mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
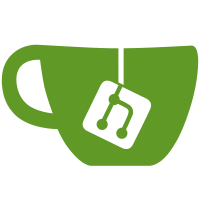
* implemented all the merkle func signatures * branch indices complete * check for index out of range in generating merkle proof * completed full tests for sparse merkle trie impl * lint * formatting * gazelle * commentary * ivan comments * fmt * get rid of the deposit trie * recalculate trie when eth1 data changes * default data response recalculates tree * update merkle trie to use raw bytes * use the new verify merkle root func * builds * if default data response historical deposits are empty, return the deposit root at the contract at the time * work on trie * trying again with more logging * keep track of merkle trie indices, use correct big int ops * use uint for merkle idx * add todo * update ticker correctly * fix config and remove unnecessary logs * readd plus one fix * clarify some details * weird imports spacing * gazelle, lint * fix tests using the new deposit trie * builds but tests still fail * rpc tests * lint * tests pass * bazel lint * rem commented block * revert att slot fix * preston comments * comments * fix build * address last comment * use counter * imports
61 lines
1.8 KiB
Go
61 lines
1.8 KiB
Go
package db
|
|
|
|
import (
|
|
"context"
|
|
"math/big"
|
|
"sort"
|
|
|
|
"github.com/prometheus/client_golang/prometheus"
|
|
"github.com/prometheus/client_golang/prometheus/promauto"
|
|
pb "github.com/prysmaticlabs/prysm/proto/beacon/p2p/v1"
|
|
"github.com/sirupsen/logrus"
|
|
"go.opencensus.io/trace"
|
|
)
|
|
|
|
var (
|
|
historicalDepositsCount = promauto.NewCounter(prometheus.CounterOpts{
|
|
Name: "beacondb_all_deposits",
|
|
Help: "The number of total deposits in the beaconDB in-memory database",
|
|
})
|
|
)
|
|
|
|
// InsertDeposit into the database. If deposit or block number are nil
|
|
// then this method does nothing.
|
|
func (db *BeaconDB) InsertDeposit(ctx context.Context, d *pb.Deposit, blockNum *big.Int) {
|
|
ctx, span := trace.StartSpan(ctx, "BeaconDB.InsertDeposit")
|
|
defer span.End()
|
|
if d == nil || blockNum == nil {
|
|
log.WithFields(logrus.Fields{
|
|
"block": blockNum,
|
|
"deposit": d,
|
|
}).Debug("Ignoring nil deposit insertion")
|
|
return
|
|
}
|
|
db.depositsLock.Lock()
|
|
defer db.depositsLock.Unlock()
|
|
db.deposits = append(db.deposits, &depositContainer{deposit: d, block: blockNum})
|
|
historicalDepositsCount.Inc()
|
|
}
|
|
|
|
// AllDeposits returns a list of deposits all historical deposits until the given block number
|
|
// (inclusive). If no block is specified then this method returns all historical deposits.
|
|
func (db *BeaconDB) AllDeposits(ctx context.Context, beforeBlk *big.Int) []*pb.Deposit {
|
|
ctx, span := trace.StartSpan(ctx, "BeaconDB.AllDeposits")
|
|
defer span.End()
|
|
db.depositsLock.RLock()
|
|
defer db.depositsLock.RUnlock()
|
|
|
|
var deposits []*pb.Deposit
|
|
for _, ctnr := range db.deposits {
|
|
if beforeBlk == nil || beforeBlk.Cmp(ctnr.block) > -1 {
|
|
deposits = append(deposits, ctnr.deposit)
|
|
}
|
|
}
|
|
// Sort the deposits by Merkle index.
|
|
sort.SliceStable(deposits, func(i, j int) bool {
|
|
return deposits[i].MerkleTreeIndex < deposits[j].MerkleTreeIndex
|
|
})
|
|
|
|
return deposits
|
|
}
|