mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-22 19:40:37 +00:00
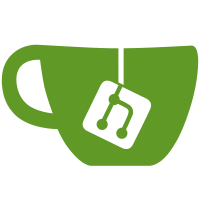
* Update V3 from V4 * Fix build v3 -> v4 * Update ssz * Update beacon_chain.pb.go * Fix formatter import * Update update-mockgen.sh comment to v4 * Fix conflicts. Pass build and tests * Fix test
57 lines
1.4 KiB
Go
57 lines
1.4 KiB
Go
//go:build !fuzz
|
|
|
|
package cache
|
|
|
|
import (
|
|
"context"
|
|
"testing"
|
|
|
|
fuzz "github.com/google/gofuzz"
|
|
"github.com/prysmaticlabs/prysm/v4/testing/assert"
|
|
"github.com/prysmaticlabs/prysm/v4/testing/require"
|
|
)
|
|
|
|
func TestCommitteeKeyFuzz_OK(t *testing.T) {
|
|
fuzzer := fuzz.NewWithSeed(0)
|
|
c := &Committees{}
|
|
|
|
for i := 0; i < 100000; i++ {
|
|
fuzzer.Fuzz(c)
|
|
k, err := committeeKeyFn(c)
|
|
require.NoError(t, err)
|
|
assert.Equal(t, key(c.Seed), k)
|
|
}
|
|
}
|
|
|
|
func TestCommitteeCache_FuzzCommitteesByEpoch(t *testing.T) {
|
|
cache := NewCommitteesCache()
|
|
fuzzer := fuzz.NewWithSeed(0)
|
|
c := &Committees{}
|
|
|
|
for i := 0; i < 100000; i++ {
|
|
fuzzer.Fuzz(c)
|
|
require.NoError(t, cache.AddCommitteeShuffledList(context.Background(), c))
|
|
_, err := cache.Committee(context.Background(), 0, c.Seed, 0)
|
|
require.NoError(t, err)
|
|
}
|
|
|
|
assert.Equal(t, maxCommitteesCacheSize, len(cache.CommitteeCache.Keys()), "Incorrect key size")
|
|
}
|
|
|
|
func TestCommitteeCache_FuzzActiveIndices(t *testing.T) {
|
|
cache := NewCommitteesCache()
|
|
fuzzer := fuzz.NewWithSeed(0)
|
|
c := &Committees{}
|
|
|
|
for i := 0; i < 100000; i++ {
|
|
fuzzer.Fuzz(c)
|
|
require.NoError(t, cache.AddCommitteeShuffledList(context.Background(), c))
|
|
|
|
indices, err := cache.ActiveIndices(context.Background(), c.Seed)
|
|
require.NoError(t, err)
|
|
assert.DeepEqual(t, c.SortedIndices, indices)
|
|
}
|
|
|
|
assert.Equal(t, maxCommitteesCacheSize, len(cache.CommitteeCache.Keys()), "Incorrect key size")
|
|
}
|