
Go
70 lines
1.7 KiB
Go
// Package testutil defines common unit test utils such as asserting logs.
|
|
package testutil
|
|
|
|
import (
|
|
"strings"
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/sirupsen/logrus/hooks/test"
|
|
)
|
|
|
|
// AssertLogsContain checks that the desired string is a subset of the current log output.
|
|
// Set exitOnFail to true to immediately exit the test on failure
|
|
func AssertLogsContain(t *testing.T, hook *test.Hook, want string) {
|
|
assertLogs(t, hook, want, true)
|
|
}
|
|
|
|
// AssertLogsDoNotContain is the inverse check of AssertLogsContain
|
|
func AssertLogsDoNotContain(t *testing.T, hook *test.Hook, want string) {
|
|
assertLogs(t, hook, want, false)
|
|
}
|
|
|
|
func assertLogs(t *testing.T, hook *test.Hook, want string, flag bool) {
|
|
t.Logf("scanning for: %s", want)
|
|
entries := hook.AllEntries()
|
|
match := false
|
|
for _, e := range entries {
|
|
msg, err := e.String()
|
|
if err != nil {
|
|
t.Fatalf("Failed to format log entry to string: %v", err)
|
|
}
|
|
if strings.Contains(msg, want) {
|
|
match = true
|
|
}
|
|
t.Logf("log: %s", msg)
|
|
}
|
|
|
|
if flag && !match {
|
|
t.Fatalf("log not found: %s", want)
|
|
} else if !flag && match {
|
|
t.Fatalf("unwanted log found: %s", want)
|
|
}
|
|
}
|
|
|
|
// WaitForLog waits for the desired string to appear the logs within a
|
|
// time period. If it does not appear within the limit, the function
|
|
// will throw an error.
|
|
func WaitForLog(t *testing.T, hook *test.Hook, want string) {
|
|
t.Logf("waiting for: %s", want)
|
|
match := false
|
|
timer := time.After(1 * time.Second)
|
|
|
|
for {
|
|
select {
|
|
case <-timer:
|
|
t.Fatalf("log not found in time period: %s", want)
|
|
default:
|
|
if match {
|
|
return
|
|
}
|
|
entries := hook.AllEntries()
|
|
for _, e := range entries {
|
|
if strings.Contains(e.Message, want) {
|
|
match = true
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|