mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
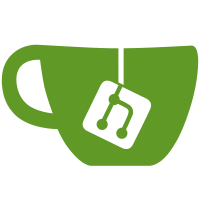
* move testutil * util pkg * build * gaz Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com>
76 lines
2.3 KiB
Go
76 lines
2.3 KiB
Go
package client
|
|
|
|
import (
|
|
"testing"
|
|
|
|
types "github.com/prysmaticlabs/eth2-types"
|
|
ethpb "github.com/prysmaticlabs/prysm/proto/prysm/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/testing/require"
|
|
logTest "github.com/sirupsen/logrus/hooks/test"
|
|
)
|
|
|
|
func TestLogNextDutyCountDown_NoDuty(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
v := &validator{
|
|
logDutyCountDown: true,
|
|
duties: ðpb.DutiesResponse{CurrentEpochDuties: []*ethpb.DutiesResponse_Duty{
|
|
{AttesterSlot: 100, ProposerSlots: []types.Slot{105}},
|
|
{AttesterSlot: 110},
|
|
{AttesterSlot: 120},
|
|
}},
|
|
}
|
|
require.NoError(t, v.LogNextDutyTimeLeft(121))
|
|
require.LogsContain(t, hook, "No duty until next epoch")
|
|
}
|
|
|
|
func TestLogNextDutyCountDown_HasDutyAttester(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
v := &validator{
|
|
logDutyCountDown: true,
|
|
duties: ðpb.DutiesResponse{CurrentEpochDuties: []*ethpb.DutiesResponse_Duty{
|
|
{AttesterSlot: 100, ProposerSlots: []types.Slot{105}},
|
|
{AttesterSlot: 110},
|
|
{AttesterSlot: 120},
|
|
}},
|
|
}
|
|
require.NoError(t, v.LogNextDutyTimeLeft(115))
|
|
require.LogsContain(t, hook, "\"Next duty\" attesting=1 currentSlot=115 dutySlot=120 prefix=validator proposing=0")
|
|
}
|
|
|
|
func TestLogNextDutyCountDown_HasDutyProposer(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
v := &validator{
|
|
logDutyCountDown: true,
|
|
duties: ðpb.DutiesResponse{CurrentEpochDuties: []*ethpb.DutiesResponse_Duty{
|
|
{AttesterSlot: 100, ProposerSlots: []types.Slot{105}},
|
|
{AttesterSlot: 110},
|
|
{AttesterSlot: 120},
|
|
}},
|
|
}
|
|
require.NoError(t, v.LogNextDutyTimeLeft(101))
|
|
require.LogsContain(t, hook, "\"Next duty\" attesting=0 currentSlot=101 dutySlot=105 prefix=validator proposing=1")
|
|
}
|
|
|
|
func TestLogNextDutyCountDown_HasMultipleDuties(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
v := &validator{
|
|
logDutyCountDown: true,
|
|
duties: ðpb.DutiesResponse{CurrentEpochDuties: []*ethpb.DutiesResponse_Duty{
|
|
{AttesterSlot: 120},
|
|
{AttesterSlot: 110},
|
|
{AttesterSlot: 105},
|
|
{AttesterSlot: 105},
|
|
{AttesterSlot: 100, ProposerSlots: []types.Slot{105}},
|
|
}},
|
|
}
|
|
require.NoError(t, v.LogNextDutyTimeLeft(101))
|
|
require.LogsContain(t, hook, "\"Next duty\" attesting=2 currentSlot=101 dutySlot=105 prefix=validator proposing=1")
|
|
}
|
|
|
|
func TestLogNextDutyCountDown_NilDuty(t *testing.T) {
|
|
v := &validator{
|
|
logDutyCountDown: true,
|
|
}
|
|
require.NoError(t, v.LogNextDutyTimeLeft(101))
|
|
}
|