mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-29 06:37:17 +00:00
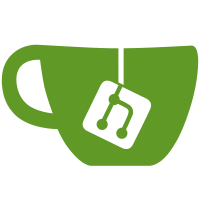
* add reject all pubsub validator to stop automatic propagation of messages * gaz * Merge branch 'master' of github.com:prysmaticlabs/prysm into pubsub-validator * refactor p2p validator pipeline * add sanity check * Merge branch 'pubsub-validator' of github.com:prysmaticlabs/prysm into pubsub-validator * fixed up test * rem * gaz * Merge refs/heads/master into pubsub-validator * fix from self test * ensure validator data is set * resolve todo * Merge refs/heads/master into pubsub-validator * gaz * Merge refs/heads/master into pubsub-validator * Merge branch 'pubsub-validator' of github.com:prysmaticlabs/prysm into pubsub-validator * Merge refs/heads/master into pubsub-validator * remove all of the 'from self' logic. filed https://github.com/libp2p/go-libp2p-pubsub/issues/250 * Merge branch 'pubsub-validator' of github.com:prysmaticlabs/prysm into pubsub-validator * gaz * update comment * Merge refs/heads/master into pubsub-validator * rename "VaidatorData" * Merge branch 'pubsub-validator' of github.com:prysmaticlabs/prysm into pubsub-validator * refactor * one more bit of refactoring * Update beacon-chain/sync/validate_beacon_attestation.go Co-Authored-By: terence tsao <terence@prysmaticlabs.com> * skip validation on self messages, add @nisdas feedback to increment failure counter * Merge branch 'pubsub-validator' of github.com:prysmaticlabs/prysm into pubsub-validator * remove flakey
82 lines
2.7 KiB
Go
82 lines
2.7 KiB
Go
package sync
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
|
|
"github.com/libp2p/go-libp2p-core/peer"
|
|
pubsub "github.com/libp2p/go-libp2p-pubsub"
|
|
"github.com/pkg/errors"
|
|
ethpb "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/shared/bytesutil"
|
|
"github.com/prysmaticlabs/prysm/shared/traceutil"
|
|
"github.com/sirupsen/logrus"
|
|
"go.opencensus.io/trace"
|
|
)
|
|
|
|
var errPointsToBlockNotInDatabase = errors.New("attestation points to a block which is not in the database")
|
|
|
|
// validateBeaconAttestation validates that the block being voted for passes validation before forwarding to the
|
|
// network.
|
|
func (r *Service) validateBeaconAttestation(ctx context.Context, pid peer.ID, msg *pubsub.Message) bool {
|
|
// Validation runs on publish (not just subscriptions), so we should approve any message from
|
|
// ourselves.
|
|
if pid == r.p2p.PeerID() {
|
|
return true
|
|
}
|
|
|
|
// Attestation processing requires the target block to be present in the database, so we'll skip
|
|
// validating or processing attestations until fully synced.
|
|
if r.initialSync.Syncing() {
|
|
return false
|
|
}
|
|
|
|
ctx, span := trace.StartSpan(ctx, "sync.validateBeaconAttestation")
|
|
defer span.End()
|
|
|
|
// TODO(1332): Add blocks.VerifyAttestation before processing further.
|
|
// Discussion: https://github.com/ethereum/eth2.0-specs/issues/1332
|
|
|
|
m, err := r.decodePubsubMessage(msg)
|
|
if err != nil {
|
|
log.WithError(err).Error("Failed to decode message")
|
|
traceutil.AnnotateError(span, err)
|
|
return false
|
|
}
|
|
att, ok := m.(*ethpb.Attestation)
|
|
if !ok {
|
|
traceutil.AnnotateError(span, errors.New("wrong proto message type"))
|
|
log.Error("Wrong proto message type")
|
|
return false
|
|
}
|
|
|
|
span.AddAttributes(
|
|
trace.StringAttribute("blockRoot", fmt.Sprintf("%#x", att.Data.BeaconBlockRoot)),
|
|
)
|
|
|
|
// Only valid blocks are saved in the database.
|
|
if !r.db.HasBlock(ctx, bytesutil.ToBytes32(att.Data.BeaconBlockRoot)) {
|
|
log.WithField(
|
|
"blockRoot",
|
|
fmt.Sprintf("%#x", att.Data.BeaconBlockRoot),
|
|
).WithError(errPointsToBlockNotInDatabase).Debug("Ignored incoming attestation that points to a block which is not in the database")
|
|
traceutil.AnnotateError(span, errPointsToBlockNotInDatabase)
|
|
return false
|
|
}
|
|
|
|
finalizedEpoch := r.chain.FinalizedCheckpt().Epoch
|
|
attestationDataEpochOld := finalizedEpoch >= att.Data.Source.Epoch || finalizedEpoch >= att.Data.Target.Epoch
|
|
if finalizedEpoch != 0 && attestationDataEpochOld {
|
|
traceutil.AnnotateError(span, errors.New("wrong proto message type"))
|
|
log.WithFields(logrus.Fields{
|
|
"TargetEpoch": att.Data.Target.Epoch,
|
|
"SourceEpoch": att.Data.Source.Epoch,
|
|
}).Debug("Rejecting old attestation")
|
|
return false
|
|
}
|
|
|
|
msg.ValidatorData = att // Used in downstream subscriber
|
|
|
|
return true
|
|
}
|